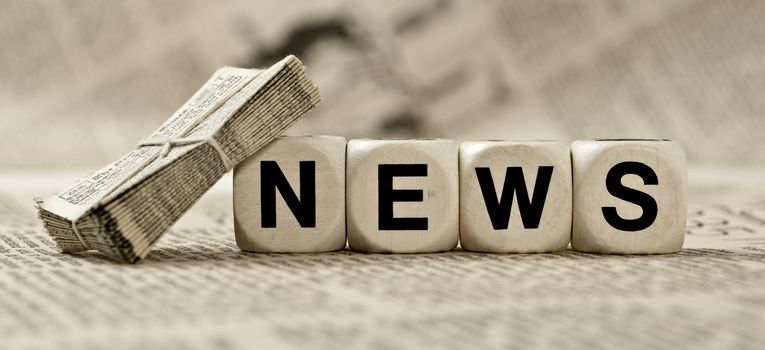
In this tutorial, we are going to build a news app with HTML, Bootstrap and ES6 features of javascript.
We will also being using a free news endpoint from techcruch.
Let’s get started.
We will be working with this endpoint: https://techcrunch.com/wp-json/wp/v2/posts?per_page=100&context=embed
The first thing we’ll do is to create our HTML holding tags and add our Bootstrap dependency
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
<title></title>
</head>
<body>
<div class="container">
<center>
<div class="spinner-border" role="status" id="loading"></div>
</center>
<div class="row row-cols-1 row-cols-md-3 g-4" id="news"></div>
</div>
</body>
Next, we will write our Javascript code that consumes the endpoint
window.addEventListener('load', (event) => {
const api_url =
"https://techcrunch.com/wp-json/wp/v2/posts?per_page=100&context=embed";
// Defining async function
async function getapi(url) {
// Storing response
const response = await fetch(url);
// Storing data in form of JSON
var data = await response.json();
console.log(data);
if (response) {
hideloader();
}
show(data);
}
// Calling that async function
getapi(api_url);
})
Let’s do some explanation here.
Here we’re loading our endpoint once the webpage loads that’s why we’re using the load event listener, and we’re using Fetch API to get our response. Now when the page loads, we also have a spinner running simultaneously as well. Then we’re saying, if we have gotten a response, we want to hide the spinner. That’s why we calling the function hideloader()
We’re also have a function show(data) which will be showing our response. But first we have to define it.
for (let item of data) {
// let description = item.course_description.substring(0, 100).concat('...')
tab += `
<div class="col-12 col-md-6 mb-6 col-lg-4 d-flex">
<div class="card shadow-light-lg mb-6 mb-md-0 lift lift-lg">
<img src=${item.jetpack_featured_media_url} alt="" class="card-img-top">
<div class="card-body position-relative">
<div class="position-relative text-right mt-n8 mr-n4 mb-3">
<span class="badge badge-pill badge-success">
<span class="h6 text-uppercase"><i class="fa fa-check"></i></span>
</span>
</div>
<h3 class="text-capitalize" style="font-family:poppins" >
${item.title.rendered}
</h3>
<p class="text-muted">
${item.excerpt.rendered}
</p>
<button type="button" href="#showModal" id=${item.course_id} data-toggle="modal" class="btn btn-danger font-weight-bold text-decoration-none" onclick="showCourses(this.id)">
Read more <i class="fe fe-arrow-right ml-3"></i>
</button>
<p>${item.date}</p>
</div>
</div>
</div>
`;
}
// Setting innerHTML as tab variable
document.getElementById("news").innerHTML = tab;
Here, we are looping through our response and presenting them in our html.
Here’s the FULL WORKING CODE
<!DOCTYPE html>
<html lang="en" dir="ltr">
<head>
<meta charset="utf-8">
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
<title></title>
</head>
<body>
<div class="container">
<center>
<div class="spinner-border" role="status" id="loading"></div>
</center>
<div class="row row-cols-1 row-cols-md-3 g-4" id="news"></div>
</div>
<script>
window.addEventListener('load', (event) => {
const api_url =
"https://techcrunch.com/wp-json/wp/v2/posts?per_page=100&context=embed";
// Defining async function
async function getapi(url) {
// Storing response
const response = await fetch(url);
// Storing data in form of JSON
var data = await response.json();
console.log(data);
if (response) {
hideloader();
}
show(data);
}
// Calling that async function
getapi(api_url);
// Function to hide the loader
function hideloader() {
document.getElementById('loading').style.display = 'none';
}
// Function to define innerHTML for HTML table
function show(data) {
let tab = ``;
// Loop to access all rows
for (let item of data) {
// let description = item.course_description.substring(0, 100).concat('...')
tab += `
<div class="col-12 col-md-6 mb-6 col-lg-4 d-flex">
<div class="card shadow-light-lg mb-6 mb-md-0 lift lift-lg">
<img src=${item.jetpack_featured_media_url} alt="" class="card-img-top">
<div class="card-body position-relative">
<div class="position-relative text-right mt-n8 mr-n4 mb-3">
<span class="badge badge-pill badge-success">
<span class="h6 text-uppercase"><i class="fa fa-check"></i></span>
</span>
</div>
<h3 class="text-capitalize" style="font-family:poppins" >
${item.title.rendered}
</h3>
<p class="text-muted">
${item.excerpt.rendered}
</p>
<button type="button" href="#showModal" id=${item.course_id} data-toggle="modal" class="btn btn-danger font-weight-bold text-decoration-none" onclick="showCourses(this.id)">
Read more <i class="fe fe-arrow-right ml-3"></i>
</button>
<p>${item.date}</p>
</div>
</div>
</div>
`;
}
// Setting innerHTML as tab variable
document.getElementById("news").innerHTML = tab;
}
})
</script>
</body>
</html>
Result:
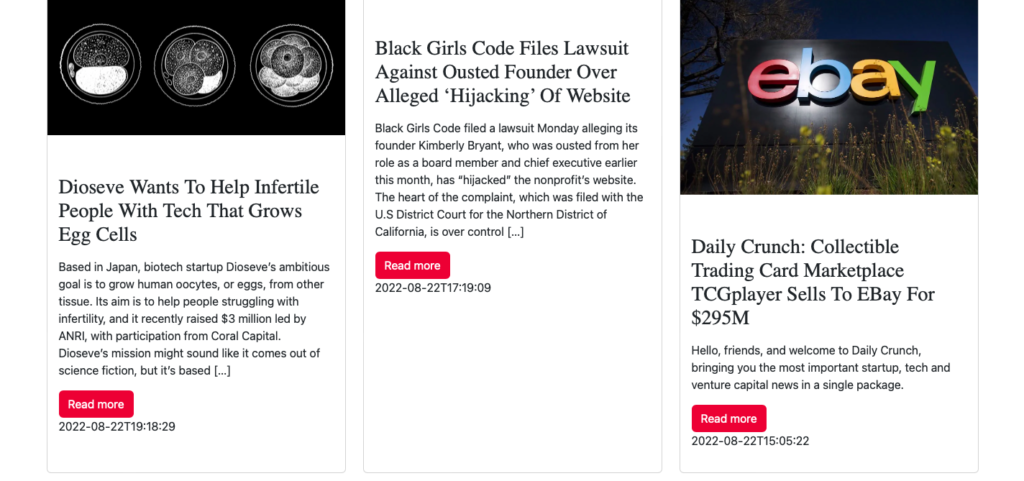
So here’s the full result in its basic usage. If you would like to format the date to a more readable format, we’ve covered how to use moment.js here