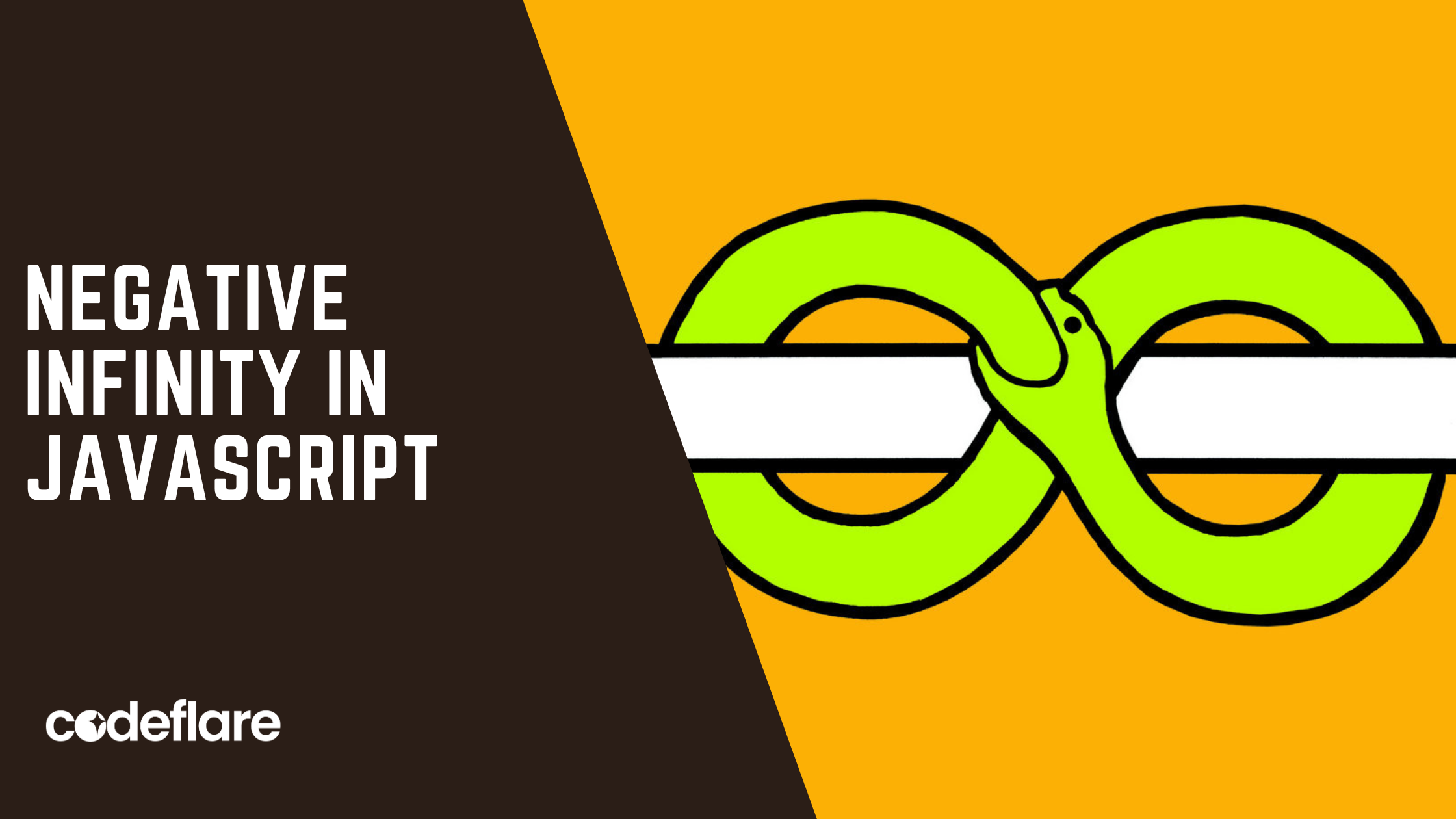
Negative infinity in JavaScript is a special value that represents the concept of a number that is infinitely small or approaching negative infinity. In this article, we’ll delve into what negative infinity is, how it is represented in JavaScript, its use cases, and some considerations when working with it in the context of programming.
