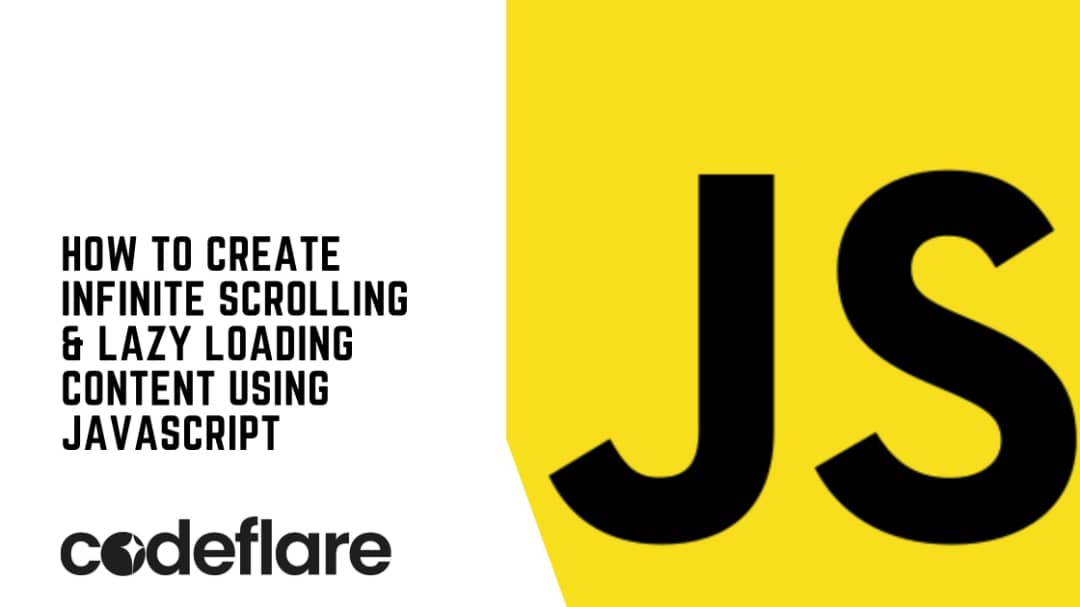
Infinite scrolling and lazy loading are two powerful techniques that can significantly improve the user experience on websites by loading content dynamically as users scroll. This approach not only enhances performance by loading content only when needed but also provides a seamless browsing experience. In this article, we’ll guide you through the steps to implement infinite scrolling and lazy loading using JavaScript, focusing on the JavaScript Infinite Scrolling and Lazy Loading Tutorial.
1. Introduction
Infinite scrolling automatically loads more content as the user scrolls down the page, eliminating the need for pagination. Lazy loading, on the other hand, delays the loading of images or other content until they are about to enter the viewport. Together, these techniques can create a smooth and efficient user experience.
2. Setting Up the HTML Structure
First, we’ll create the basic HTML structure for our content. We’ll use a container to hold the items that will be loaded dynamically.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Infinite Scrolling and Lazy Loading</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="content-container" id="content-container">
<!-- Content will be dynamically loaded here -->
</div>
<div id="loader" class="loader">Loading...</div>
<script src="script.js"></script>
</body>
</html>
3. Styling with CSS
Next, we’ll add some basic CSS to style the content container and the loader.
Best IT Training Institute in Abuja
/* styles.css */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
flex-direction: column;
align-items: center;
}
.content-container {
width: 80%;
max-width: 800px;
margin-top: 20px;
}
.content-item {
background-color: #f4f4f4;
margin-bottom: 20px;
padding: 20px;
border-radius: 8px;
}
.loader {
display: none;
margin: 20px 0;
}
4. Implementing Infinite Scrolling with JavaScript
Now, let’s implement the infinite scrolling functionality. We’ll add an event listener to the window to detect when the user is close to the bottom of the page and load more content.
// script.js
document.addEventListener('DOMContentLoaded', () => {
const contentContainer = document.getElementById('content-container');
const loader = document.getElementById('loader');
let page = 1;
const loadMoreContent = () => {
loader.style.display = 'block';
fetch(`https://jsonplaceholder.typicode.com/posts?_page=${page}&_limit=5`)
.then(response => response.json())
.then(data => {
data.forEach(item => {
const contentItem = document.createElement('div');
contentItem.classList.add('content-item');
contentItem.innerHTML = `
<h2>${item.title}</h2>
<p>${item.body}</p>
`;
contentContainer.appendChild(contentItem);
});
loader.style.display = 'none';
page++;
})
.catch(error => console.error('Error fetching data:', error));
};
const handleScroll = () => {
const { scrollTop, scrollHeight, clientHeight } = document.documentElement;
if (scrollTop + clientHeight >= scrollHeight - 5) {
loadMoreContent();
}
};
window.addEventListener('scroll', handleScroll);
// Initial content load
loadMoreContent();
});
5. Adding Lazy Loading for Images
To enhance performance further, we can implement lazy loading for images. We’ll modify our HTML and JavaScript to include image elements and load them only when they are about to enter the viewport.
