The FlatList component is an effective way to display items in a scrollable list view. We can fetch data from an API and display in FlatList in React Native. It is a useful alternative to ScrollViews, especially when dealing with large data sets.
Flat lists, has the following features:
- Fully cross-platform.
- Optional horizontal mode.
- Configurable viewability callbacks.
- Header support.
- Footer support.
- Separator support.
- Pull to Refresh.
- Scroll loading.
- ScrollToIndex support.
- Multiple column support.
In this article, we shall see how we can fetch data from an endpoint and display the data using FlatList in React Native.
So, I will assume that you already know how to create a new React Native application. If you’re just getting started, you can check out the article on creating a new React Native project here.
Here’s what a new React Native application should look like:
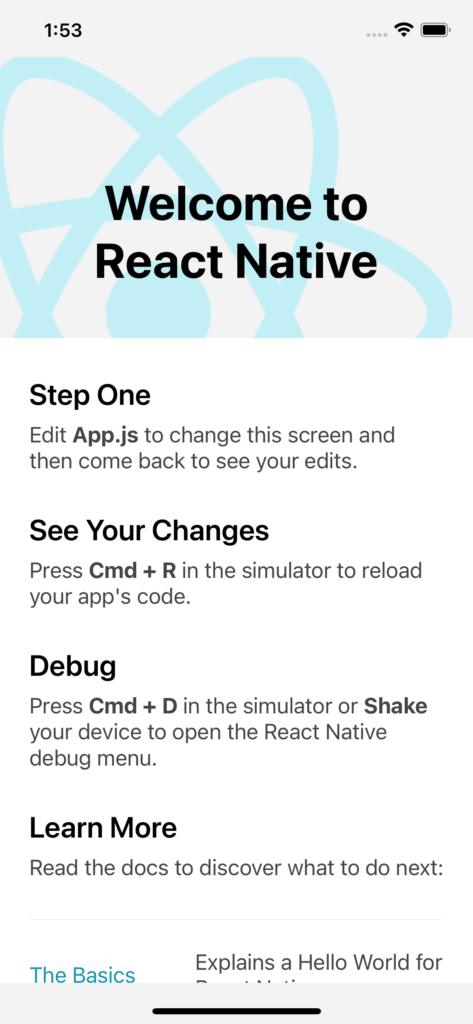
We are going to be fetching data from the Cat Facts API. So we will write our React Native code as follows:
fetchItem() {
requestAnimationFrame(() =>
fetch(`https://catfact.ninja/breeds`, {
method: 'GET',
})
.then(response => response.json())
.then(responseJson => {
console.warn(responseJson);
})
.catch(error => {
{
alert(error)
}
}),
);
}
componentDidMount(){
this.fetchItem()
}
Let’s explain what’s happening here.
Here, we’re calling the endpoint https://catfact.ninja/breeds and logging the response to at least confirm that we’re even getting a response. We’re then calling that method in our componentDidMount()
Here’s the response from the call
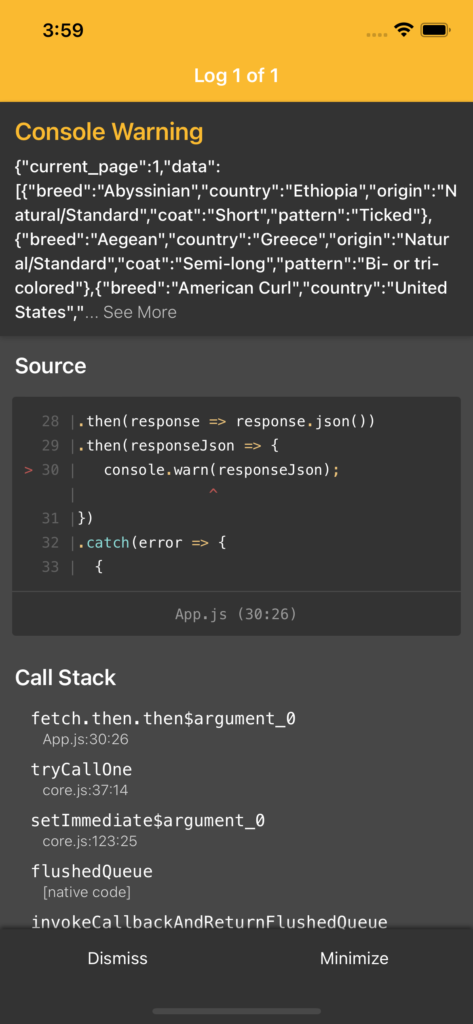
Moving on, let’s render just the breeds and display without any form of styling. We should be able to see something on the screen.
