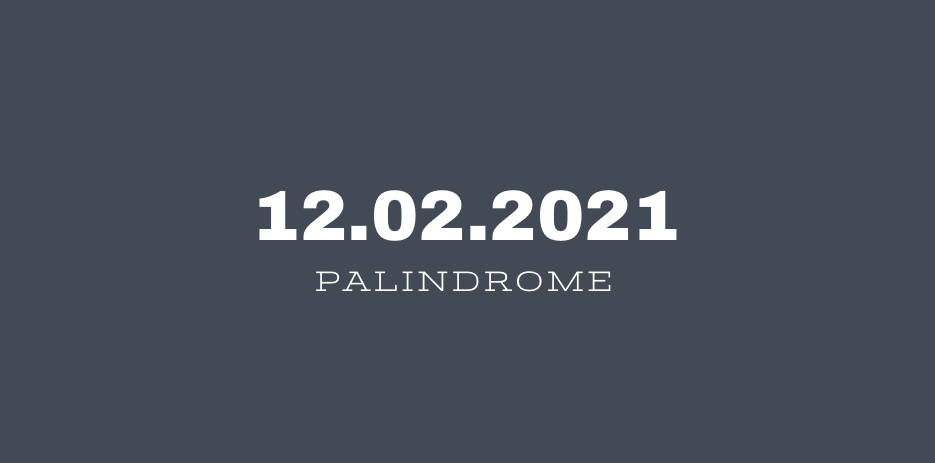
It is true that Strings can be palindromic in nature. The same can equally be said of numbers.
A palindromic number (sometimes called numeral or numeric palindrome) is one such that if its digits are reversed, it stays the same.
This number is said to have a reflectional symmetry across the vertical axis. Example of such numbers are 16461, 151, 242, etc.
Let us now see how we can check for this palindromic quality using Java programming language.
class CheckPalindrome{
public static void main(Strings args[]){
System.out.println(isPalindromeInt(121));
}
public static String isPalindromeInt(int n) {
int reversedNum = 0;
int remainder;
int originalNum = n;
while (n != 0) {
remainder = num % 10;
reversedNum = reversedNum * 10 + remainder;
n /= 10;
}
// check if reversedNum and originalNum are equal
if (originalNum == reversedNum) {
return originalNum + "is Palindrome.";
}
else {
return originalNum + "is not Palindrome.";
}
}
}
So what we did here was to create a static method that will return our given result based on the parameters passed.
By using the modulus operator, we effectively get the remainder when we divide by 10 and then attempt to do a reversal.
If the reversed number is still equal to the reversed number, then it is a palindrome, otherwise it is not.
This is effectively how Palindrome works.
Let us know what you think in the comments.