Login forms help give authentication to users of a particular platform, and it is utilised most applications.
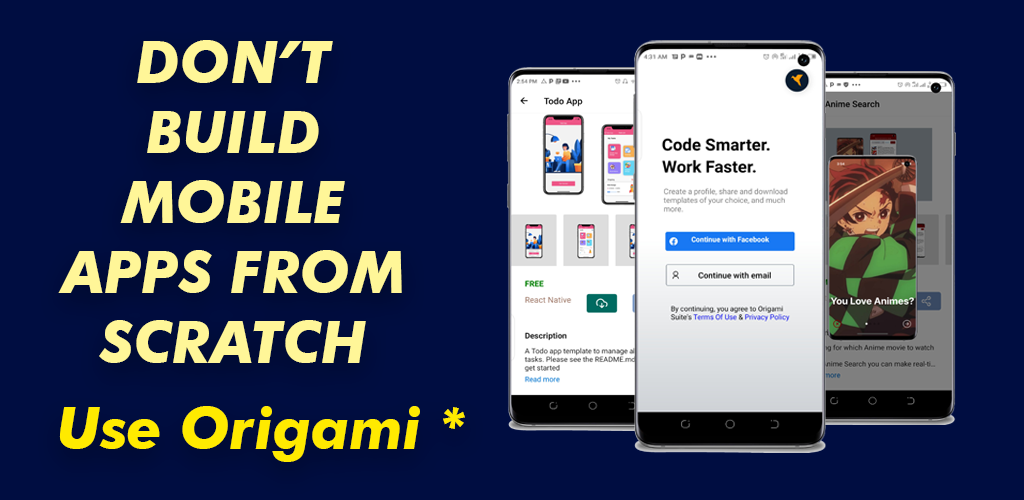
The toggle password visibility button both reveal and hide the passwords of user as they enter them in the password.
This helps users see and verify their password combination before clicking the the submit button.
Let’s see how to implement Login and Registration form in Android Studio.
1. Create a new Android Studio Project
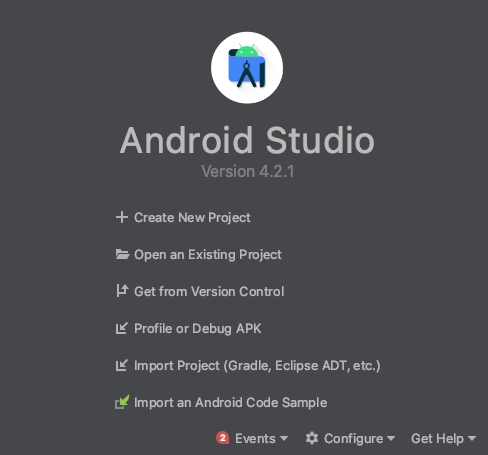
2. Choose Empty Activity
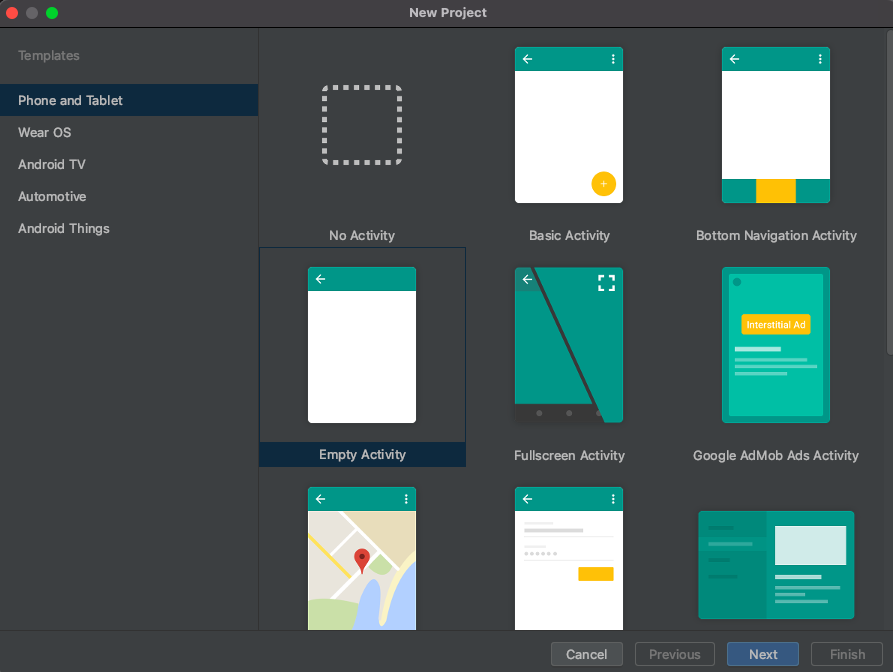
3. Name Your Project and Click Finish
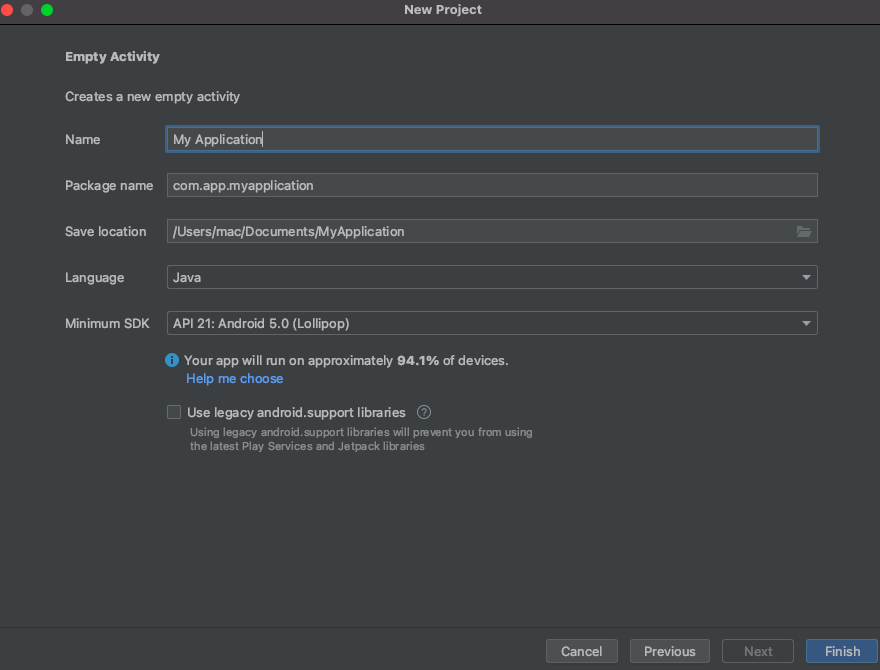
4. Add Show and Hide icons to Drawable Folder
Go to File -> New -> Vector Asset
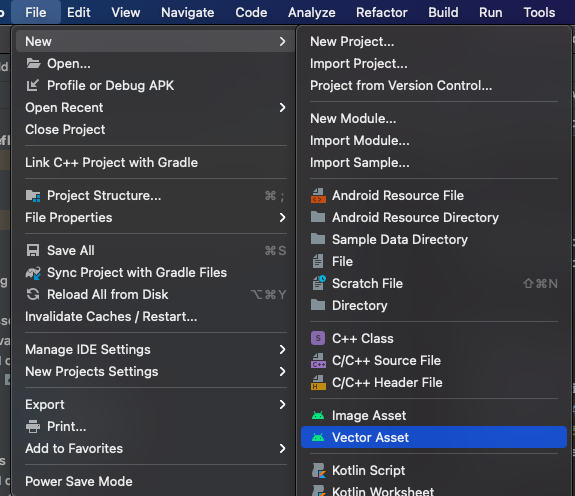
5. Click on the clip art icon and search icon
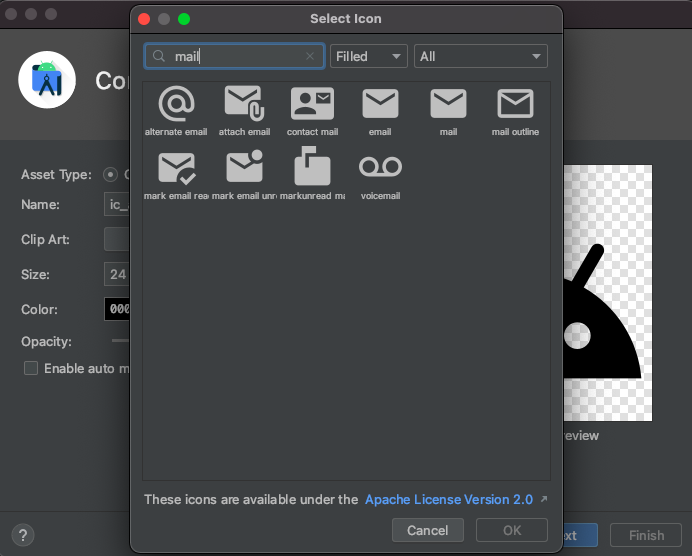
6. Add the following code to your activity_main.xml or similar layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<LinearLayout
android:id="@+id/layout_first"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical"
android:layout_marginTop="27dp">
<ImageView
android:layout_width="90dp"
android:padding="18dp"
android:layout_height="90dp"
android:background="@drawable/round_layout"
android:src="@drawable/key" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:layout_marginTop="18dp"
android:text="@string/wlc_sign_in"/>
</LinearLayout>
<LinearLayout
android:id="@+id/form"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingStart="27dp"
android:paddingEnd="27dp"
android:gravity="center"
android:layout_marginTop="36dp"
android:orientation="vertical"
