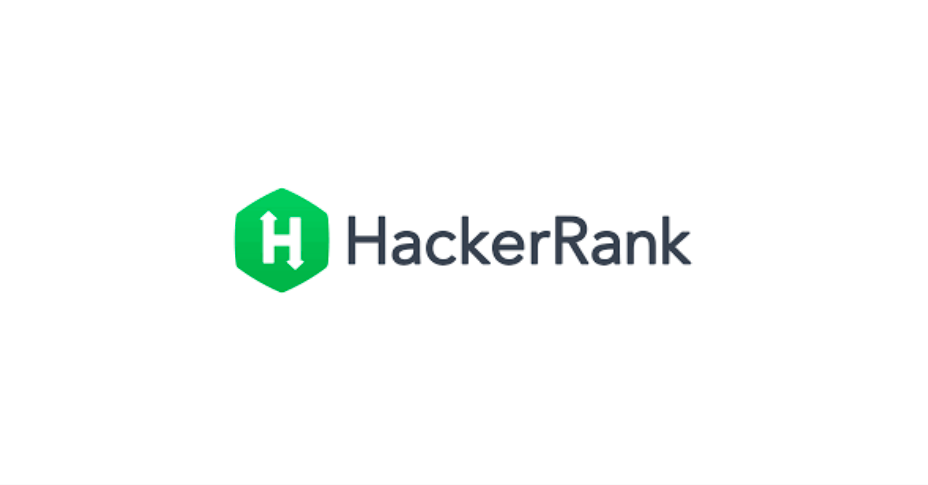
solve the hackerrank substring challenge (Java solution)
In this article we will solve the HackerRank Substring challenge (Java solution). Let’s take a look at the question
Question:
A substring is a group of contiguous characters in a string. For instance, all substrings of abc are [a, b, c, ab, bc, abc].
In this challenge, you will be given a binary representation of a number. You must determine the total number of substrings present that match the following conditions:
- The 0’s and 1’s are grouped consecutively (e.g., 01, 10, 0011, 1100, 000111, etc).
- The number of 0’s in the substring is equal to the number of 1’s in the substring.
As an example, consider the string 001101. The 4 substrings matching the two conditions include [0011, 01, 10, 01]. Note that 01 appears twice, from indexes 1-2 and 4-5. There are other substrings, e.g. 001 and 011 that match the first condition but not the second.
Java Solution to the Hackerrank challenge:
Let’s solve the hackerrank substring challenge using Java
import java.io.*;
import java.util.*;
import java.text.*;
import java.math.*;
import java.util.regex.*;
public class Solution {
/*
* Complete the function below.
*/
static int counting(String s) {
int curr = 1, prev = 0, ans = 0;
for(int i = 1; i< s.length(); i++)
if(s.charAt(i) == s.charAt(i-1)) curr++;
else{
ans += Math.min(curr, prev);
prev = curr;
curr = 1;
}
return ans + Math.min(curr, prev);
}
public static void main(String[] args) throws IOException {
Scanner in = new Scanner(System.in);
final String fileName = System.getenv("OUTPUT_PATH");
BufferedWriter bw = null;
if (fileName != null) {
bw = new BufferedWriter(new FileWriter(fileName));
}
else {
bw = new BufferedWriter(new OutputStreamWriter(System.out));
}
int res;
String s;
try {
s = in.nextLine();
} catch (Exception e) {
s = null;
}
res = counting(s);
bw.write(String.valueOf(res));
bw.newLine();
bw.close();
}
}
Here, we declared three variables cur, prev and ans. We’re also looping through to get the characters at the given index.
This is how we solve the hackerrank substring challenge using Java
Download the Codeflare mobile app and start learning how to code
Solve the FizzBuzz HackerRank Challenge
Start your software development training journey
public static int CountUniqueSubstrings(string s)
{
int count = 0;
int n = s.Length;
HashSet uniqueChars = new HashSet();
for (int i = 0; i < n; i++)
{
uniqueChars.Clear();
for (int j = i; j < n; j++)
{
if (uniqueChars.Contains(s[j]))
{
break;
}
uniqueChars.Add(s[j]);
count++;
}
}
return count;
}