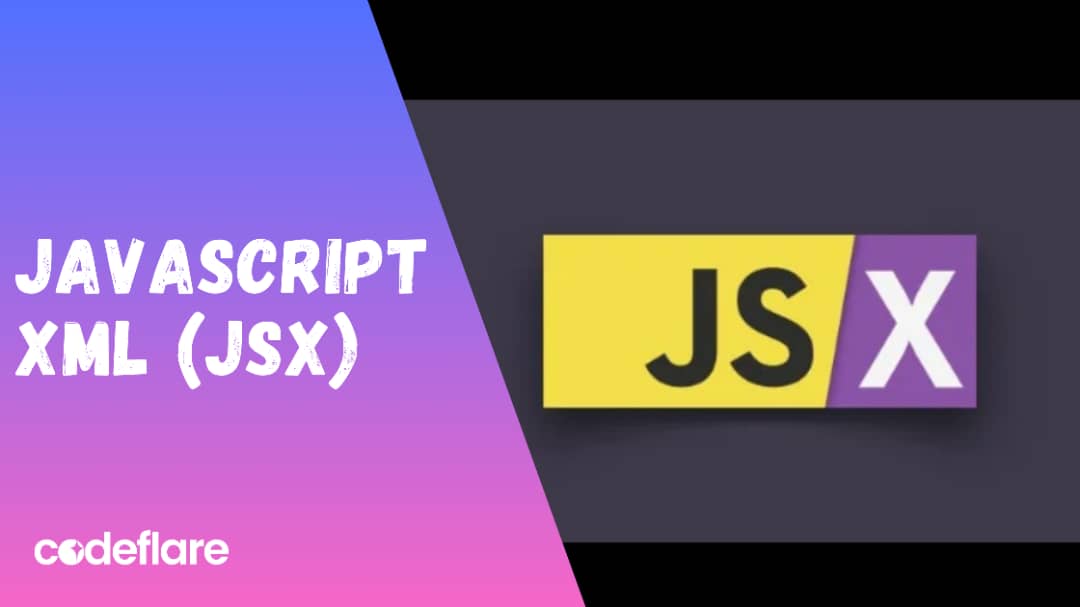
In the world of modern web development, JSX (JavaScript XML) has become a pivotal part of creating user interfaces with React. JSX provides a syntax that combines the best of both JavaScript and HTML, making it easier to write and understand the structure of components. This article delves into what JSX is, why it’s useful, and how to use it effectively in your React projects.
What is JSX?
JSX stands for JavaScript XML. It is a syntax extension for JavaScript, introduced by React, that allows developers to write HTML elements and components in JavaScript code. JSX looks similar to HTML but allows for the full power of JavaScript within it.
For example, a simple JSX snippet might look like this:
const element = <h1>Hello, world!</h1>;
This JSX code creates a React element that renders an <h1>
tag with the text “Hello, world!”.
Why Use JSX?
- Declarative Syntax: JSX provides a more readable and declarative syntax for defining UI components compared to traditional JavaScript. It resembles HTML, making it easier for developers familiar with HTML to work with.
- Embedded Expressions: JSX allows you to embed JavaScript expressions within curly braces
{}
. This makes it possible to dynamically generate content based on variables or function calls.
const name = 'Alice';
const element = <h1>Hello, {name}!</h1>;
3. Component Composition: JSX makes it simple to compose complex UIs by nesting components. Components can be used as tags, and their logic can be encapsulated within them.
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
const element = <Welcome name="Sara" />;
4. Pre-Processing: JSX needs to be transformed into JavaScript code before it can be executed by browsers. This transformation is handled by tools like Babel, which convert JSX into React.createElement calls.
// JSX
const element = <h1>Hello, world!</h1>;
// Transformed JavaScript
const element = React.createElement('h1', null, 'Hello, world!');
Using JSX with React
JSX is primarily used with React, but it’s not a requirement. However, React’s component model and JSX work seamlessly together to define and manage complex user interfaces.
- Creating Components: JSX allows you to define components with ease. Each component can be represented as a function or class that returns JSX.
function MyComponent() {
return <div>This is my component!</div>;
}
2. Conditional Rendering: You can use JavaScript operators like conditional (&&
), ternary (? :
), or functions within JSX to control rendering based on certain conditions.
const isLoggedIn = true;
const element = <div>{isLoggedIn ? 'Welcome!' : 'Please sign in.'}</div>;
3. Lists and Keys: JSX makes it easy to render lists of items using the map
function. Each element in the list should have a unique key
prop to help React manage the list efficiently.
const numbers = [1, 2, 3];
const listItems = numbers.map(number =>
<li key={number.toString()}>{number}</li>
);
Best Practices
- Use Curly Braces for JavaScript Expressions: Always use curly braces
{}
to embed JavaScript expressions within JSX. - Avoid Logic in JSX: Keep complex logic out of JSX. Instead, move it to functions or variables for clarity.
- Use Descriptive Component Names: Name your components descriptively to make your code more readable and maintainable.
- Keep JSX Readable: Format your JSX code to be readable. This includes proper indentation and breaking down complex components into smaller ones.
Conclusion
JSX is a powerful tool that enhances the development experience when working with React. By understanding and leveraging JavaScript XML (JSX) in React, you can write cleaner, more intuitive code, making it easier to build and maintain sophisticated web applications. As you become more familiar with JSX, you’ll appreciate its role in creating dynamic and efficient user interfaces.
Understanding JavaScript filter method