Object Oriented Programming (OOP) is a way of writing code such that you can create different objects (also called “instances”) from a common blueprint.
Each created instance has properties that are not shared with other instances.
For example if we have an Animal blueprint, we can create other animal instances with different names.
Typically, object oriented programming is about structuring your program such that you have multiple levels of blueprints. This is also referred to as “inheritance” or “subclassing“.
Another aspect of object oriented programming involves a process whereby we hide certain pieces of information so that they are not accessible and cannot be written to. This process is known as “encapsulation“.
For the sake of this tutorial, we shall look at two (2) approaches to objected-oriented programming which are:
- Constructor functions.
- Class Syntax
Constructor Functions
Constructors are special functions that contain “this” keywords.
“this” lets you store and access unique values created for each instance, and this instance can be created using the “new” keyword.
function Human(firstName, lastName){
this.firstName = firstName;
this.lastName = lastName;
}
const human = new Human("Lawson", "Luke");
console.log(human.firstName); //Lawson
console.log(human.lastName); //Luke
Class Syntax
Classes, which many have referred to as “syntactic sugar”, are templates for creating objects.
Classes are an alternative of constructor functions and can be defined using the “class” keyword followed by the desired name of the class.
class Human {
}
Constructor Methods in Classes
The constructor method is a special method for creating and initialising an object created with a class.
There can only be just one special method with the name “constructor” in a class, and if more than one occurrence exist, a syntaxError will be thrown.
Class Human {
constructor(firstName, lastName){
this.firstName = firstName;
this.lastName = lastName;
}
}
const human = new Human("Matt" "Hendrick");
console.log(human.firstName); //Matt
console.log(human.lastName); //Hendrick
Hoisting
Hoisting means calling a variable or function before it is declared.
Well, this happens because Javascript has a default behaviour of moving declarations to the top, regardless of where it is declared.
But this is not the same with classes.
You first need to declare your class before you can access it, otherwise a ReferenceError will be thrown.
Conclusion
Object oriented programming is an exciting way of writing code, and it cuts across several programming languages like PHP for instance.
However, you should use this concept sparingly, especially when your codebase is small and not very complex.
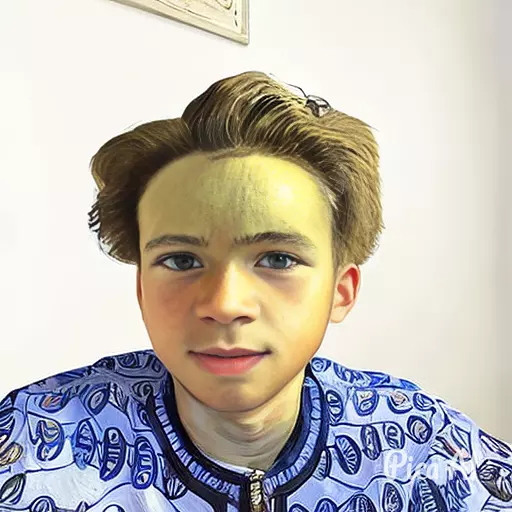