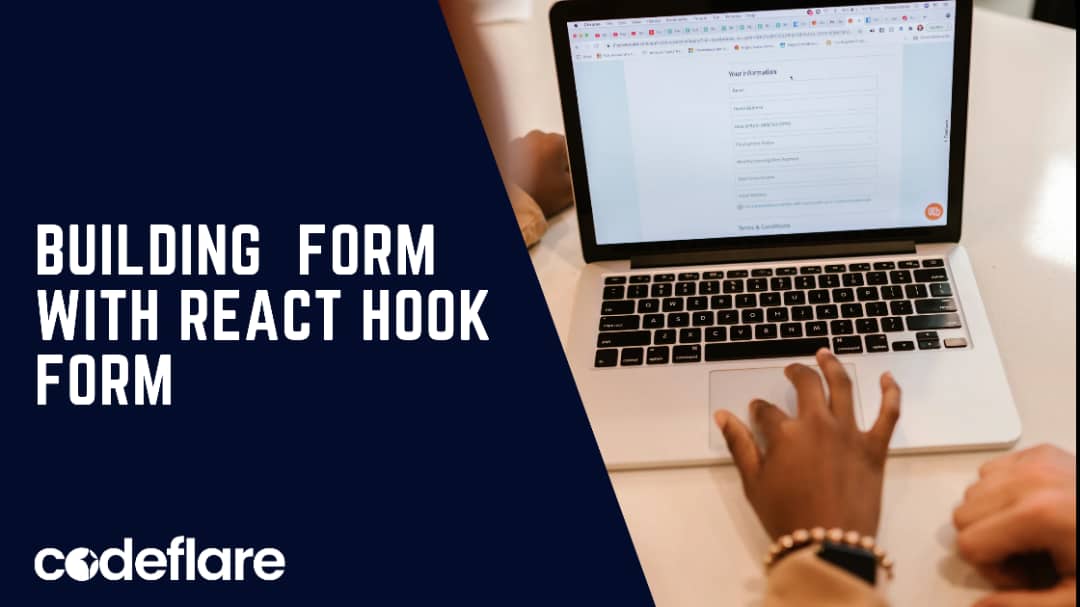
When it comes to building forms in React, handling state and validation can become a complex task. React Hook Form is a powerful and simple solution for managing forms efficiently in React applications. It reduces re-renders, is easy to use, and integrates well with modern form validation libraries. In this article, we’ll dive into why and how you can build forms with React Hook Form.
Why Use React Hook Form?
Before jumping into the code, let’s explore why React Hook Form is a popular choice for managing forms:
- Minimal Re-renders: React Hook Form uses uncontrolled components, meaning it doesn’t require frequent re-renders when managing form state.
- Easy Validation: You can easily integrate with validation libraries like Yup or use custom validation.
- Tiny Bundle Size: It has a small footprint, keeping your project light.
- Improved Performance: Handling large forms with many fields is efficient due to its optimized structure.
- Great Developer Experience: Simple API and a variety of features that enhance productivity.
Setting Up React Hook Form
To get started, first install the package:
npm install react-hook-form
Once installed, let’s begin by creating a basic form.
Step 1: Create a Simple Form
Here’s an example of how to create a simple form with React Hook Form.
import React from 'react';
import { useForm } from 'react-hook-form';
const SimpleForm = () => {
const { register, handleSubmit, formState: { errors } } = useForm();
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label>Name</label>
<input {...register("name", { required: true })} />
{errors.name && <p>Name is required</p>}
</div>
<div>
<label>Email</label>
<input {...register("email", {
required: "Email is required",
pattern: {
value: /^\S+@\S+$/i,
message: "Entered value does not match email format"
}
})} />
{errors.email && <p>{errors.email.message}</p>}
</div>
<button type="submit">Submit</button>
</form>
);
};
export default SimpleForm;
Breakdown of the Code
- useForm: This hook provides functions to register input fields, handle form submissions, and manage form state.
- register: Used to register the form inputs. In this example, the
name
andemail
fields are registered. - handleSubmit: This function processes the form submission. It prevents default behavior and returns the form values.
- errors: This object is automatically populated when validation errors occur, and it helps display custom error messages.
Step 2: Form Validation with Yup
React Hook Form works seamlessly with Yup, a JavaScript schema builder for value parsing and validation. Let’s integrate Yup into the form to provide more robust validation.
First, install Yup and the resolver for React Hook Form:
npm install @hookform/resolvers yup
Then, set up the validation schema and connect it to React Hook Form:
import React from 'react';
import { useForm } from 'react-hook-form';
import { yupResolver } from '@hookform/resolvers/yup';
import * as yup from 'yup';
const schema = yup.object().shape({
name: yup.string().required("Name is required"),
email: yup.string().email("Invalid email format").required("Email is required")
});
const FormWithYupValidation = () => {
const { register, handleSubmit, formState: { errors } } = useForm({
resolver: yupResolver(schema)
});
const onSubmit = (data) => {
console.log(data);
};
return (
<form onSubmit={handleSubmit(onSubmit)}>
<div>
<label>Name</label>
<input {...register("name")} />
{errors.name && <p>{errors.name.message}</p>}
</div>
<div>
<label>Email</label>
<input {...register("email")} />
{errors.email && <p>{errors.email.message}</p>}
</div>
<button type="submit">Submit</button>
</form>
);
};
export default FormWithYupValidation;
Key Changes:
- yupResolver: This resolver is used to integrate Yup with React Hook Form, making it easier to manage complex validation rules.
- schema: The Yup schema defines the structure and validation rules for each form field.
Step 3: Managing Complex Forms
React Hook Form also makes it easy to manage more complex forms, such as those with dynamic fields, multiple steps, or file uploads.
Handling Dynamic Fields
For dynamically adding or removing fields, you can use the useFieldArray hook:
import { useFieldArray } from 'react-hook-form';
const { fields, append, remove } = useFieldArray({
control,
name: "users"
});
return (
<div>
{fields.map((field, index) => (
<div key={field.id}>
<input {...register(`users.${index}.name`)} />
<button type="button" onClick={() => remove(index)}>Remove</button>
</div>
))}
<button type="button" onClick={() => append({ name: '' })}>Add User</button>
</div>
);
Conclusion
Building forms with React Hook Form is a fantastic solution for React applications. Its optimized performance, ease of use, and seamless integration with validation libraries like Yup make it a go-to tool for form handling. Whether you’re working with simple or complex forms, building forms with React Hook Form simplifies the process and enhances the developer experience.
Integrating it into your next project will enhance performance and form management, providing a smoother and more effective way to build interactive and robust forms.