Estimated reading time: 3 minutes
According to the React Native Navigation documentation, the StackNavigator provides a way for your app to transition between screens where each new screen is placed on top of a stack.
Unlike the web browser, react native doesn’t have a built-in API to handle screen navigation. If you want to make use of navigation in your project, you will have to specify what type of navigation you want to use and add the respective dependencies.
The StackNavigator in React Native provides a way for your app to transition between screens and manage navigation history.
Here are the steps you can take to quickly create a React Native Stack Navigator, fast and easy.
Step 1: Add the required dependencies
Navigation in React is a little different from what is generally obtainable, especially in HTML where you just have to wrap the url or the file path in anchor tags. In React, however, you have to make sure that you have added the needed dependencies. These include the navigation dependency, gesture handler, masked view as well as the stack navigator itself.
To put it simply, for every component that you want to use in React Native, you will need to make sure there is a supported dependency for it.
On your terminal or command prompt, cd to your project folder and add the following:
yarn add @react-native-community/masked-view
yarn add react-native-gesture-handler
yarn add react-native-reanimated
yarn add react-native-safe-area-context
yarn add react-navigation
yarn add react-navigation-stack
Step 2: Create your screens
Next we will create a Home, About and Contact screens. But first let us create a folder called screens.
Home screen: Create a Home.js screen and add the following code
import React, {Component} from 'react';
import {View, Text, StyleSheet} from 'react-native';
class Home extends Component {
render() {
return (
<View
style={{
flex: 1,
flexDirection: 'column',
alignItems: 'center',
justifyContent: 'center',
}}>
<Text style={styles.textStyle}>Home</Text>
<Text
style={styles.textStyle}
onPress={() => this.props.navigation.navigate('About')}>
About
</Text>
<Text
style={styles.textStyle}
onPress={() => this.props.navigation.navigate('Contact')}>
Contact
</Text>
</View>
);
}
}
const styles = StyleSheet.create({
textStyle: {
fontSize: 22,
},
});
export default Home;
Create an About.js screen and add the following code:
import React, { Component } from "react";
import { View, Text } from 'react-native';
class About extends Component{
render(){
return(
<View style={{flex:1, flexDirection:'column', alignItems:'center', justifyContent:'center'}}>
<Text style={{fontSize: 22}}>
About us
</Text>
</View>
)
}
}
export default About;
Create a Contact.js and add the below code
import React, { Component } from "react";
import { View, Text } from 'react-native';
class Contact extends Component {
render(){
return(
<View>
<Text>
Contact
</Text>
</View>
)
}
}
export default Contact;
Step 3: Create the Stack Navigator
Finally we will create a folder called routes and add the Stack.js file
Stack.js
import React, { Component } from "react";
import { createAppContainer } from "react-navigation";
import { createStackNavigator } from "react-navigation-stack";
import About from "../screens/About";
import Contact from "../screens/Contact";
import Home from "../screens/Home";
class Stack extends Component{
render(){
return(
<AppContainer />
)
}
}
const myStack = createStackNavigator({
'Home' : Home,
'About' : About,
'Contact' : Contact
}, {
initialRouteName: 'Home'
});
const AppContainer = createAppContainer(myStack)
export default Stack;
Result:
Congratulations! You have successfully created a React Native Stack Navigator.
Watch Video Tutorial instead?
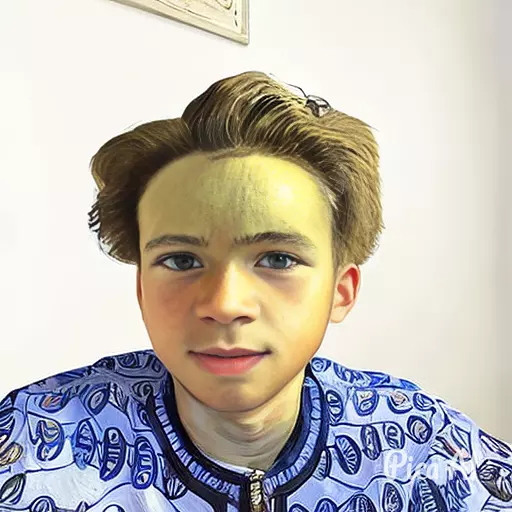