As a React software developer, managing the structure of components efficiently is crucial for building scalable and maintainable applications. One powerful tool in a React developer’s arsenal is React Fragments.
Despite being a relatively simple concept, React Fragments can significantly impact the way components are organized and rendered. In this article, we’ll see what React Fragments are, why they are important, and how they can be used to improve your React applications.
Understanding React Fragments
In React, components are the building blocks of UIs, encapsulating various pieces of user interface logic and presentation. Traditionally, when rendering components, they must return a single parent element. For instance, consider a scenario where you want to render a list of items using a component. Without React Fragments, you might be tempted to wrap these items in an unnecessary parent <div>
element just to satisfy this requirement:
// Without Fragments
const ItemList = () => {
return (
<div>
<Item />
<Item />
<Item />
</div>
);
};
While this approach works perfectly fine, it introduces an extra DOM element (<div>
) that serves no semantic purpose other than satisfying React’s rendering constraints. This can clutter the DOM unnecessarily and affect the structure of your HTML.
Enter React Fragments
React Fragments provide a solution to this problem by allowing you to group multiple elements without adding extra nodes to the DOM. They act as a wrapper, similar to a <div>
, but without actually creating a new DOM node. Instead, they enable you to return a list of elements without any additional wrapping:
// With Fragments
import React, { Fragment } from 'react';
const ItemList = () => {
return (
<Fragment>
<Item />
<Item />
<Item />
</Fragment>
);
};
In the above example, <Fragment>
is a special syntax provided by React to create a fragment. You can also use the shorthand syntax <>
and </>
instead of <Fragment>
and </Fragment>
respectively.
// With Fragments
import React from 'react';
const ItemList = () => {
return (
<>
<Item />
<Item />
<Item />
</>
);
};
Benefits of Using React Fragments
1. Cleaner DOM Structure:
React Fragments help keep your DOM structure cleaner by eliminating unnecessary wrapper elements. This leads to more semantic and concise markup.
2. Improved Performance:
Since React Fragments don’t add extra DOM nodes, they contribute to better rendering performance and reduced memory overhead, especially when dealing with large lists or nested components.
3. Enhanced Readability:
By removing the clutter of wrapper elements, React Fragments make your JSX code more readable and maintainable, as it focuses solely on the content of your components.
4. Compatibility:
React Fragments are fully compatible with key React features like the virtual DOM, JSX syntax, and server-side rendering, ensuring seamless integration into your React applications.
Practical Use Cases
1. Lists and Iterations:
Use React Fragments to render lists of items without adding unnecessary <div>
elements around each item.
2. Grouping UI Elements:
Group related UI elements together using React Fragments, especially when rendering multiple components within a single parent component.
3. Conditional Rendering:
Conditionally render multiple elements without introducing additional wrapper elements based on certain conditions.
Conclusion
React Fragments offer a simple yet powerful way to structure your components more efficiently, improving both performance and readability. By eliminating the need for unnecessary wrapper elements, React Fragments allow you to create cleaner, more concise JSX code without compromising on functionality. Whether you’re rendering lists, grouping UI elements, or conditionally rendering components, React Fragments provide a versatile solution for organizing your React applications.
In your next React project, consider leveraging React Fragments to streamline your component structure and optimize rendering performance. With their minimal syntax and maximum impact, React Fragments empower you to build better React applications with cleaner, more efficient code.
Become a certified React Developer
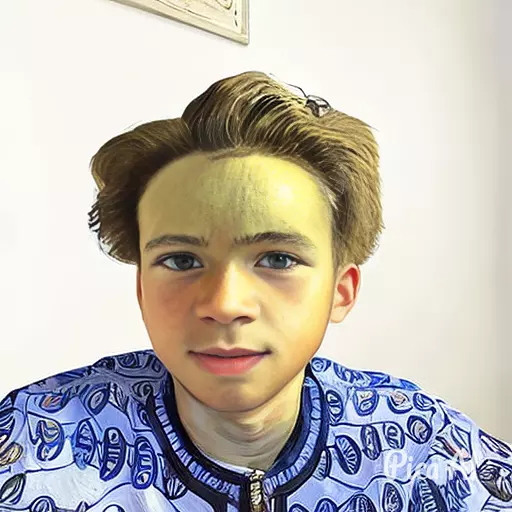