HTML Modification
<div class="content-item">
<h2>${item.title}</h2>
<img class="lazy-load" data-src="path/to/image.jpg" alt="Image description">
<p>${item.body}</p>
</div>
JavaScript for Lazy Loading
// script.js
document.addEventListener('DOMContentLoaded', () => {
const contentContainer = document.getElementById('content-container');
const loader = document.getElementById('loader');
let page = 1;
const loadMoreContent = () => {
loader.style.display = 'block';
fetch(`https://jsonplaceholder.typicode.com/posts?_page=${page}&_limit=5`)
.then(response => response.json())
.then(data => {
data.forEach(item => {
const contentItem = document.createElement('div');
contentItem.classList.add('content-item');
contentItem.innerHTML = `
<h2>${item.title}</h2>
<img class="lazy-load" data-src="path/to/image.jpg" alt="Image description">
<p>${item.body}</p>
`;
contentContainer.appendChild(contentItem);
});
loader.style.display = 'none';
lazyLoadImages();
page++;
})
.catch(error => console.error('Error fetching data:', error));
};
const lazyLoadImages = () => {
const lazyImages = document.querySelectorAll('.lazy-load');
const config = {
rootMargin: '0px 0px 50px 0px',
threshold: 0.01
};
let observer = new IntersectionObserver((entries, self) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src;
img.classList.remove('lazy-load');
self.unobserve(img);
}
});
}, config);
lazyImages.forEach(image => observer.observe(image));
};
const handleScroll = () => {
const { scrollTop, scrollHeight, clientHeight } = document.documentElement;
if (scrollTop + clientHeight >= scrollHeight - 5) {
loadMoreContent();
}
};
window.addEventListener('scroll', handleScroll);
// Initial content load
loadMoreContent();
});
Below is a combined HTML file that incorporates infinite scrolling and lazy loading for images using JavaScript. This file includes all the necessary HTML, CSS, and JavaScript in one place.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Infinite Scrolling and Lazy Loading</title>
<style>
/* CSS styles for the page */
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
display: flex;
flex-direction: column;
align-items: center;
}
.content-container {
width: 80%;
max-width: 800px;
margin-top: 20px;
}
.content-item {
background-color: #f4f4f4;
margin-bottom: 20px;
padding: 20px;
border-radius: 8px;
}
.content-item img {
width: 100%;
height: auto;
display: block;
margin: 10px 0;
}
.loader {
display: none;
margin: 20px 0;
}
</style>
</head>
<body>
<!-- Container for dynamic content -->
<div class="content-container" id="content-container">
<!-- Content will be dynamically loaded here -->
</div>
<!-- Loader shown while fetching new content -->
<div id="loader" class="loader">Loading...</div>
<script>
document.addEventListener('DOMContentLoaded', () => {
const contentContainer = document.getElementById('content-container');
const loader = document.getElementById('loader');
let page = 1;
const loadMoreContent = () => {
loader.style.display = 'block';
fetch(`https://jsonplaceholder.typicode.com/posts?_page=${page}&_limit=5`)
.then(response => response.json())
.then(data => {
data.forEach(item => {
const contentItem = document.createElement('div');
contentItem.classList.add('content-item');
// This is the div with the class content-item
contentItem.innerHTML = `
<div class="content-item">
<h2>${item.title}</h2>
<img class="lazy-load" data-src="https://via.placeholder.com/600x400?text=Image+${item.id}" alt="Image description">
<p>${item.body}</p>
</div>
`;
contentContainer.appendChild(contentItem);
});
loader.style.display = 'none';
lazyLoadImages();
page++;
})
.catch(error => console.error('Error fetching data:', error));
};
const lazyLoadImages = () => {
const lazyImages = document.querySelectorAll('.lazy-load');
const config = {
rootMargin: '0px 0px 50px 0px',
threshold: 0.01
};
let observer = new IntersectionObserver((entries, self) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
const img = entry.target;
img.src = img.dataset.src;
img.classList.remove('lazy-load');
self.unobserve(img);
}
});
}, config);
lazyImages.forEach(image => observer.observe(image));
};
const handleScroll = () => {
const { scrollTop, scrollHeight, clientHeight } = document.documentElement;
if (scrollTop + clientHeight >= scrollHeight - 5) {
loadMoreContent();
}
};
window.addEventListener('scroll', handleScroll);
// Initial content load
loadMoreContent();
});
</script>
</body>
</html>
Detailed Explanation:
- HTML Structure:
- The
content-container
div will hold all dynamically loaded content. - The
loader
div is shown when new content is being fetched.
- The
- CSS:
- Basic styling for the body, container, content items, images and loader.
- JavaScript:
- When the page is loaded, the script initializes variables for the container and loader, and sets the initial page number to 1.
loadMoreContent
function:- Shows the loader.
- Fetches data from the JSONPlaceholder API.
- Creates a new
contentItem
div for each post. - Adds an
img
tag with adata-src
attribute for lazy loading. - Appends the new content item to the container.
- Hides the loader.
- Calls
lazyLoadImages
to set up lazy loading for the new images. - Increments the page number for the next API call.
lazyLoadImages
function:- Uses the Intersection Observer API to observe images with the
lazy-load
class. - Sets the actual
src
attribute when the image enters the viewport, removing thelazy-load
class.
- Uses the Intersection Observer API to observe images with the
handleScroll
function:- Checks if the user has scrolled near the bottom of the page.
- Calls
loadMoreContent
to load more content if near the bottom.
- Adds an event listener for the scroll event to call
handleScroll
.
6. Conclusion
Creating infinite scrolling and lazy loading content using JavaScript can significantly enhance the performance and user experience of your website. By following the steps outlined in this article, you can implement these features effectively. Remember to replace the API URLs and other placeholders with your actual data sources to ensure seamless integration of infinite scrolling and lazy loading on your site. This tutorial focuses on JavaScript infinite scrolling and lazy loading implementation, providing a comprehensive guide for optimizing content delivery.
The challenges in todays crypto world