The HTML Geolocation API is used to locate a user’s geographical position. For this to work accurately and not compromise privacy, the user would have to give consent and approval.
The Geolocation API is supported by major browsers including Chrome, Safari, Mozilla Firefox, Edge and Opera.
Fast Track Your Mobile App Development Process
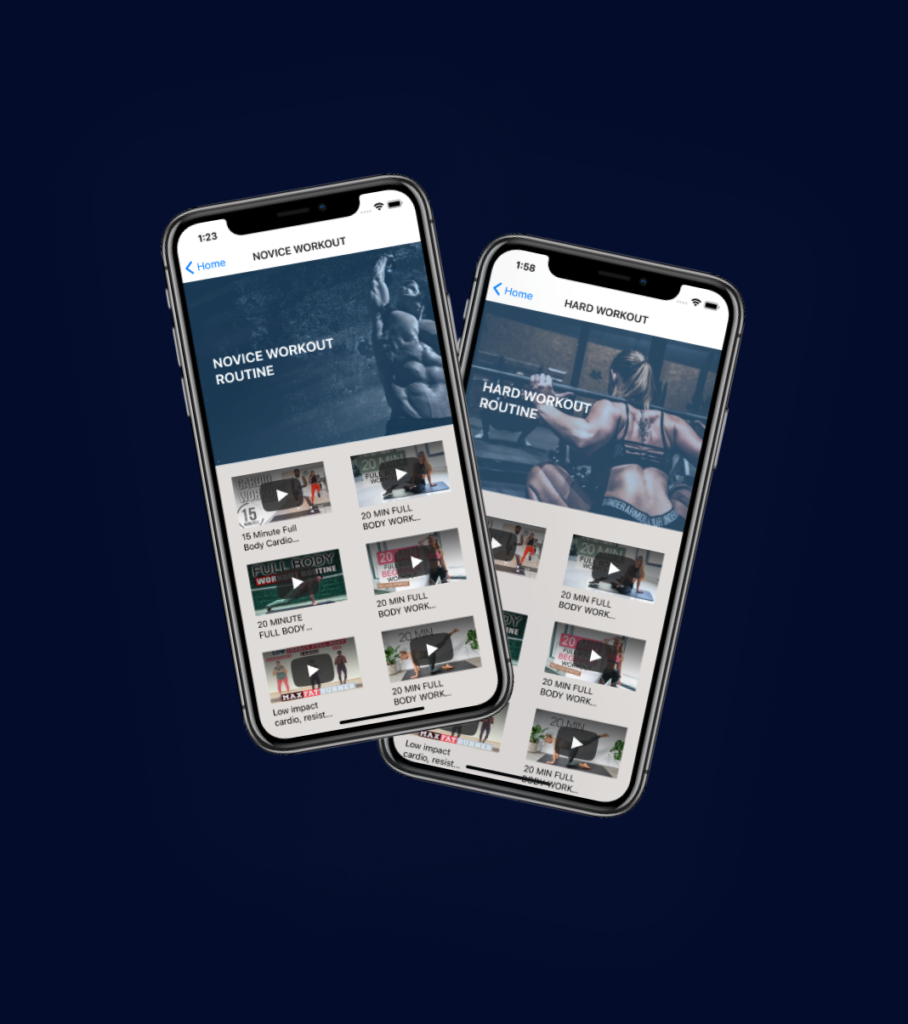
How to Use the Geolocation API
The geolocation is made available through the navigator.geolocation object. This means it has properties that could be called to get certain information.
When using the Geolocation API, the first thing we want to do is to check if it is supported by the user’s browser. If it is, then we can proceed. If not, we return a message to the user.
if ('geolocation' in navigator) {
/* geolocation is available */
} else {
/* geolocation IS NOT available */
}
Getting the Current Position
The getCurrentPosition returns the user’s current position.
This initiates an asynchronous request to detect the user’s position, and queries the positioning hardware to get up-to-date information. After the position is determined, the defined callback function is executed.
You can optionally provide a second callback function to be executed if an error occurs and also a third optional parameter where you can set the maximum age of the position returned, the time to wait for a request, and if you want high accuracy for the position.
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
x.innerHTML = "Latitude: " + position.coords.latitude +
"<br>Longitude: " + position.coords.longitude;
}
</script>
Error Handling
The getCurrentPosition() method takes in a second parameter that handles error. This error function is expected to run if the application fails to get the user’s position.
function showError(error) {
switch(error.code) {
case error.PERMISSION_DENIED:
x.innerHTML = "User denied the request for Geolocation."
break;
case error.POSITION_UNAVAILABLE:
x.innerHTML = "Location information is unavailable."
break;
case error.TIMEOUT:
x.innerHTML = "The request to get user location timed out."
break;
case error.UNKNOWN_ERROR:
x.innerHTML = "An unknown error occurred."
break;
}
}
We can then add this parameter to our function.
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition, showError);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
Watching the Current Position
The watchPosition() is used to actively listen for a change in position. This works best with a GPS or a smartphone so that the change in position or the movement can be monitored.
<script>
var x = document.getElementById("demo");
function getLocation() {
if (navigator.geolocation) {
navigator.geolocation.watchPosition(showPosition);
} else {
x.innerHTML = "Geolocation is not supported by this browser.";
}
}
function showPosition(position) {
x.innerHTML = "Latitude: " + position.coords.latitude +
"<br>Longitude: " + position.coords.longitude;
}
</script>
The getCurrentPosition() Method Properties
The getCurrentPosition() method also returns other properties that are worthy of note.
Property | Result |
coords.latitude | The latitude as a decimal number (always returned) |
coords.longitude | The longitude as a decimal number (always returned) |
coords.accuracy | The accuracy of position (always returned) |
coords.altitude | The altitude in meters above the mean sea level (returned if available) |
coords.altitudeAccuracy | The altitude accuracy of position (returned if available) |
coords.heading | The heading as degrees clockwise from North (returned if available) |
coords.speed | The speed in meters per second (returned if available) |
timestamp | The date/time of the response (returned if available) |
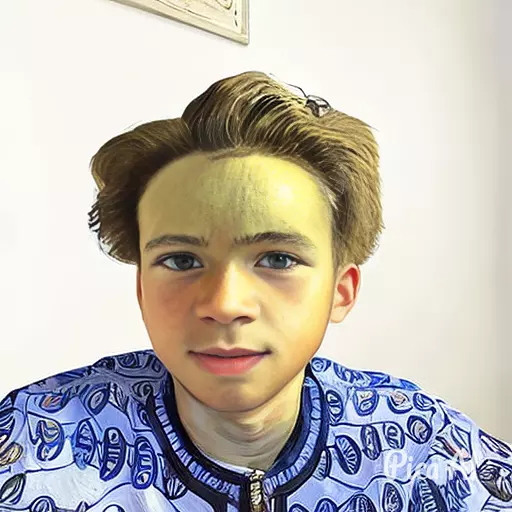