android:layout_below="@+id/layout_first">
<com.google.android.material.textfield.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/email"
app:startIconDrawable="@drawable/ic_baseline_email_24"
android:background="@color/white"
android:layout_marginBottom="27dp">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:background="@color/white"
android:ems="15"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/password"
app:startIconDrawable="@drawable/ic_baseline_lock_24"
android:background="@color/white"
app:passwordToggleEnabled="true"
app:passwordToggleTint="@color/primaryColor"
android:layout_marginBottom="36dp">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:background="@color/white"
android:ems="15"/>
</com.google.android.material.textfield.TextInputLayout>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="13dp"
android:text="@string/login_name"/>
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/form"
android:layout_marginTop="9dp"
android:layout_centerHorizontal="true">
<TextView
android:id="@+id/signin"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/primaryColor"
android:text="@string/dont_have"/>
</LinearLayout>
</RelativeLayout>
You will also notice, there’s an ImageView which you can replace with your own project image by simply adding it to the drawable folder:
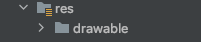
7. Let’s add the code for Registration as follows:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<LinearLayout
android:id="@+id/layout_first"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:gravity="center"
android:orientation="vertical"
android:layout_marginTop="18dp">
<ImageView
android:layout_width="117dp"
android:padding="3dp"
android:layout_height="90dp"
android:src="@drawable/add" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="18sp"
android:layout_marginTop="18dp"
android:text="@string/wlc_sign_up"/>
</LinearLayout>
<LinearLayout
android:id="@+id/form"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingStart="27dp"
android:paddingEnd="27dp"
android:gravity="center"
android:layout_marginTop="36dp"
android:orientation="vertical"
android:layout_below="@+id/layout_first">
<com.google.android.material.textfield.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/username"
app:startIconDrawable="@drawable/ic_baseline_person_24"
android:background="@color/white"
android:layout_marginBottom="27dp">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:background="@color/white"
android:ems="15"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/email"
app:startIconDrawable="@drawable/ic_baseline_email_24"
android:background="@color/white"
android:layout_marginBottom="27dp">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textEmailAddress"
android:background="@color/white"
android:ems="15"/>
</com.google.android.material.textfield.TextInputLayout>
<com.google.android.material.textfield.TextInputLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/password"
app:startIconDrawable="@drawable/ic_baseline_lock_24"
android:background="@color/white"
app:passwordToggleEnabled="true"
app:passwordToggleTint="@color/primaryColor"
android:layout_marginBottom="36dp">
<com.google.android.material.textfield.TextInputEditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:inputType="textPassword"
