A string in Javascript represents a sequence of characters.
Strings are useful for holding data that can be represented in textual forms. Some of the most-used operations on strings are to check their length
, to build and concatenate them using the + and += string operators, checking for the existence or location of substrings with the indexOf()
method, or extracting substrings with the substring()
method.
Whatever manipulation you need to do with Strings here are 12 Javascript String methods you can always reference.
1. search()
The search()
method matches a string against a regular expression **
It returns the index (position) of the first match.
The search()
method returns -1 if no match is found.
The search()
method is also case sensitive.
Example:
const str = "The quick brown fox jumps over the lazy dog";
str.search(/[^\w\s]/g);
2. split()
The split()
method splits a string into an array of substrings.
The split()
method returns the new array.
The split()
method does not change the original string.
If (” “) is used as separator, the string is split between words.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.split(',');
3. repeat()
The repeat()
method returns a string with a number of copies of a string.
The repeat()
method returns a new string.
The repeat()
method does not change the original string.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.repeat(3);
4. length
The length
property returns the length of a string.
The length
property of an empty string is 0.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.length;
5. replace()
The replace()
method searches a string for a value or a regular expression.
The replace()
method returns a new string with the value(s) replaced.
The replace()
method does not change the original string.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.replace('o', 'a');
6. indexOf()
The indexOf()
method returns the position of the first occurrence of a value in a string.
The indexOf()
method returns -1 if the value is not found.
The indexOf()
method is case sensitive.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.indexOf('fox');
7. charAt()
The charAt()
method in Ja returns the character at a specified index (position) in a string.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.indexOf('fox');
8. startsWith()
The startsWith()
method returns true
if a string starts with a specified string, otherwise it returns false
. The startsWith()
method is case sensitive.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.startsWith("The");
9. toUpperCase()
The toUpperCase()
method converts a string to uppercase letters and does not change the original string.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.toUpperCase();
10. toLowerCase()
The toLowerCase()
method converts a string to uppercase letters and does not change the original string.
Example
const str = "The quick brown fox jumps over the lazy dog";
str.toLowerCase();
11. match()
The match()
method matches a string against a regular expression **
The match()
method returns an array with the matches.
The match()
method returns null if no match is found.
const str = "The quick brown fox jumps over the lazy dog";
str.match(/[A-Z]/g);
See Also:
How to Shorten Strings With Three Dots
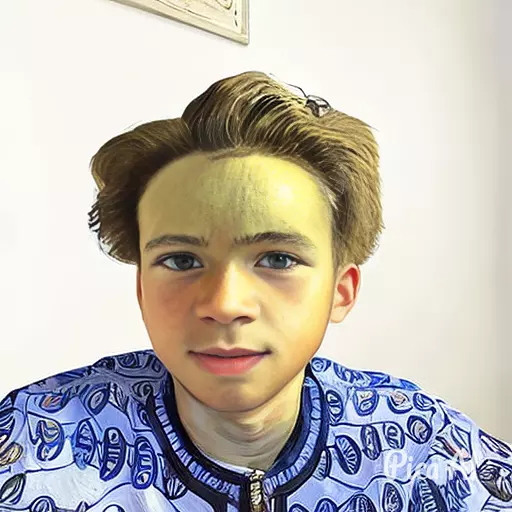