A function is a group of reusable code that can be called anywhere in your program. Functions eliminate the need of writing the same code again and again.
Functions also allow a programmer to divide a big program into a small and manageable chunks.
The way to define a function in Javascript is to use the “function” keyword.
function functionName() {
//statements here ...
}
You could also use ES6 Arrow Functions as well
Passing Parameters
Parameters can be passed while calling a function. These type of functions are called Functions with parameters.
Example
function greet(name, job){
document.write(`Name is ${name} and occupation is ${job}`);
}
<body>
<input type="button" onclick="greet('Lawson', 'Software developer')" value="greet" />
</body>
The Return Statement
A Javascript function can have an optional return statement. This is required if you want to return a value from a function. This statement should be the last statement in a function.
Example
function add(a, b){
return a + b;
}
console.log(add(2,3)) //5
Nested Functions
In a nested function, a function can be called inside another function. And it goes on and on depending on the logic of the program.
Example
function showResult(){
console.log(add(2,3)) //Our function from the previous example
}
showResult() //5
Read more about recursive functions here
The End.
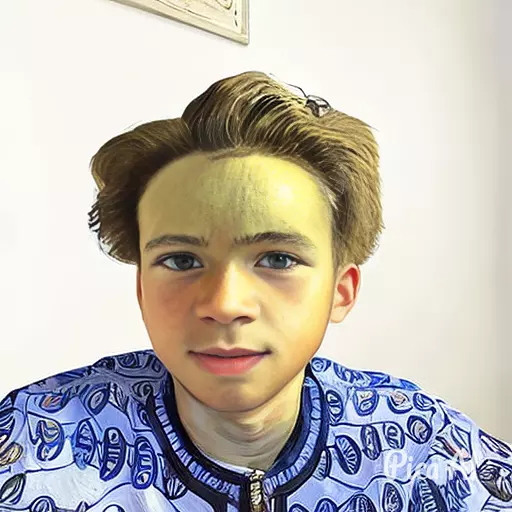