renderCats = ({item}) => (
<View style={{marginTop: 0}}>
<Text>{item.breed}</Text>
</View>
);
<FlatList
removeClippedSubviews={true}
data={this.state.data}
renderItem={item => this.renderCats(item)}
/>
So here, we’re getting the items and we’re displaying in our FlatList. Here is what it looks like:
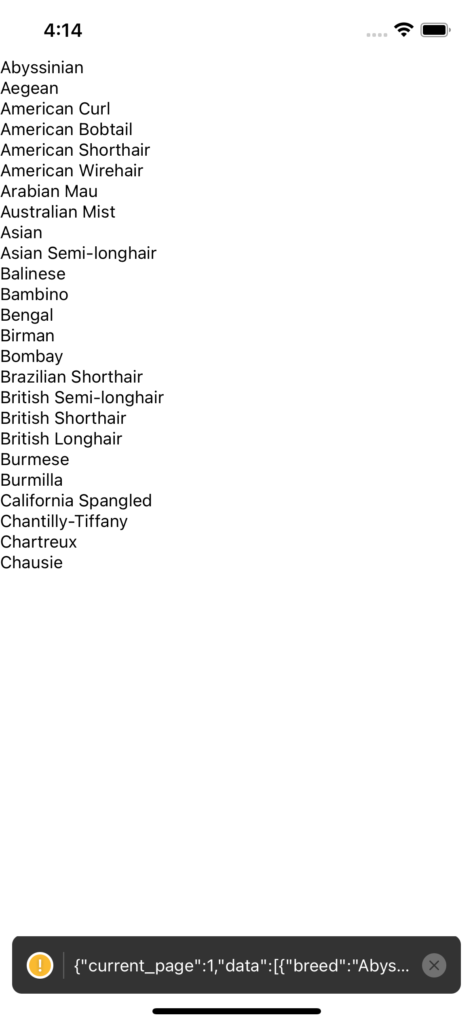
Next let’s use ListView from react-native-elements
yarn add react-native-elements
yarn add react-native-vector-icons // Add icons
cd iOS
pod install
renderCats = ({item}) => (
<View style={{marginTop: 0}}>
<ListItem bottomDivider containerStyle={{ backgroundColor: '#fbb03c' }}>
<Avatar rounded large source={{uri: 'https://cdn-prod.medicalnewstoday.com/content/images/articles/322/322868/golden-retriever-puppy.jpg'}} height={36} width={36} />
<ListItem.Content>
<ListItem.Title style={{color:'black', fontSize: 18}}>{item.breed.toUpperCase()}</ListItem.Title>
<ListItem.Subtitle style={{color: 'black'}}>{item.country}</ListItem.Subtitle>
</ListItem.Content>
</ListItem>
</View>
);
We also add a bit of styling to it.
