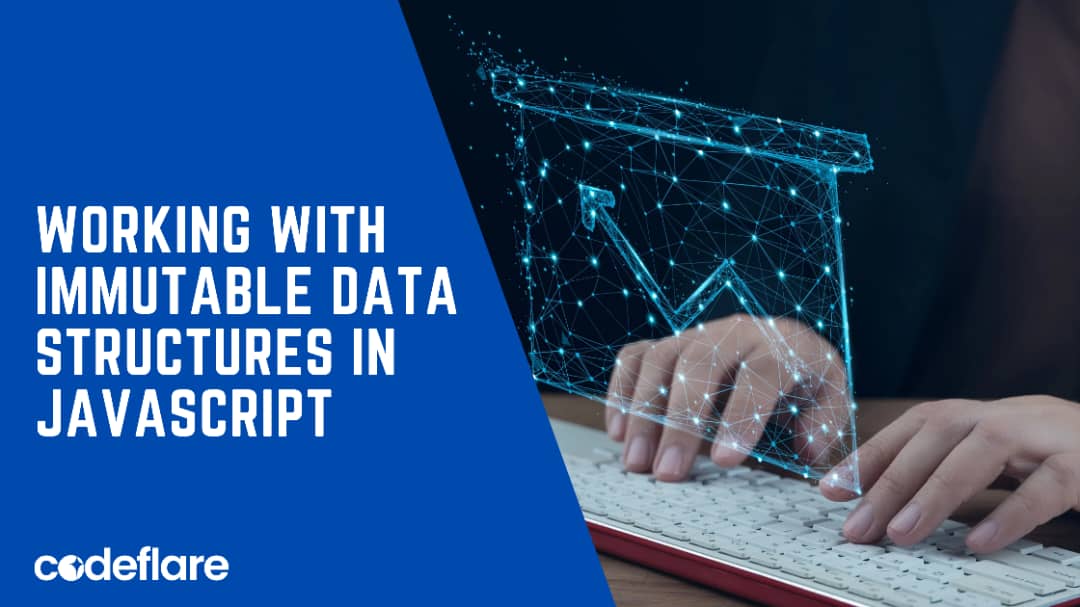
In modern JavaScript development, immutability has become a key concept, especially with the rise of functional programming and state management libraries like Redux. Immutable data structures are those that, once created, cannot be changed. Instead of modifying the original data structure, any operation that would alter the data returns a new structure, leaving the original untouched. This approach offers several benefits, including easier debugging, better performance in some cases, and a reduction in side effects, which can lead to more predictable and maintainable code.
What Are Immutable Data Structures?
Immutable data structures are objects or data types that, once created, do not allow their content to be altered. Instead, operations that would modify the data result in the creation of a new structure with the updated content. In JavaScript, common examples of immutable data include strings and numbers, but when working with more complex data types like arrays and objects, immutability must be explicitly managed.
Why Use Immutable Data Structures?
- Predictability and Debugging: Since immutable data cannot change, you can be sure that its state remains consistent throughout the program. This predictability makes it easier to debug and understand the flow of your application.
- Concurrency: Immutable data structures make it easier to manage concurrent operations. Since data doesn’t change, multiple processes or threads can read from the same data without the risk of race conditions.
- History/Undo Functionality: Many applications, especially those with user interfaces, require the ability to undo or track changes over time. Immutable data structures naturally support this by maintaining a history of states.
- Performance Optimizations: While it might seem that creating new data structures all the time would be inefficient, many immutable libraries, such as Immutable.js, use structural sharing techniques to minimize the memory and processing overhead.
Implementing Immutability in JavaScript
JavaScript doesn’t natively enforce immutability for arrays and objects, but there are several techniques and libraries that can help you implement it:
- Object.freeze(): This method allows you to shallowly freeze an object, preventing any changes to its properties.
const obj = Object.freeze({ name: "John" });
obj.name = "Doe"; // This will not change the object
2. Spread and Rest Operators: These operators can help create shallow copies of objects and arrays, which can then be modified without affecting the original structure.
const original = { name: "John", age: 30 };
const updated = { ...original, age: 31 }; // Create a new object with the updated age
3. Libraries: Libraries like Immutable.js provide a robust set of immutable data structures that are more efficient than manually copying objects or arrays.
const { Map } = require('immutable');
const map1 = Map({ name: "John" });
const map2 = map1.set("name", "Doe");
4. Functional Programming Techniques: Emphasize the use of functions that do not mutate their arguments. Instead, return new values.
const addElement = (arr, element) => [...arr, element];
Challenges of Immutability
While immutability offers many benefits, it also comes with challenges. For example, managing deeply nested objects in an immutable way can be cumbersome without the right tools. Additionally, there’s a learning curve associated with adopting an immutable mindset, especially in a language like JavaScript that is not inherently immutable.
Conclusion
Embracing immutable data structures in JavaScript can lead to more predictable, maintainable, and efficient code. Whether you use built-in methods, spread operators, or libraries like Immutable.js, the benefits of immutability can significantly improve the quality of your applications. As modern JavaScript development continues to evolve, understanding and implementing immutability will become an increasingly valuable skill.
JavaScript Decorators: Adding Functionality to Objects