Interactive maps are an excellent way to present geographic data and enhance user experience on websites. They allow users to engage with the map by zooming, panning, and clicking on various elements. In this article, we will guide you through the process of creating interactive maps using JavaScript, focusing on two popular libraries: Leaflet and Mapbox. By the end of this JavaScript interactive maps tutorial, you’ll have a functional interactive map with a search feature that allows users to locate different countries.
Step 1: Setting Up the HTML Structure
First, we need to create the basic HTML structure for our project. This will include containers for our Leaflet and Mapbox maps, as well as a search input and button.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Maps with JavaScript</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet/dist/leaflet.css" />
<link href='https://api.mapbox.com/mapbox-gl-js/v2.6.1/mapbox-gl.css' rel='stylesheet' />
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f4f4f4;
margin: 0;
padding: 0;
}
h1 {
margin-top: 20px;
}
#map, #mapbox {
height: 500px;
width: 80%;
margin: 20px auto;
border: 2px solid #333;
}
.search-container {
margin: 20px auto;
width: 80%;
display: flex;
justify-content: center;
align-items: center;
}
.search-container input {
width: 300px;
padding: 10px;
border: 1px solid #333;
border-radius: 4px;
margin-right: 10px;
}
.search-container button {
padding: 10px 20px;
border: none;
background-color: #333;
color: #fff;
border-radius: 4px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Interactive Maps with JavaScript</h1>
<div class="search-container">
<input type="text" id="search-input" placeholder="Enter a country name...">
<button onclick="searchCountry()">Search</button>
</div>
<div id="map"></div>
<div id="mapbox"></div>
<script src="https://unpkg.com/leaflet/dist/leaflet.js"></script>
<script src='https://api.mapbox.com/mapbox-gl-js/v2.6.1/mapbox-gl.js'></script>
</body>
</html>
Step 2: Initializing Leaflet and Mapbox Maps
Next, we will initialize both Leaflet and Mapbox maps in the JavaScript section of our HTML file.
<script>
// Initialize Leaflet map
const leafletMap = L.map('map').setView([51.505, -0.09], 3);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
}).addTo(leafletMap);
let leafletMarker;
let leafletCircle;
// Initialize Mapbox map
mapboxgl.accessToken = 'your.mapbox.access.token'; // Replace with your Mapbox access token
const mapboxMap = new mapboxgl.Map({
container: 'mapbox',
style: 'mapbox://styles/mapbox/streets-v11',
center: [-74.5, 40],
zoom: 3
});
let mapboxMarker;
</script>
Step 3: Adding Search Functionality
We will now add a search functionality that allows users to input a country name and locate it on the map. For this, we will use the OpenCage Geocoding API.
<script>
function searchCountry() {
const country = document.getElementById('search-input').value;
const apiKey = 'your-opencage-api-key'; // Replace with your OpenCage API key
const url = `https://api.opencagedata.com/geocode/v1/json?q=${country}&key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
if (data.results.length > 0) {
const { lat, lng } = data.results[0].geometry;
// Update Leaflet map
if (leafletMarker) {
leafletMap.removeLayer(leafletMarker);
leafletMap.removeLayer(leafletCircle);
}
leafletMap.setView([lat, lng], 6);
leafletMarker = L.marker([lat, lng]).addTo(leafletMap);
leafletCircle = L.circle([lat, lng], {
color: 'red',
fillColor: '#f03',
fillOpacity: 0.5,
radius: 50000
}).addTo(leafletMap);
// Update Mapbox map
if (mapboxMarker) {
mapboxMarker.remove();
}
mapboxMap.flyTo({ center: [lng, lat], zoom: 6 });
mapboxMarker = new mapboxgl.Marker()
.setLngLat([lng, lat])
.addTo(mapboxMap);
} else {
alert('Country not found!');
}
})
.catch(error => console.error('Error:', error));
}
</script>
Step 4: Combining Everything Together
Here’s the complete HTML file with all the steps combined:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Interactive Maps with JavaScript</title>
<link rel="stylesheet" href="https://unpkg.com/leaflet/dist/leaflet.css" />
<link href='https://api.mapbox.com/mapbox-gl-js/v2.6.1/mapbox-gl.css' rel='stylesheet' />
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f4f4f4;
margin: 0;
padding: 0;
}
h1 {
margin-top: 20px;
}
#map, #mapbox {
