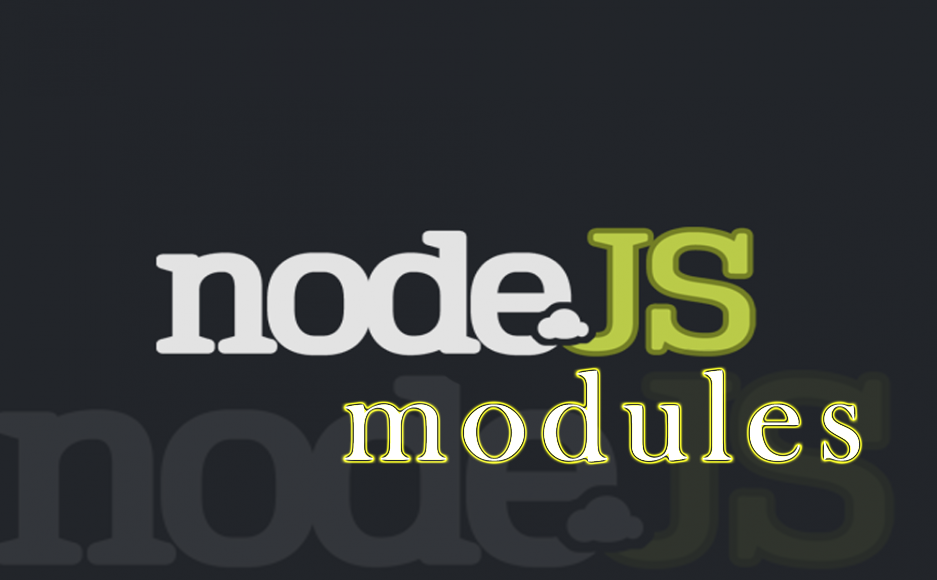
Modules are generally set of functions that you can include in your application.
These could be built-in functions that are part of the Node.js library or imported from a third-party library.
Built-in Modules
Node.js has a set of built-in modules which you can use without any further installation.
These include:
Module | Description |
---|---|
assert | Provides a set of assertion tests |
buffer | To handle binary data |
child_process | To run a child process |
cluster | To split a single Node process into multiple processes |
crypto | To handle OpenSSL cryptographic functions |
dgram | Provides implementation of UDP datagram sockets |
dns | To do DNS lookups and name resolution functions |
domain | Deprecated. To handle unhandled errors |
events | To handle events |
fs | To handle the file system |
http | To make Node.js act as an HTTP server |
https | To make Node.js act as an HTTPS server. |
net | To create servers and clients |
os | Provides information about the operation system |
path | To handle file paths |
punycode | Deprecated. A character encoding scheme |
querystring | To handle URL query strings |
readline | To handle readable streams one line at the time |
stream | To handle streaming data |
string_decoder | To decode buffer objects into strings |
timers | To execute a function after a given number of milliseconds |
tls | To implement TLS and SSL protocols |
tty | Provides classes used by a text terminal |
url | To parse URL strings |
util | To access utility functions |
v8 | To access information about V8 (the JavaScript engine) |
vm | To compile JavaScript code in a virtual machine |
zlib | To compress or decompress files |
Using a Module
To use or require a module, we use the require() function followed by the name of the module
Example:
let http = require('http');
Creating Your Own Module
You can create your own modules and include them in your project.
First, let us create a module called Time.js as follows
exports.mytTime = function() {
return Date();
}
Next, let us create a main.js file where we can use this newly-created module
let http = require("http");
let date = require('./Time');
http.createServer(function (request, response) {
response.writeHead(200, {'Content-Type': 'text/plain'});
// Send the response body as "Hello World"
response.end(`Hello world. Thank you The time is ${date.mytTime()}`);
}).listen(80);
Next we run the file on port 80 and we get the response printed on the screen.
This is how we can create modules that we can use in our project