Apply For Software Development Training Here
Introduction to Infinity in JavaScript:
In JavaScript, infinity is a numeric value that represents positive infinity, while negative infinity represents a number that is infinitely small. These values are used to denote mathematical concepts where a number exceeds the limits of representable values. The keyword Infinity
is used to represent positive infinity, and its negative counterpart is represented as -Infinity
.
Representing Negative Infinity in JavaScript:
To denote negative infinity in JavaScript, you use the constant -Infinity
. It’s important to note that -Infinity
is case-sensitive, so it must be written exactly as shown, with a capital “I”.
let negativeInfinity = -Infinity;
This variable negativeInfinity
now holds the value of negative infinity.
Use Cases of Negative Infinity:
- Mathematical Calculations:
- Negative infinity is often used in mathematical calculations where a value approaches negative infinity as a limit.
- For example, in calculus, when discussing limits as a variable approaches negative infinity,
-Infinity
is used to represent that concept.
- Sorting Arrays:
- When sorting arrays, negative infinity can be used as a placeholder for elements that should come first in the sorted order.
- This is useful when implementing custom sorting algorithms.
let numbers = [3, 1, 4, -Infinity, 2];
numbers.sort(); // Result: [-Infinity, 1, 2, 3, 4]
- Default Values:
- Negative infinity can be used as a default value when working with minimum values, ensuring that any real value provided will be greater than the default.
let minValue = Math.min(someValue, -Infinity);
Considerations when Working with Negative Infinity:
- Comparison:
- Comparisons involving negative infinity can behave unexpectedly. For example, any finite number is greater than negative infinity, but comparing negative infinity with itself results in equality.
console.log(-Infinity < 0); // true
console.log(-Infinity === -Infinity); // true
- Arithmetic Operations:
- Performing arithmetic operations with negative infinity can also lead to unexpected results. For example, adding or subtracting any finite number from negative infinity still results in negative infinity.
console.log(-Infinity + 5); // -Infinity
console.log(-Infinity - 10); // -Infinity
Conclusion:
In JavaScript, negative infinity is a special value that represents the concept of a number that is infinitely small or approaches negative infinity as a limit. It is a crucial part of the language when dealing with mathematical calculations, sorting algorithms, and default values. Understanding how to use and handle negative infinity is essential for writing robust and predictable JavaScript code. As with any programming concept, it’s crucial to be aware of potential pitfalls and handle negative infinity appropriately in different scenarios.
Take The JavaScript Quiz Today
Test your JavaScript proficiency level by taking the JavaScript quiz and downloading your certificate right away. Take the quiz
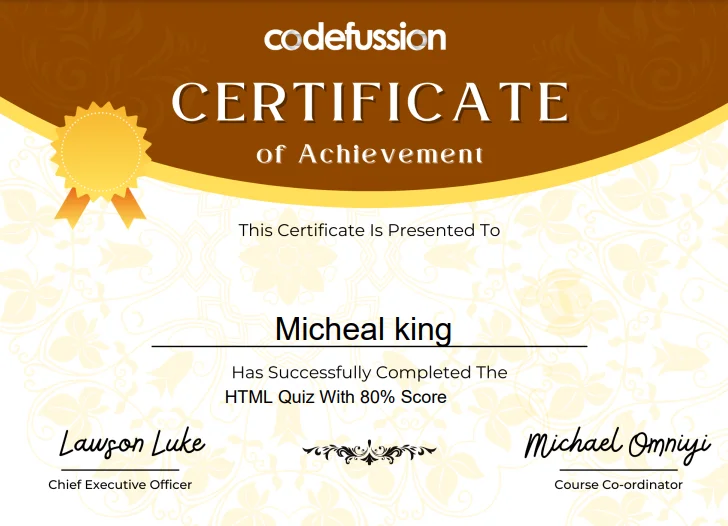