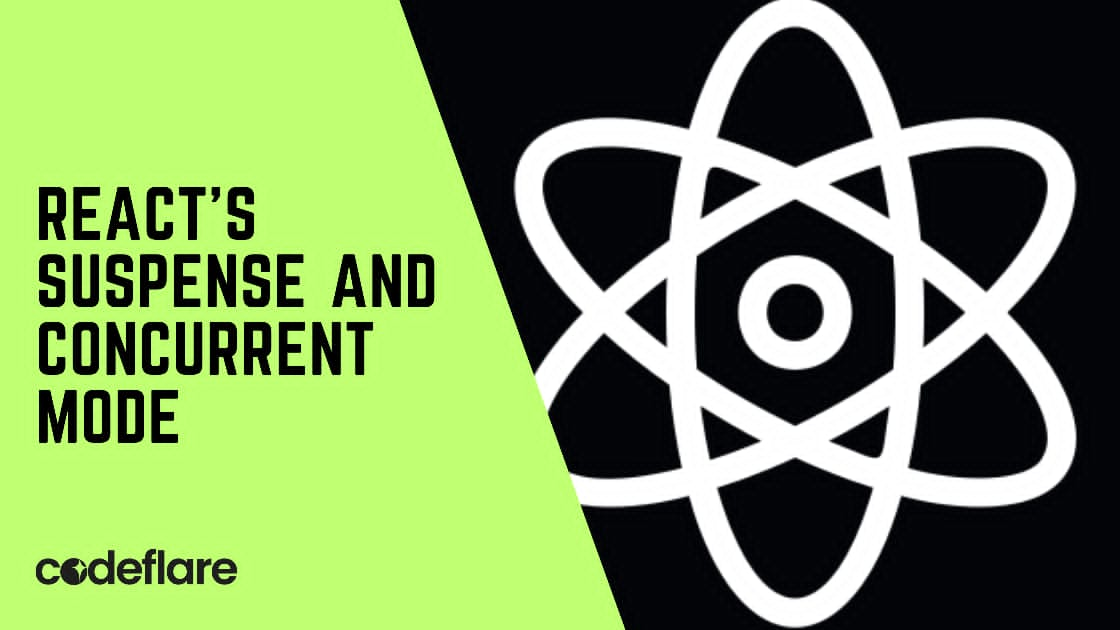
React’s Suspense and Concurrent Mode represent two of the most exciting advancements in React, designed to make user interfaces more responsive, seamless, and efficient. These features introduce new ways to handle asynchronous rendering and manage UI updates more gracefully, improving the overall user experience.
In this article, we’ll dive into what Suspense and Concurrent Mode are, how they work, and why they are game-changers in the React ecosystem.
What is Suspense?
Suspense in React is a powerful feature that allows you to wait for some code (typically asynchronous data) to load before rendering a part of the component tree. This is incredibly useful when you have components that depend on asynchronous data, like API calls.
It allows you to declaratively handle loading states in your app. Suspense helps improve the user experience by providing a smoother transition when loading data or code splitting.
Basic Example of Suspense:
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function App() {
return (
<div>
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
</div>
);
}
export default App;
In this example:
- We use
React.lazy()
to dynamically importLazyComponent
. - The
<Suspense>
component wraps aroundLazyComponent
and provides afallback
UI while waiting for it to load. In this case, the fallback is a simple “Loading…” message.
This improves the app’s performance by loading components only when they are needed, rather than at the initial page load.
Suspense for Data Fetching
Initially introduced for code splitting, Suspense is also designed to handle asynchronous data fetching. Although it’s still in the experimental phase, it provides a more declarative way to manage data loading by pausing rendering until the necessary data is available.
Here’s an example using the experimental Suspense for Data Fetching:
const resource = fetchData();
function MyComponent() {
const data = resource.read();
return <div>{data}</div>;
}
function App() {
return (
<Suspense fallback={<div>Loading data...</div>}>
<MyComponent />
</Suspense>
);
}
In this example:
fetchData()
returns a resource thatSuspense
can wait on.- The rendering is suspended until the
resource.read()
method completes.
What is Concurrent Mode?
Concurrent Mode is another experimental feature in React designed to make applications more responsive by allowing React to interrupt long-running tasks. Instead of processing large updates all at once, React can prioritize important updates, such as user interactions, making the app feel faster and more responsive.
Concurrent Mode introduces a new way of rendering that makes React applications handle heavy computations more efficiently by focusing on user-perceived performance.
Key Features of Concurrent Mode
- Time-Slicing:
- React can break down large rendering tasks into smaller chunks and spread them over multiple frames, giving the browser more time to handle user interactions.
- Interruptible Rendering:
- React can pause rendering and prioritize more urgent tasks (like user input), then resume rendering later. This ensures smoother user interactions.
- Automatic Batching:
- React batches multiple state updates and renders them in one go, optimizing the performance of your app by reducing the number of renders.
How Suspense and Concurrent Mode Work Together
Suspense and Concurrent Mode are designed to complement each other. When you use Suspense in Concurrent Mode, React can start rendering parts of your UI that are ready while waiting for other parts (e.g., data fetching) to complete.
Here’s an example of how these two can work together:
import React, { Suspense } from 'react';
import { createRoot } from 'react-dom/client';
import fetchData from './fetchData';
const resource = fetchData();
function MyComponent() {
const data = resource.read();
return <div>{data}</div>;
}
const rootElement = document.getElementById('root');
const root = createRoot(rootElement);
root.render(
<Suspense fallback={<div>Loading...</div>}>
<MyComponent />
</Suspense>
);
In this example, Concurrent Mode ensures that rendering is responsive, while Suspense pauses the rendering of MyComponent
until its data is ready.
Benefits of Using Suspense and Concurrent Mode
- Improved User Experience:
- With Suspense, you can show placeholder content while the app loads critical resources, resulting in smoother transitions and better user experience.
- Prioritizing Important Updates:
- Concurrent Mode ensures that more important tasks, such as handling user interactions, are prioritized over background tasks, making your app feel snappier.
- Better Performance:
- By breaking large renders into smaller tasks, React can ensure the browser doesn’t get locked up, preventing the “freezing” effect users often experience with complex UIs.
- Declarative Loading States:
- Instead of manually managing loading states, Suspense allows you to declare what should happen while waiting for resources.
Conclusion
React’s Suspense and Concurrent Mode are powerful tools that enhance the performance and user experience of modern web applications. By using Suspense for loading states and Concurrent Mode for rendering optimization, developers can create smoother, more responsive applications.
Understanding PHP Autoload functionality