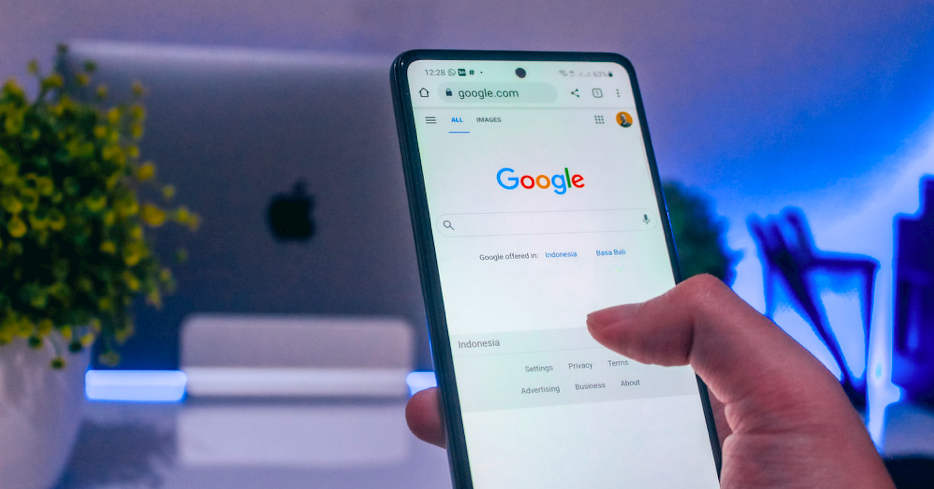
You can use JavaScript to search for a particular word, item or phrase in API. This can be done in a step-step process as follows:
- Design your search bar. This is where the user will type whatever they want to search from the given endpoint
<form>
<input id="search" type="text" placeholder="search destination" />
<button id="button" type="button">Search</button>
</form>
2. Next, you need to define where you want the search results to show. This is usually within your body tag. You can define a `section` tag for that and style it accordingly
<section>
<div id="content"></div>
</section>
3. The next thing to do after you have determined where to show your results is to consume the given endpoint and loop through the results accordingly. Here I’m going to consume data from a localhost endpoint. If you’re looking for how to work with live API, we created a JavaScript news application you can check out.
<script>
const searchInput = document.getElementById("search")
const button = document.getElementById("button")
const content = document.getElementById("content")
let html = []
button.addEventListener("click", (e) => {
const value = e.target.value.toLowerCase()
console.warn(value);
html.forEach(post => {
const isVisible =
searchInput.toLowerCase().includes(value)
// posts.element.classList.toggle("hide", !isVisible)
})
content.innerHTML = "" //set to empty before loading new search results
fetch(`http://192.168.64.2/project/api/search.php`, {
method:'POST',
body: JSON.stringify({
search: `${searchInput.value}`,
}),
headers: {
'Content-Type': 'application/json; charset=UTF-8'
},
})
.then((response) => response.json())
.then(data => {
console.log(data);
if(data !== "no records were found"){
const html = data.map(item => {
return `
<div class="card">
<div class="image" style="background-image:url('${item.jetpack_featured_media_url}')">
</div>
<div class="title-container">
<p class="title">
${item.title.rendered}
</p>
<div class="price-badge">
<p class="price">
${item.date}
</p>
</div>
</div>
<p class="content" style="margin-top: 18px">
${item.excerpt.rendered}
</p>
<a href="destination.php?id=${item.id}">
<div class="button-center">
<div class="more"><b>Read more </b> <i class="fas fa-angle-right"></i></div>
</div>
</a>
</div>
`
}).join(' ');
console.log(html);
document.querySelector('#content').insertAdjacentHTML('beforeend', html);
}else{
document.querySelector('#content').innerHTML = "No records found";
}
})
})
</script>
If you study the code, you will observe that we are saving our response in the array variable `html` and then we’re looping through the array to show the result. We’re also checking whether or not the endpoint returns any result.
This is how you can use JavaScript to perform search function on an API. Feel free to try it out and let me know what you think in the comments.