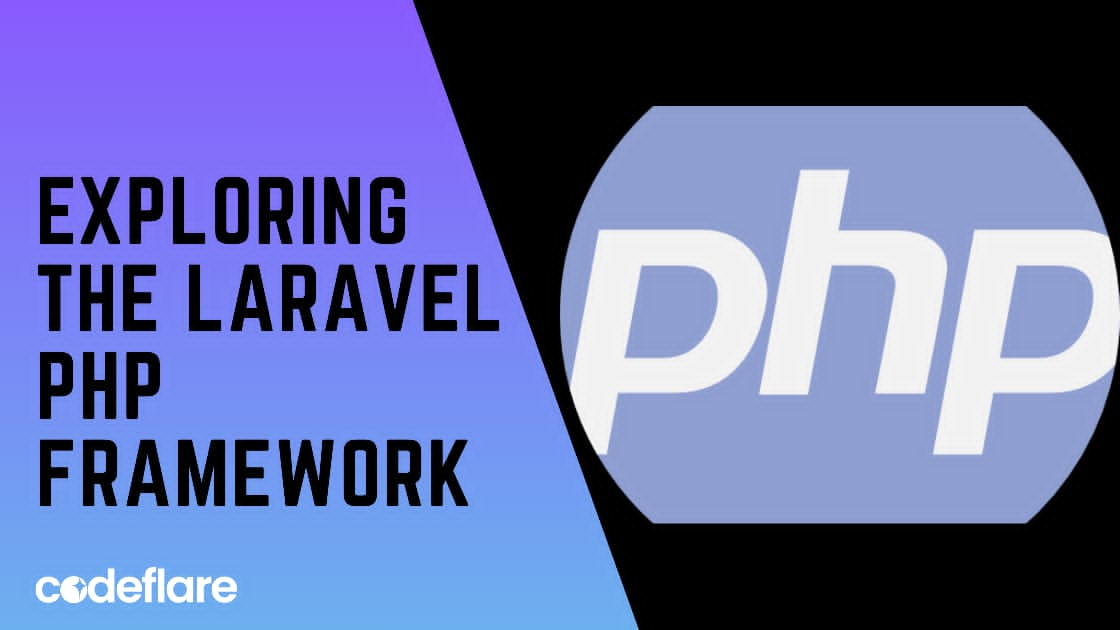
Introduction
Laravel has become one of the most popular PHP frameworks, thanks to its elegant syntax, powerful features, and robust ecosystem. While Laravel is known for its user-friendly approach to common tasks like routing, authentication, and database interactions, it also offers a wealth of advanced features that can significantly improve the efficiency and scalability of your applications. This article will explore some of these advanced techniques and features in Laravel, helping you take your PHP development skills to the next level.
Advanced Routing Techniques
Routing in Laravel is straightforward, but the framework provides advanced routing features that can help you build complex applications more efficiently. Some of these techniques include:
- Route Model BindingRoute model binding allows you to automatically inject models into your routes, simplifying the process of retrieving data from the database.
// Implicit Binding
Route::get('/user/{user}', function (App\Models\User $user) {
return $user->email;
});
// Explicit Binding
Route::get('/post/{post}', function (Post $post) {
return $post->title;
})->where('post', '[0-9]+');
2. Custom Route Macros
Laravel allows you to extend its routing system by defining custom route macros.
use Illuminate\Support\Facades\Route;
Route::macro('admin', function () {
Route::get('dashboard', function () {
// Logic here...
});
});
// Usage
Route::admin();
3. Rate Limiting
Laravel provides built-in rate limiting to prevent abuse and ensure your application scales effectively.
Route::middleware('throttle:10,1')->group(function () {
Route::get('/profile', function () {
// Profile logic...
});
Route::post('/update-profile', function () {
// Update profile logic...