android:background="@color/white"
android:ems="15"/>
</com.google.android.material.textfield.TextInputLayout>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="13dp"
android:text="@string/login_name"/>
</LinearLayout>
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/form"
android:layout_marginTop="9dp"
android:layout_centerHorizontal="true">
<TextView
android:id="@+id/signup"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textColor="@color/primaryColor"
android:text="@string/already_member"/>
</LinearLayout>
</RelativeLayout>
8. Add the following to strings.xml file
<string name="login_name">Sign in</string>
<string name="register_name">Sign up</string>
<string name="wlc_sign_in">Welcome back, sign in</string>
<string name="wlc_sign_up">Sign up to continue</string>
<string name="email">Email</string>
<string name="password">Password</string>
<string name="already_member">Already have an account, Sign in</string>
<string name="dont_have">Don\'t have an account, Sign up</string>
<string name="username">Username</string>
9. Finally add relevant colours to colors.xml file
<color name="primaryColor">@color/darkIndigo</color>
<color name="buttonColor">#800000</color>
<color name="diamondDust">#f8f5e6</color>
<color name="gray">#999</color>
<color name="darkIndigo">#171d4b</color>
<color name="gooseBerry">#afe19f</color>
10. Run the app. You should have something like this:
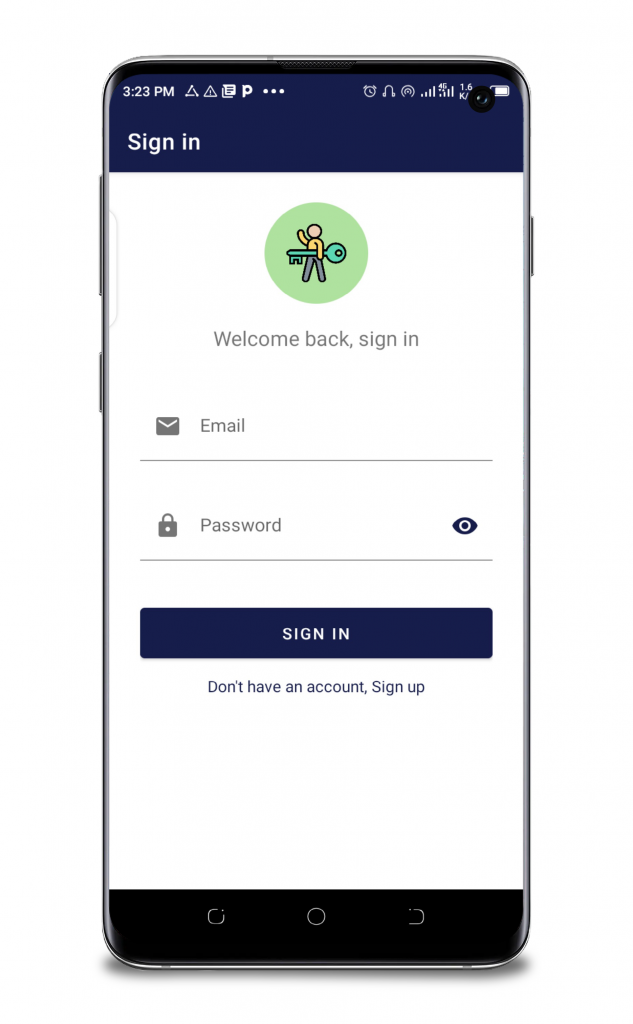
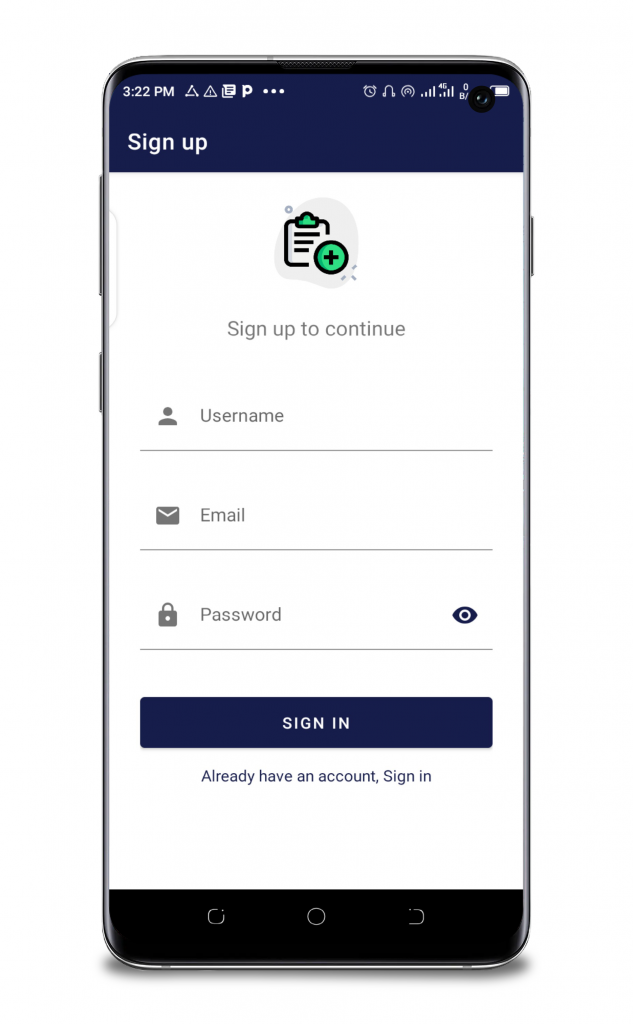
Why the Confirm Password Field is Useless
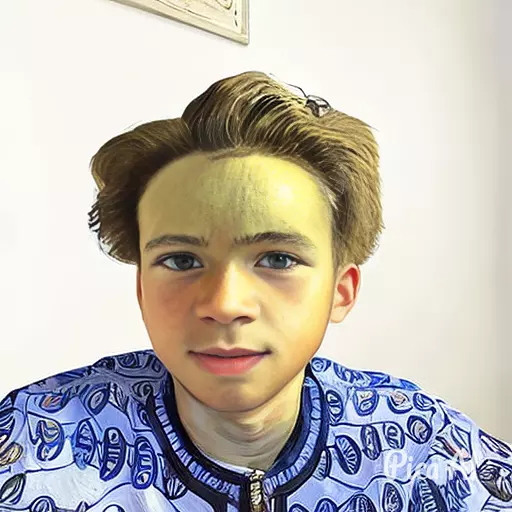