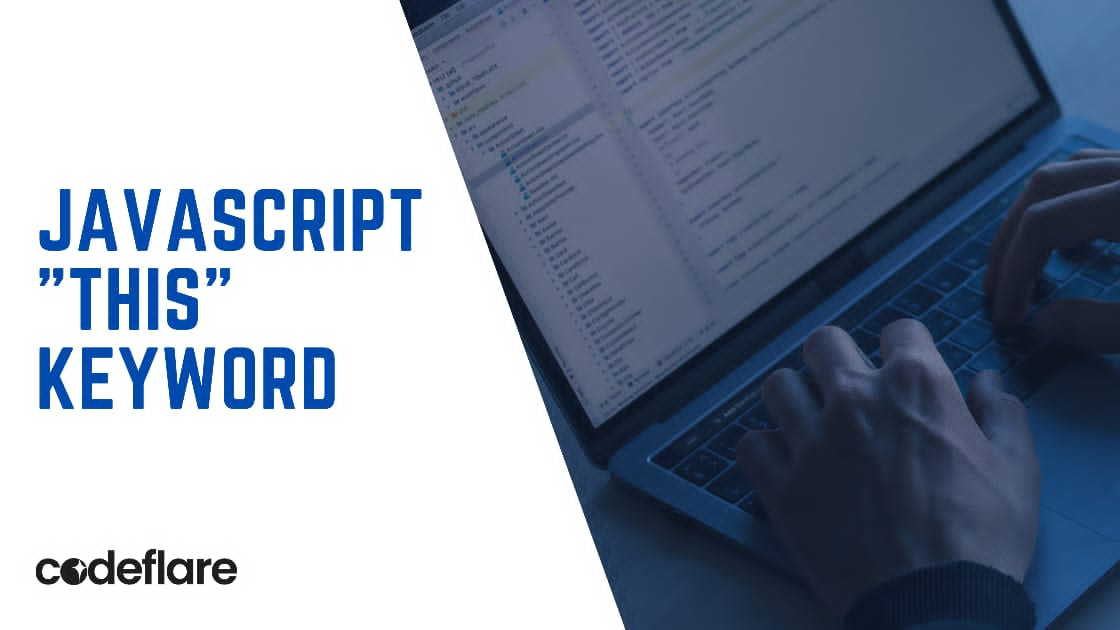
The this
keyword in JavaScript is one of the most powerful yet often confusing aspects of the language. It can behave differently depending on how and where it is used, making it essential for developers to understand its nuances. In this article, we’ll take a deep dive into the this
keyword, how it works, and the various contexts in which it operates.
What is the this
Keyword?
In JavaScript, this
keyword refers to the object that is executing the current function. However, depending on the context in which the function is called, the value of this
can change.
The value of this
is determined at runtime and can vary based on the following factors:
- Where and how a function is invoked.
- Whether strict mode is enabled.
- The type of function (e.g., regular functions vs. arrow functions).
Global Context
In the global execution context, this
points to the global object, which is window
in a browser.
console.log(this); // Window object in browsers
In this example, outside of any function, this
points to the global object, which is window
.
Function Context
In a function, this
behaves differently depending on how the function is invoked.
1. Regular Function Calls
When a function is called as a simple function, this
refers to the global object in non-strict mode, and undefined
in strict mode.
function show() {
console.log(this);
}
show(); // In non-strict mode: Window, in strict mode: undefined
2. Object Methods
When a function is called as a method of an object, this
refers to the object to which the method belongs.
const person = {
name: "John",
greet: function() {
console.log(this.name);
}
};
person.greet(); // "John"
In this case, this
inside greet()
refers to the person
object because the function is a method of that object.
Arrow Functions
One of the most critical differences in behavior comes when using arrow functions. Unlike regular functions, arrow functions do not have their own this
. Instead, they inherit this
from the surrounding lexical context.
const person = {
name: "Alice",
greet: () => {
console.log(this.name);
}
};
person.greet(); // undefined
Here, the arrow function does not bind this
to the person
object, resulting in undefined
.
Lexical Binding with Arrow Functions
Arrow functions are particularly useful when you want to maintain the value of this
from the enclosing context.
function Person() {
this.name = "John";
setTimeout(() => {
console.log(this.name); // Refers to the Person instance
}, 1000);
}
const p = new Person(); // Outputs "John" after 1 second
In this example, this
inside the arrow function refers to the Person
instance because arrow functions lexically bind this
.
Constructor Functions
In JavaScript, constructor functions are used to create objects. When a function is used as a constructor (called with new
), this
refers to the newly created object.
function Car(make, model) {
this.make = make;
this.model = model;
}
const car1 = new Car("Toyota", "Camry");
console.log(car1.make); // "Toyota"
In this case, this
refers to the new Car
object being created.
Explicit Binding
JavaScript allows you to explicitly bind this
using methods like call()
, apply()
, and bind()
.
call()
and apply()
Both call()
and apply()
allow you to invoke a function with a specific this
value. The difference between them lies in how they handle arguments: call()
takes arguments individually, while apply()
takes them as an array.
function greet() {
console.log(this.name);
}
const person = { name: "Emily" };
greet.call(person); // "Emily"
greet.apply(person); // "Emily"
Here, both call()
and apply()
explicitly set this
to the person
object.
bind()
The bind()
method returns a new function with a permanently bound this
.
const greetPerson = greet.bind(person);
greetPerson(); // "Emily"
In this example, bind()
creates a new function where this
is permanently set to person
.
Context vs. Scope
One common source of confusion is mixing up this
with scope. Scope refers to the accessibility of variables, whereas this
refers to the object that is currently executing the code. They are separate concepts, and understanding both is key to mastering JavaScript.
Strict Mode and this
When using strict mode ("use strict";
), the value of this
in a function is undefined
instead of the global object if not explicitly set.
"use strict";
function show() {
console.log(this);
}
show(); // undefined
This helps avoid common pitfalls and unintended behavior in code.
Conclusion
The this
keyword is central to many JavaScript functions and constructs. It can take on different values depending on the context in which it is used, making it crucial for developers to understand how it works in various situations. From object methods to arrow functions and explicit bindings with call()
, apply()
, and bind()
, mastering this
is a fundamental skill for writing clean, bug-free JavaScript code.
Implementing Code Splitting in React with React.Lazy