});
});
Middleware: Advanced Use Cases
Middleware is a powerful feature in Laravel that allows you to filter HTTP requests entering your application. Here are some advanced use cases:
1. Dynamic Middleware
You can apply middleware dynamically within your controllers.
public function __construct()
{
$this->middleware(function ($request, $next) {
// Perform action...
return $next($request);
});
}
2. Middleware Parameters
Laravel allows you to pass parameters to middleware.
// Middleware
public function handle($request, Closure $next, $role)
{
if (!$request->user()->hasRole($role)) {
// Redirect if not authorized...
}
return $next($request);
}
// Applying middleware with parameters
Route::get('/admin', function () {
// Admin logic...
})->middleware('role:admin');
3. Grouping Middleware
By grouping middleware, you can apply multiple middleware to a set of routes with a single declaration.
Route::middleware(['auth', 'log:activity'])->group(function () {
Route::get('/dashboard', function () {
// Dashboard logic...
});
Route::get('/settings', function () {
// Settings logic...
});
});
Eloquent ORM: Advanced Query Techniques
Laravel’s Eloquent ORM is a powerful tool for interacting with databases. Here are some advanced query techniques:
1. Eager Loading
Eloquent’s eager loading feature allows you to load related models alongside the main model.
$users = App\Models\User::with('posts', 'comments')->get();
2. Query Scopes
Query scopes allow you to define reusable query logic.
// Local Scope
public function scopeActive($query)
{
return $query->where('active', 1);
}
// Usage
$activeUsers = App\Models\User::active()->get();
3. Mutators and Accessors
Eloquent’s mutators and accessors allow you to modify data before it’s saved to the database or retrieved from it.
// Mutator
public function setPasswordAttribute($value)
{
$this->attributes['password'] = bcrypt($value);
}
// Accessor
public function getNameAttribute($value)
{
return ucfirst($value);
}
Queues and Jobs: Asynchronous Processing
Laravel’s queue system allows you to defer time-consuming tasks to be handled asynchronously in the background:
1. Job Chaining
Laravel allows you to chain jobs together.
$job = new ProcessOrder($order);
$job->chain([
new SendOrderNotification($order),
new UpdateStock($order)
]);
dispatch($job);
2. Job Batching
You can dispatch multiple jobs at once and wait until all jobs in the batch are complete.
Bus::batch([
new ImportCsvJob,
new ProcessCsvJob,
new SendReportJob
])->then(function (Batch $batch) {
// All jobs completed...
})->catch(function (Batch $batch, Throwable $e) {
// Handle failed jobs...
})->dispatch();
3. Failed Job Handling
Laravel provides tools for handling failed jobs, including the ability to automatically retry jobs.
// Handle failed job in the job class
public function failed(Exception $exception)
{
// Send user notification of failure, etc...
}
Testing: Advanced Techniques
Laravel’s testing tools are designed to help you write comprehensive tests for your application:
1. Mocking and Fakes
Laravel provides built-in support for mocking and fakes.
Mail::fake();
$this->post('/send-email', $data);
Mail::assertSent(OrderShipped::class, function ($mail) use ($order) {
return $mail->order->id === $order->id;
});
2. Browser Testing
Laravel Dusk allows you to write and execute tests for your application’s user interface.
$this->browse(function (Browser $browser) {
$browser->visit('/login')
->type('email', '[email protected]')
->type('password', 'secret')
->press('Login')
->assertPathIs('/home');
});
3. Parallel Testing
Laravel’s parallel testing feature allows you to run your tests concurrently.
php artisan test --parallel
Conclusion
Laravel’s advanced features and techniques offer powerful tools for building complex, scalable applications. By mastering these techniques, you can take full advantage of Laravel’s capabilities and develop applications that are both robust and efficient. Whether you’re optimizing your routes, enhancing your database interactions, or implementing asynchronous processing, Laravel provides the tools you need to succeed. As you continue to explore and experiment with these advanced features, you’ll find new ways to improve your applications and deliver even greater value to your users.
Multithreading in PHP: Handling Concurrent Requests Efficiently