height: 500px;
width: 80%;
margin: 20px auto;
border: 2px solid #333;
}
.search-container {
margin: 20px auto;
width: 80%;
display: flex;
justify-content: center;
align-items: center;
}
.search-container input {
width: 300px;
padding: 10px;
border: 1px solid #333;
border-radius: 4px;
margin-right: 10px;
}
.search-container button {
padding: 10px 20px;
border: none;
background-color: #333;
color: #fff;
border-radius: 4px;
cursor: pointer;
}
</style>
</head>
<body>
<h1>Interactive Maps with JavaScript</h1>
<div class="search-container">
<input type="text" id="search-input" placeholder="Enter a country name...">
<button onclick="searchCountry()">Search</button>
</div>
<div id="map"></div>
<div id="mapbox"></div>
<script src="https://unpkg.com/leaflet/dist/leaflet.js"></script>
<script src='https://api.mapbox.com/mapbox-gl-js/v2.6.1/mapbox-gl.js'></script>
<script>
// Initialize Leaflet map
const leafletMap = L.map('map').setView([51.505, -0.09], 3);
L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', {
attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
}).addTo(leafletMap);
let leafletMarker;
let leafletCircle;
// Initialize Mapbox map
mapboxgl.accessToken = 'your.mapbox.access.token'; // Replace with your Mapbox access token
const mapboxMap = new mapboxgl.Map({
container: 'mapbox',
style: 'mapbox://styles/mapbox/streets-v11',
center: [-74.5, 40],
zoom: 3
});
let mapboxMarker;
function searchCountry() {
const country = document.getElementById('search-input').value;
const apiKey = 'your-opencage-api-key'; // Replace with your OpenCage API key
const url = `https://api.opencagedata.com/geocode/v1/json?q=${country}&key=${apiKey}`;
fetch(url)
.then(response => response.json())
.then(data => {
if (data.results.length > 0) {
const { lat, lng } = data.results[0].geometry;
// Update Leaflet map
if (leafletMarker) {
leafletMap.removeLayer(leafletMarker);
leafletMap.removeLayer(leafletCircle);
}
leafletMap.setView([lat, lng], 6);
leafletMarker = L.marker([lat, lng]).addTo(leafletMap);
leafletCircle = L.circle([lat, lng], {
color: 'red',
fillColor: '#f03',
fillOpacity: 0.5,
radius: 50000
}).addTo(leafletMap);
// Update Mapbox map
if (mapboxMarker) {
mapboxMarker.remove();
}
mapboxMap.flyTo({ center: [lng, lat], zoom: 6 });
mapboxMarker = new mapboxgl.Marker()
.setLngLat([lng, lat])
.addTo(mapboxMap);
} else {
alert('Country not found!');
}
})
.catch(error => console.error('Error:', error));
}
</script>
</body>
</html>
Here’s the outcome!
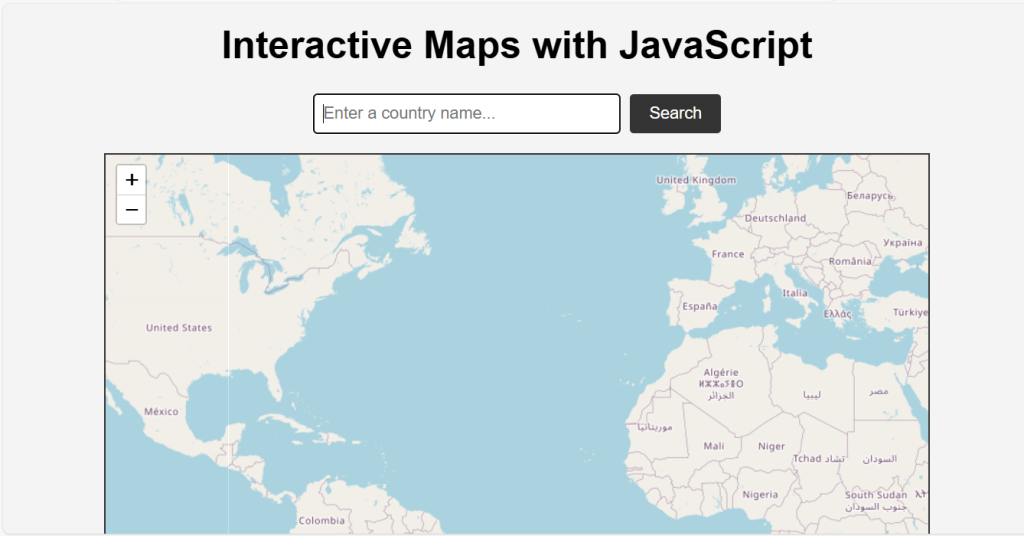
Important Notes:
- Search Input and Button: Added an input field and a button for users to enter a country name and initiate a search.
- JavaScript Search Functionality:
searchCountry
function fetches data from the OpenCage Geocoding API using the country name provided by the user. It then updates both the Leaflet and Mapbox maps to center on the searched country’s coordinates. - Leaflet Map Update: Adds a marker and circle to the Leaflet map at the searched country’s coordinates.
- Mapbox Map Update: Adds a marker to the Mapbox map and centers it on the searched country’s coordinates.
- Styling: You can further customize the styling as per your requirements.
- Error Handling: Additional error handling can be added to improve user experience in case of network errors or invalid inputs.
Replace 'your.mapbox.access.token'
and 'your-opencage-api-key'
with your actual Mapbox access token and OpenCage API key, respectively.
Conclusion
Creating interactive maps using JavaScript libraries like Leaflet and Mapbox is a powerful way to enhance the user experience on your website. By following the steps outlined in this article, you can create a basic interactive map and expand upon it with various features and customizations. Whether you need a simple map to display a location or a complex one with multiple layers and interactions, these libraries provide the tools you need to build engaging and interactive maps. This JavaScript tutorial covers everything from setting up your map and adding markers to incorporating search functionality and customizing map styles.
Further Resources
By exploring these resources, you can continue to enhance your maps and learn more about the advanced capabilities of these powerful JavaScript libraries.
How to create Tabs and accordions using JavaScript