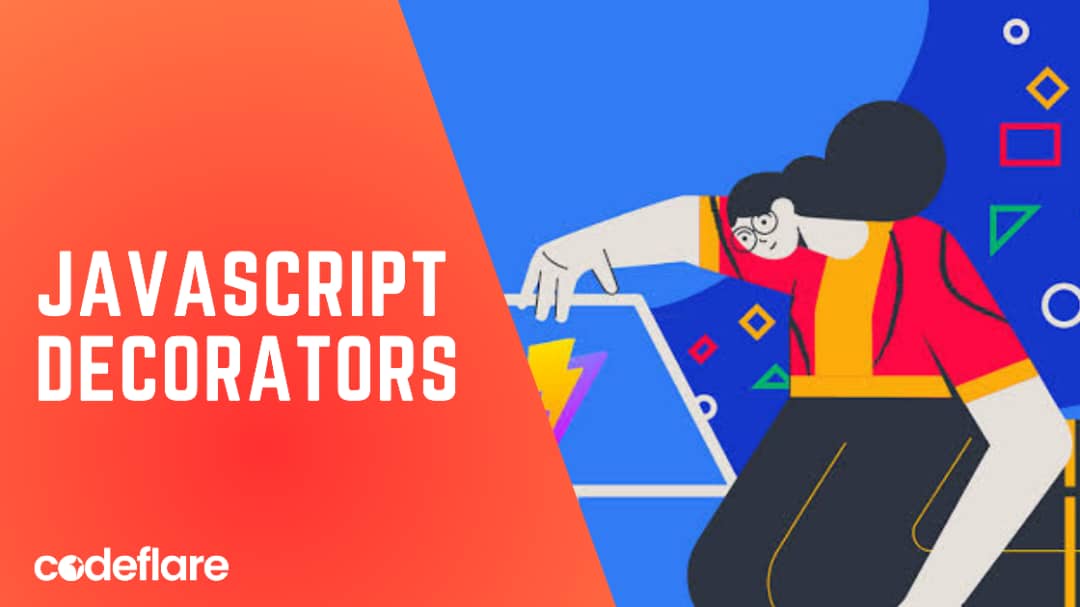
In modern JavaScript development, decorators have emerged as a powerful pattern for extending the functionality of objects and classes in a clean and maintainable way. While decorators are not yet a native part of JavaScript, they are widely used in frameworks like Angular and libraries like TypeScript to enhance the capabilities of functions, methods, and entire classes. This article will explore the concept of JavaScript decorators, how they work, and how you can use them to add functionality to objects in your applications.
What Are JavaScript Decorators?
A decorator is a function that takes an object or a class as an argument and returns a new object or class with added behavior. In essence, decorators wrap or augment existing objects, allowing you to add new methods, modify existing ones, or even change how an object or method behaves altogether.
For example, you can use a decorator to add logging functionality to a method, so every time the method runs, it creates a log entry. Another common use involves enforcing access control by adding checks before executing a method.
How Decorators Work
Although JavaScript does not natively support decorators as of now, you can implement them using higher-order functions or by leveraging libraries like TypeScript. In a typical decorator pattern:
- Create the Decorator Function: This function takes the target object (such as a class or method) and optionally additional arguments.
- Modify or Extend the Target: Inside the decorator function, you can modify the target’s behavior, add properties or methods, or wrap it with additional functionality.
- Return the New Object: The decorator returns a new object or class that includes the modified or extended behavior.
Here’s a simple example:
function logger(target, key, descriptor) {
const originalMethod = descriptor.value;
descriptor.value = function(...args) {
console.log(`Calling ${key} with`, args);
return originalMethod.apply(this, args);
};
return descriptor;
}
class Example {
@logger
sayHello(name) {
return `Hello, ${name}!`;
}
}
const example = new Example();
example.sayHello('World'); // Logs: Calling sayHello with ['World']
In this example, the logger
decorator adds logging to the sayHello
method, so every time the method is called, the arguments are logged to the console.
Use Cases for Decorators
Decorators offer versatility, serving a variety of scenarios effectively.
- Logging: Automatically log method calls, arguments, and return values.
- Memoization: Cache the results of expensive function calls to improve performance.
- Validation: Ensure that method inputs meet certain criteria before execution.
- Access Control: Restrict access to methods based on user roles or permissions.
- Performance Monitoring: Track how long methods take to execute and identify bottlenecks.
Conclusion
JavaScript decorators provide a powerful and flexible approach to enhancing objects and classes with additional functionality. Although they aren’t officially part of the JavaScript standard yet, developers frequently use them in modern development through various frameworks and libraries. By mastering decorators, you can write cleaner, more modular, and maintainable code, making your applications more robust and easier to extend.
JavaScript Proxies: Enhancing Object Behavior