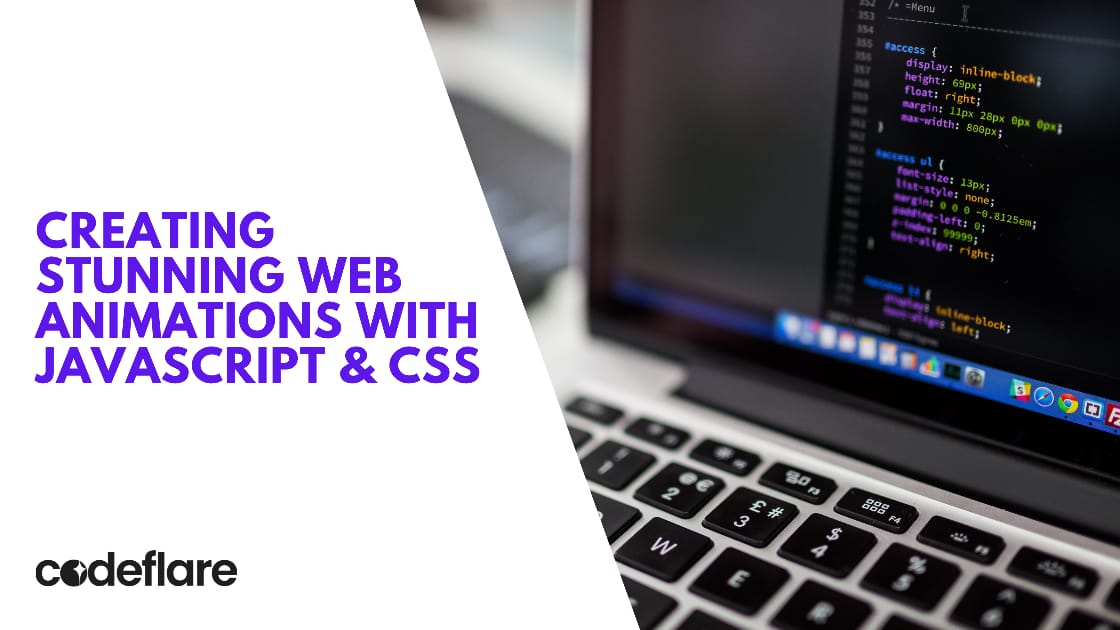
Web animations bring websites to life, adding flair and interactivity that can captivate users. By leveraging JavaScript and CSS, you can create stunning web animations that are both visually appealing and functional. This article will guide you through the basics of crafting impressive web animations with JavaScript and CSS, enhancing user experience and making your site more engaging.
Why Use Web Animations?
Animations can:
- Draw Attention: Highlight important elements and guide users through your content.
- Enhance Usability: Provide feedback and make interactions more intuitive.
- Create Aesthetic Appeal: Add visual interest and improve the overall look of your site.
Getting Started with CSS Animations
CSS animations allow you to animate properties like color, size, and position with smooth transitions. Here’s a simple example to get you started:
/* Define the keyframes for the animation */
@keyframes fadeIn {
from { opacity: 0; }
to { opacity: 1; }
}
/* Apply the animation to an element */
.animated-element {
animation: fadeIn 2s ease-in-out;
}
In this example, an element with the class .animated-element
will gradually fade in over 2 seconds.
Adding Interactivity with JavaScript
JavaScript provides more control over animations, allowing for complex interactions and dynamic effects. You can use JavaScript to start, stop, or adjust animations based on user actions.
Here’s an example of using JavaScript to animate an element:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>JavaScript Animation</title>
<style>
.box {
width: 100px;
height: 100px;
background-color: blue;
position: relative;
}
</style>
</head>
<body>
<div class="box"></div>
<button id="animateBtn">Animate</button>
<script>
document.getElementById('animateBtn').addEventListener('click', function() {
let box = document.querySelector('.box');
box.style.transition = 'transform 2s ease-in-out';
box.style.transform = 'translateX(200px)';
});
</script>
</body>
</html>
In this example, clicking the “Animate” button will move the .box
element 200 pixels to the right over 2 seconds.
Combining JavaScript and CSS Animations
You can combine CSS and JavaScript to create more sophisticated animations. Use CSS for basic animations and JavaScript to add interactivity and control.
For example, you can trigger a CSS animation with JavaScript like this:
let element = document.querySelector('.animated-element');
element.classList.add('start-animation');
And in your CSS:
@keyframes slideIn {
from { transform: translateX(-100%); }
to { transform: translateX(0); }
}
.start-animation {
animation: slideIn 1s forwards;
}
Here, adding the class .start-animation
will trigger the slideIn
animation.
Best Practices for Web Animations
- Keep It Simple: Avoid overly complex animations that might impact performance.
- Optimize Performance: Use efficient animations and test on various devices.
- Enhance Usability: Ensure animations improve user experience and don’t distract or confuse users.
Conclusion
Creating beautiful web animations with JavaScript and CSS can significantly enhance the user experience on your website. By mastering these techniques, you can add interactive and visually appealing elements that captivate and engage your audience. Experiment with different styles and effects to find what works best for your site.