/**
* Sample React Native App
* https://github.com/facebook/react-native
*
* @format
* @flow strict-local
*/
import React, { PureComponent } from 'react';
import {
StatusBar,
StyleSheet,
SafeAreaView,
Text,
View,
TouchableOpacity,
FlatList
} from 'react-native';
import { ListItem, Avatar } from 'react-native-elements';
import Icon from 'react-native-vector-icons/Ionicons'
class App extends PureComponent {
constructor(props){
super(props);
this.state = {
data: []
}
}
fetchItem() {
requestAnimationFrame(() =>
fetch(`https://catfact.ninja/breeds`, {
method: 'GET',
})
.then(response => response.json())
.then(responseJson => {
this.setState({data: responseJson.data})
console.warn(responseJson);
})
.catch(error => {
{
alert(error)
}
}),
);
}
renderCats = ({item}) => (
<View style={{marginTop: 0}}>
<ListItem bottomDivider containerStyle={{ backgroundColor: '#fbb03c' }}>
<Avatar rounded large source={{uri: 'https://cdn-prod.medicalnewstoday.com/content/images/articles/322/322868/golden-retriever-puppy.jpg'}} height={36} width={36} />
<ListItem.Content>
<ListItem.Title style={{color:'black', fontSize: 18}}>{item.breed.toUpperCase()}</ListItem.Title>
<ListItem.Subtitle style={{color: 'black'}}>{item.country}</ListItem.Subtitle>
</ListItem.Content>
</ListItem>
</View>
);
componentDidMount(){
this.fetchItem()
}
render(){
return(
<SafeAreaView style={{ flex: 1, backgroundColor: '#fbb03c' }}>
<FlatList
removeClippedSubviews={true}
data={this.state.data}
renderItem={item => this.renderCats(item)}
/>
</SafeAreaView>
)
}
}
export default App;
Here’s the full source code on our Github Repo. Don’t forget to follow us on Github and our social media handles @codeflaretech.
Don’t forget to follow
TADA!
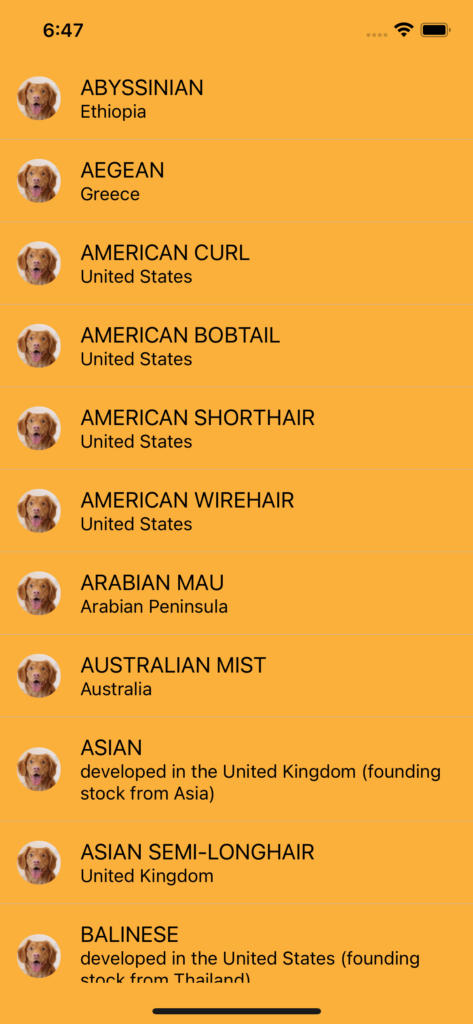
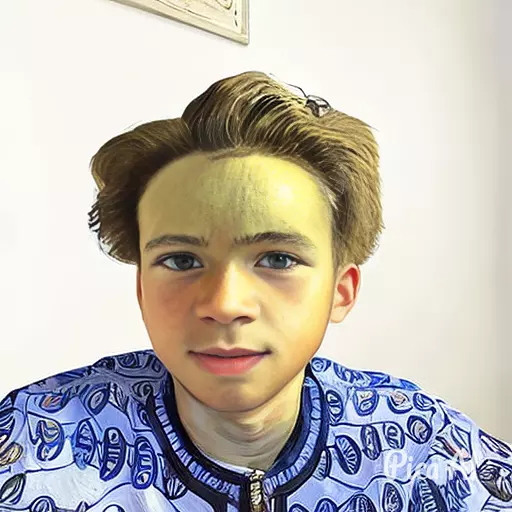