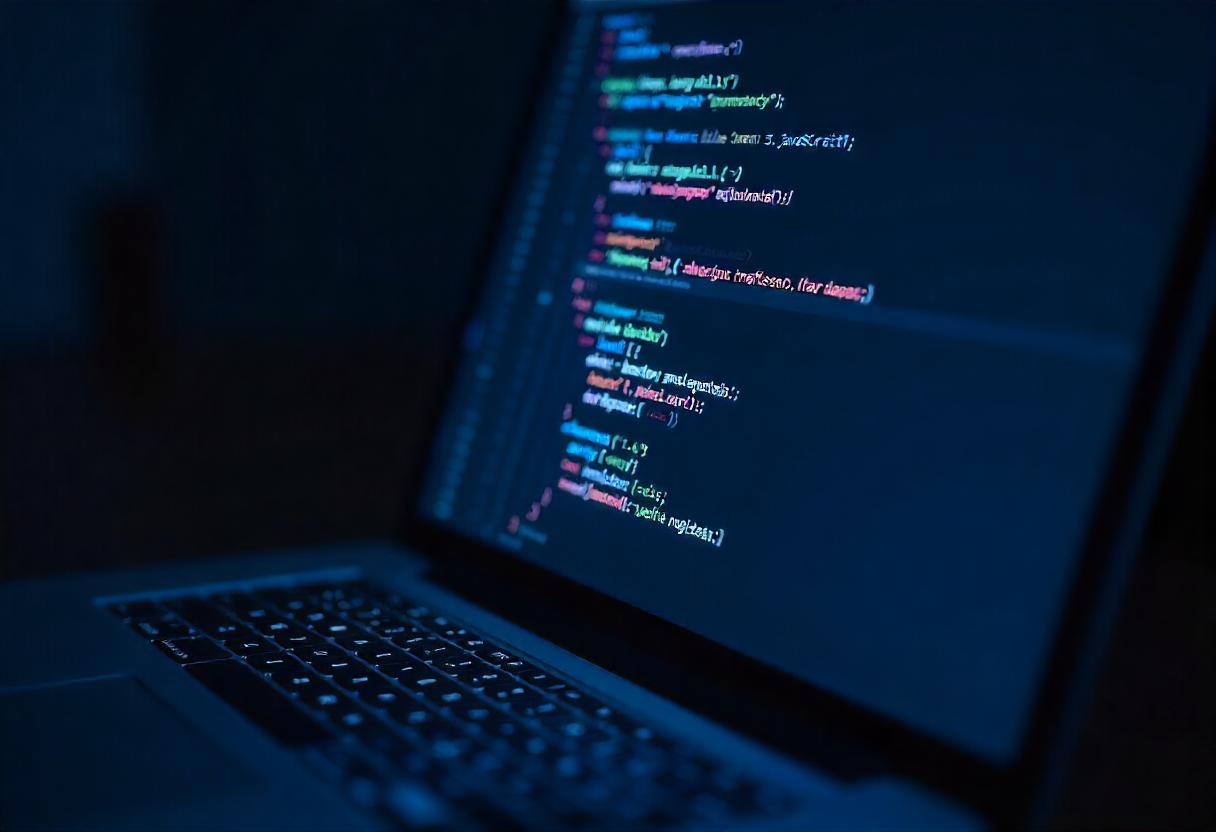
When debugging JavaScript, you’ll often encounter ReferenceError
and TypeError
. While both indicate something went wrong, they mean very different things. Let’s break them down with examples.
See document.querySelector() vs. getElementById(): Which is Faster?
1. ReferenceError
– “Variable Doesn’t Exist”
Occurs when: You try to use a variable or function that hasn’t been declared.
Example Causes
console.log(nonExistentVar); // ReferenceError: nonExistentVar is not defined
someUndefinedFunction(); // ReferenceError: someUndefinedFunction is not defined
How to Fix?
✔ Declare the variable/function before using it.
✔ Check for typos (myVar
vs. myvar
).
2. TypeError
– “Wrong Type or Invalid Operation”
Occurs when: You try to do something invalid with a value, like:
- Calling a non-function.
- Accessing properties of
null
orundefined
.
Example Causes
let foo = null;
foo.bar; // TypeError: Cannot read property 'bar' of null
let num = 42;
num(); // TypeError: num is not a function
How to Fix?
✔ Check if a variable is null
/undefined
before using it.
✔ Ensure functions/methods exist before calling them.
3. Key Differences
Error | Meaning | Common Causes |
---|---|---|
ReferenceError | Variable/function doesn’t exist. | Misspelled names, missing imports. |
TypeError | Operation invalid for the type. | Calling non-functions, accessing null props. |
4. How to Debug?
- For
ReferenceError
: - Check if the variable/function is defined in scope.
- Verify imports (if using modules).
- For
TypeError
: - Use
typeof
or optional chaining (?.
) to avoidnull
/undefined
issues. - Ensure functions are actually callable.
5. Quick Summary
ReferenceError
→ “Does this exist?”TypeError
→ “Can I do this with it?”
Pro Tip: Use TypeScript or ESLint to catch these errors early! 🚀
Which error trips you up more often? Let us know in the comments! 💬