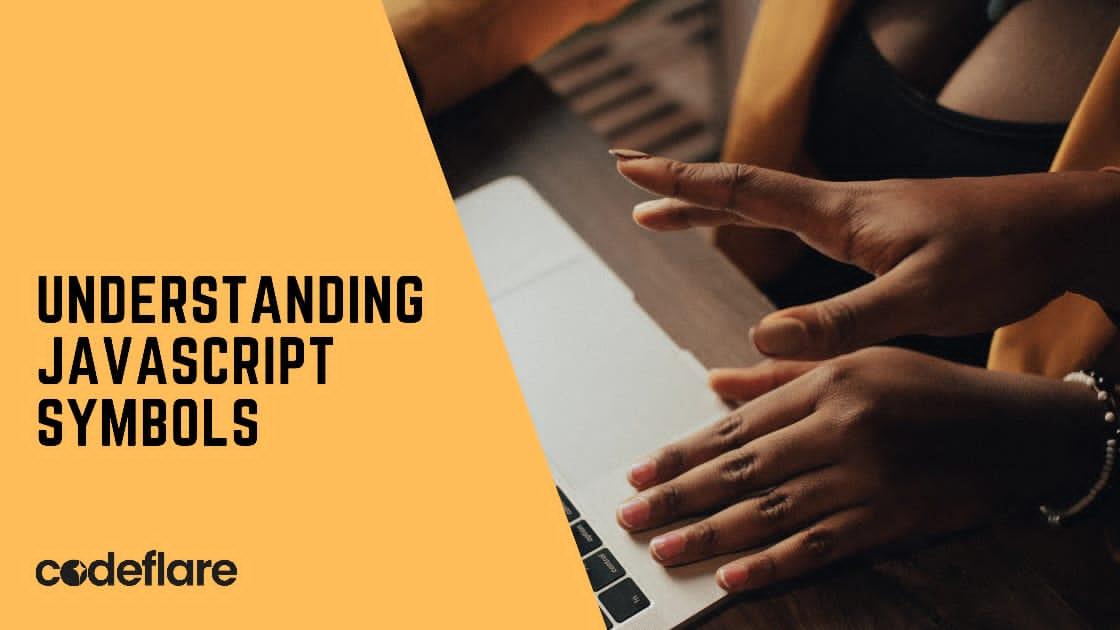
JavaScript, as a programming language, is continuously evolving. With each update, new features are introduced that enhance the language’s capabilities, allowing developers to write more efficient and maintainable code. One such feature that was introduced in ECMAScript 2015 (ES6) is the Symbol data type. Though it may not be as commonly used as strings or numbers, understanding Symbols is essential for leveraging the full potential of JavaScript.
What are Symbols?
A Symbol is a unique and immutable primitive value that is used as the key of an object property. Unlike strings or numbers, every Symbol is unique, even if two Symbols have the same description. This uniqueness is what makes Symbols a powerful tool in JavaScript, especially when it comes to preventing property name collisions in objects.
Creating a Symbol
You can create a Symbol by calling the Symbol()
function:
const sym1 = Symbol();
const sym2 = Symbol();
console.log(sym1 === sym2); // false
In the example above, sym1
and sym2
are two different Symbols, even though they were created in the same way. They are unique and do not equal each other.
You can also pass an optional description (a string) to the Symbol()
function, which is useful for debugging:
const sym3 = Symbol('description');
console.log(sym3); // Symbol(description)
The description is not the identifier of the Symbol but rather a label that helps in debugging.
Why Use Symbols?
Symbols are particularly useful in scenarios where you need to ensure that an object’s property is unique and cannot be accidentally overridden or accessed. Here are some common use cases:
- Avoiding Property Name Collisions: Symbols can be used to create properties on objects without worrying about name conflicts:
const symKey = Symbol('key');
const obj = {
[symKey]: 'value'
};
console.log(obj[symKey]); // 'value'
In this example, the symKey
is unique and ensures that no other property can overwrite it.
2. Implementing Hidden Object Properties: Symbols can be used to create properties that are not easily accessible or enumerable:
const hiddenKey = Symbol('hidden');
const myObject = {
visible: 'I can be seen',
[hiddenKey]: 'You cannot see me'
};
for (let key in myObject) {
console.log(key); // logs only 'visible'
}
console.log(Object.keys(myObject)); // ['visible']
console.log(Object.getOwnPropertyNames(myObject)); // ['visible']
console.log(Object.getOwnPropertySymbols(myObject)); // [Symbol(hidden)]
The hiddenKey
Symbol is not included in loops or methods that return the object’s keys, making it effectively hidden from the usual object introspection methods.
3. Defining Constants: Since Symbols are unique and immutable, they make excellent constants:
const COLOR_RED = Symbol('red');
const COLOR_GREEN = Symbol('green');
function getColorName(color) {
switch(color) {
case COLOR_RED:
return 'Red';
case COLOR_GREEN:
return 'Green';
default:
return 'Unknown color';
}
}
4. Using Well-Known Symbols: JavaScript has several built-in, well-known Symbols that change the behavior of objects and functions. For example:
Symbol.iterator
: Allows an object to be iterable.Symbol.toStringTag
: Customizes the default string description of an object.
const myArray = [1, 2, 3];
const iterator = myArray[Symbol.iterator]();
console.log(iterator.next().value); // 1
const customObj = {
[Symbol.toStringTag]: 'CustomObject'
};
console.log(customObj.toString()); // [object CustomObject]
Symbols in Practice
Symbols might seem abstract at first, but they provide a level of abstraction and safety that is invaluable in complex applications, libraries, or frameworks. When you’re working on a project that involves multiple teams or third-party integrations, Symbols can help ensure that your object properties remain distinct and free from naming conflicts.
Conclusion
Understanding and using Symbols can elevate your JavaScript code by providing unique, non-colliding property keys and offering mechanisms for implementing hidden or constant properties. While they might not be needed in every project, when the need arises, Symbols can be an incredibly powerful tool in your JavaScript toolkit.
Working with Immutable Data structures in JavaScript