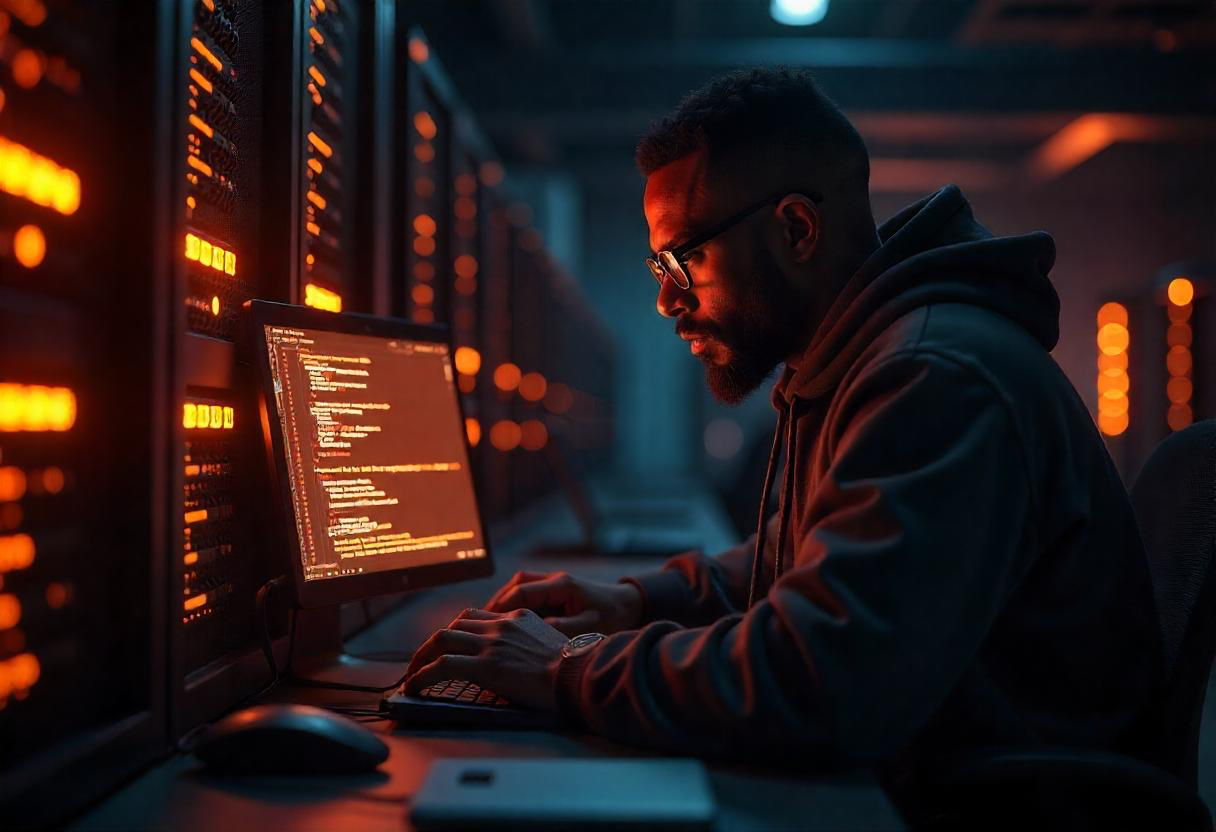
One of the most common errors in JavaScript is the dreaded TypeError: Cannot read property 'x' of undefined
. This happens when you try to access a nested property of an object that doesn’t exist. See Top 5 JavaScript Console Methods to Simplify Debugging.
Before optional chaining (?.
), developers had to write lengthy checks to avoid these errors. Now, with optional chaining, we can write cleaner, safer code.
What is Optional Chaining (?.
)?
Optional chaining (?.
) is a JavaScript feature (introduced in ES2020) that allows you to safely access nested object properties without explicit null checks.
Syntax
obj?.prop // Access property
obj?.[expr] // Access dynamic property
func?.(args) // Call function if it exists
Why Use Optional Chaining?
1. Avoid Lengthy Null Checks
Old Way (Without ?.
)
if (user && user.address && user.address.city) {
console.log(user.address.city);
}
New Way (With ?.
)
console.log(user?.address?.city); // No error if `user` or `address` is null/undefined
2. Safe Function Calls
Prevents errors when calling a method that may not exist.
const result = apiResponse?.getData?.(); // Only calls `getData()` if it exists
3. Safe Array Access
Avoid errors when accessing array indices.
const firstItem = arr?.[0]; // Returns `undefined` if `arr` is null/undefined
Common Use Cases
1. API Responses
APIs often return unpredictable structures. Optional chaining prevents crashes:
const userName = apiResponse?.user?.name || "Guest";
2. Config Objects
Safely access nested config values:
const theme = config?.ui?.theme || "dark";
3. DOM Manipulation
Avoid errors when querying elements that may not exist:
const buttonText = document.querySelector(".btn")?.textContent;
Important Notes
- Does NOT Replace Proper Error Handling – Use when you expect optional values, not for critical missing data.
- Works with
null
andundefined
– Returnsundefined
if any part of the chain isnull
orundefined
. - Not Supported in Very Old Browsers – Use Babel or TypeScript for backward compatibility.
Browser Support
✅ Modern Browsers (Chrome 80+, Firefox 74+, Safari 13.1+)
🚫 No IE11 Support
Conclusion
Optional chaining (?.
) is a game-changer for writing cleaner, safer JavaScript. It reduces boilerplate code and prevents runtime errors when accessing nested properties.
Start using it today to make your code more robust! 🚀
Do you use optional chaining in your projects? Share your experience in the comments! 👇