When building software applications there are scenarios where you will want to upload and instantly preview the uploaded image(s). Before we delve into the code aspect, here are a few reasons why you might want to add the image preview functionality to your application.
Benefits of Instantly Previewing Images
- Enhanced User Experience: Providing a preview of an image before uploading or processing allows users to confirm they’ve selected the correct file. It enhances the user experience by reducing errors and ensuring the selected image meets their expectations.
- Validation and Error Handling: Image previewing can help in validating the selected image before any action is taken. It allows for early error detection, such as incorrect file formats or corrupted files, preventing unnecessary server requests or processing.
- Improved Interactivity: Displaying a preview of the image in real-time as the user selects or adjusts it adds an interactive element to the application. Users can make informed decisions about the image they want to upload or modify.
- Feedback and Confirmation: Seeing a visual representation of the selected image provides users with immediate feedback and confirmation. It assures them that the chosen image is the one they intended to upload or process.
- Customization and Cropping: In more advanced scenarios, image previews can be used to allow users to crop, resize, or make adjustments to the image before final submission, offering a more tailored experience.
- Reduced Server Load: By enabling users to preview images before uploading, unnecessary server requests for incorrect or undesirable images can be avoided, reducing server load and bandwidth usage.
- User Empowerment: Giving users the ability to preview images aligns with user-centric design principles, empowering them to make informed decisions and enhancing their sense of control within the application.
How to Preview Image in React
<div class="container">
<h2>Upload Image:</h2>
<input type="file" onChange={handleChange} /><br />
<img src={file} style={{ maxWidth: '100%', height: 'auto' }}/>
</div>
Next, we want to define the handleChange function.
const [file, setFile] = useState();
function handleChange(e) {
console.log(e.target.files);
setFile(URL.createObjectURL(e.target.files[0]));
}
Full code
import { useState, useEffect } from 'react';
const Home = () => {
const [file, setFile] = useState();
function handleChange(e) {
console.log(e.target.files);
setFile(URL.createObjectURL(e.target.files[0]));
}
return(
<div class="container">
<h2>Upload Image:</h2>
<input type="file" onChange={handleChange} /><br />
<img src={file} style={{ maxWidth: '100%', height: 'auto' }}/>
</div>
)
}
export default Home
Next, we want to instantly preview uploaded image in React JS. Here’s what the code looks like:
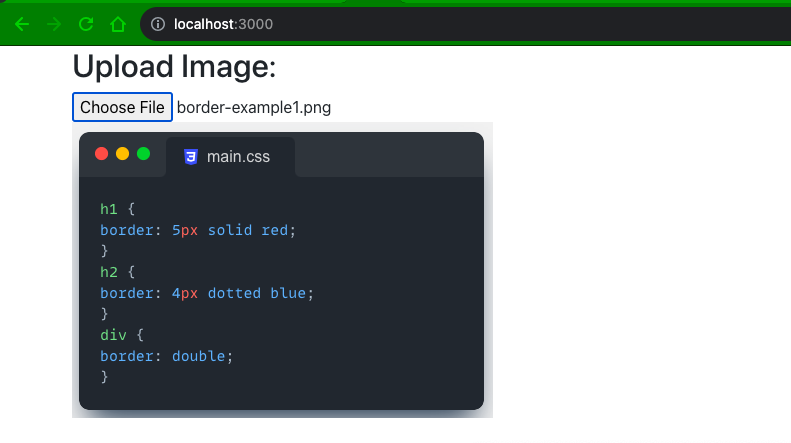
We have done similar image upload and preview with PHP’s PDO and JQuery
Here is how to instantly preview uploaded image in React JS
Conclusion
In summary, incorporating image previews within an application contributes significantly to user satisfaction, error prevention, and overall usability, ensuring a smoother and more efficient user experience.
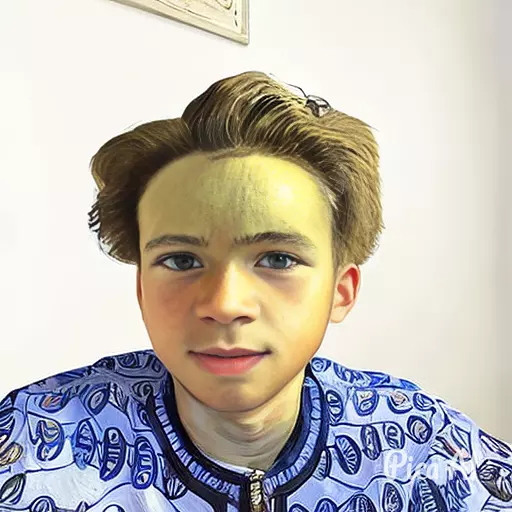