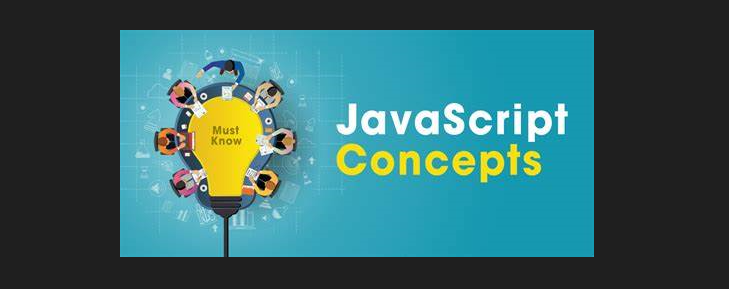
JavaScript is a versatile and powerful programming language that is essential for web development. Whether you are a beginner or an experienced developer, understanding key JavaScript concepts is crucial for building efficient and effective applications. Here are seven fundamental JavaScript concepts every developer should know:
1. Closures
Closures are a fundamental concept in JavaScript that allows functions to access variables from an enclosing scope, even after that scope has exited. This is particularly useful for creating private variables and functions.
Example:
function outerFunction(outerVariable) {
return function innerFunction(innerVariable) {
console.log('Outer Variable: ' + outerVariable);
console.log('Inner Variable: ' + innerVariable);
};
}
const newFunction = outerFunction('outside');
newFunction('inside');
2. Asynchronous Programming (Promises and Async/Await)
JavaScript is single-threaded, meaning it can only do one thing at a time. Asynchronous programming allows JavaScript to perform tasks without blocking the main thread. Promises and async/await
are modern ways to handle asynchronous operations.
Example with Promises:
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched');
}, 2000);
});
}
fetchData().then(data => console.log(data));
Example with Async/Await:
async function fetchData() {
const data = await new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Data fetched');
}, 2000);
});
console.log(data);
}
fetchData();
3. Prototypal Inheritance
JavaScript uses prototypal inheritance, where objects inherit properties and methods from other objects. Understanding how prototypal inheritance works is essential for mastering JavaScript’s object-oriented programming.
Example:
function Person(name) {
this.name = name;
}
Person.prototype.sayHello = function() {
console.log('Hello, my name is ' + this.name);
};
const alice = new Person('Alice');
alice.sayHello();
4. The Event Loop
The event loop is a crucial concept for understanding how JavaScript handles asynchronous operations. It allows JavaScript to perform non-blocking operations by offloading tasks to the browser or Node.js API, which then calls a callback when the operation is complete.
Example:
console.log('Start');
setTimeout(() => {
console.log('Timeout');
}, 0);
console.log('End');
5. Higher-Order Functions
Higher-order functions are functions that operate on other functions, either by taking them as arguments or by returning them. This concept is vital for functional programming in JavaScript.
Example:
function greet(name) {
return function(message) {
console.log(message + ', ' + name);
};
}
const greetAlice = greet('Alice');
greetAlice('Hello');
6. Currying
Currying is a technique in functional programming where a function is transformed into a series of functions, each with a single argument. This helps in creating more modular and reusable code.
Example:
function multiply(a) {
return function(b) {
return a * b;
};
}
const multiplyByTwo = multiply(2);
console.log(multiplyByTwo(3)); // Output: 6
7. Modules
Modules allow developers to break down their code into smaller, reusable pieces. Using modules helps in organizing and managing code, especially in larger applications.
Example with ES6 Modules:
math.js
export function add(a, b) {
return a + b;
}
export function subtract(a, b) {
return a - b;
}
main.js
:
import { add, subtract } from './math.js';
console.log(add(5, 3)); // Output: 8
console.log(subtract(5, 3)); // Output: 2
Conclusion
Understanding these seven JavaScript concepts is essential for any developer looking to master the language. Closures, asynchronous programming, prototypal inheritance, the event loop, higher-order functions, currying, and modules form the foundation of modern JavaScript development. By mastering these concepts, developers can write more efficient, readable, and maintainable code.
How to conduct a website audit for SEO