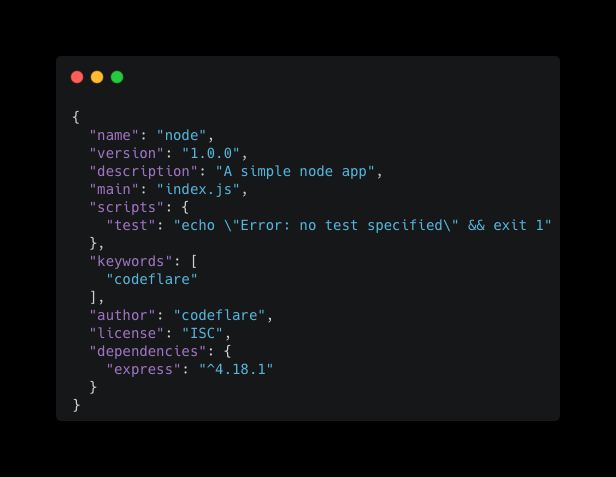
Node.js is useful for building back-end services like APIs, Web Applications or Mobile Applications. It’s used in production by large companies such as Walmart, Uber, Netflix, Paypal, etc.
In this tutorial, we are going to see how we can create and run our first Node JS application.
Free Web And Mobile App Template Source Code
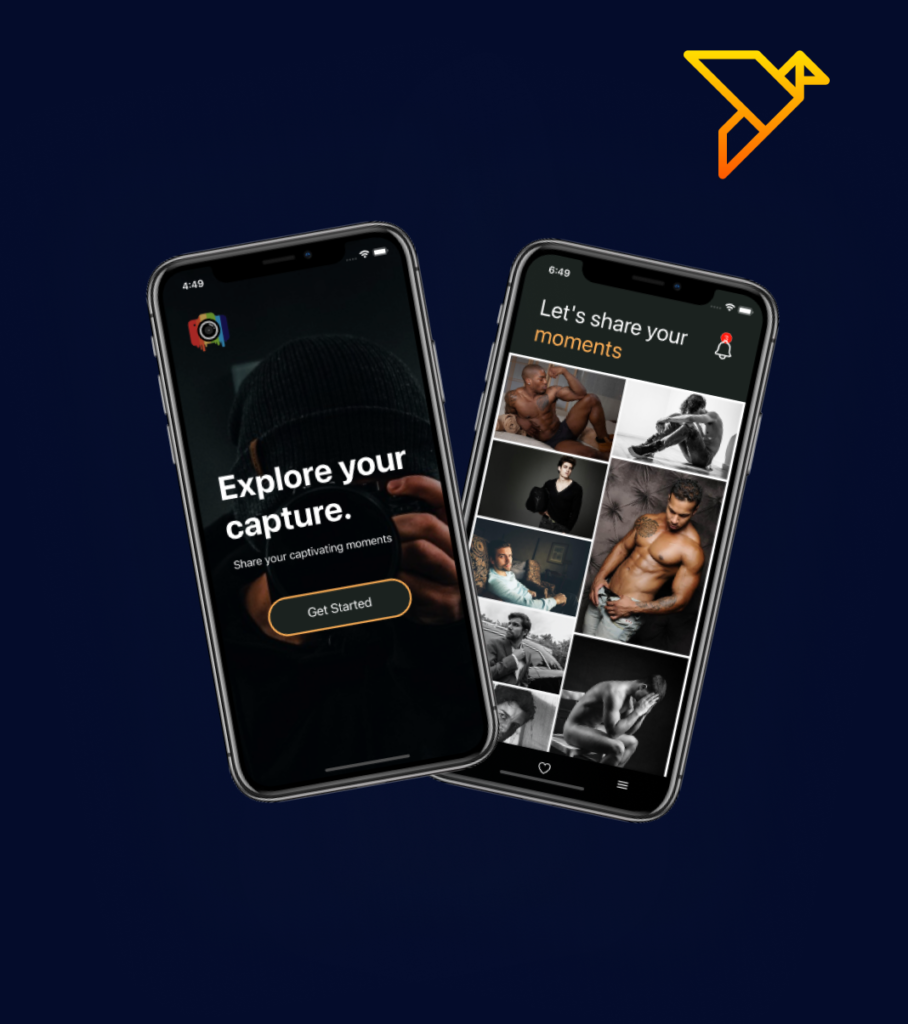
Let’s get started!
1. Download Node JS
First you need to download Node JS. To confirm that it is installed run the command:
node -v
2. Create a folder and open it up in the terminal
Create a project folder and call it whatever you want to call it. Then open up that folder in your terminal / command prompt and run the following command:
npm init
The npm init
command is used to create a Node.js project. The npm init command will create a package where the project files will be stored. All the modules you download will be stored in the package.json file
You will be prompted to add the following project information when creating a project:
- Project name
- Project initial version
- Project description
- The project’s entry point
- The project’s test command
- The project’s git repository
- The project’s license
The default project entry point is usually index.js. Although that can differ from server to server as will look for app.js or main.js to run your application.
3. Add express server
Next, we will add our express server dependency. Express will give you an easier solution to middleware, routing, templating and even debugging.
Run the command:
npm i express
Your package.json file should look like this
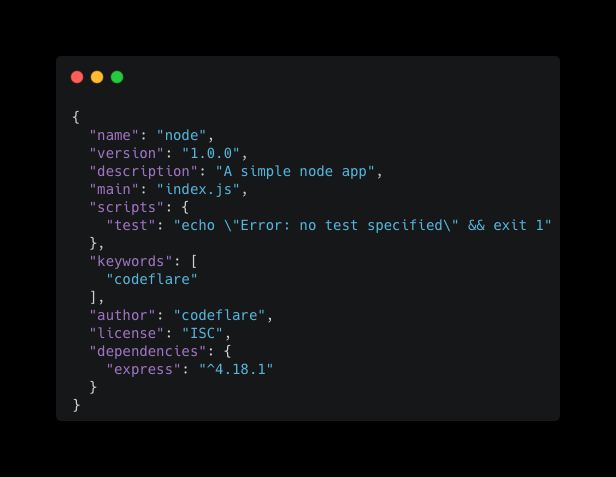
4. Create the index.js file
Now, go to your directory and create a file called index.js, which will be the entry point to our application and add the following code:
const express = require('express');
const app = express();
const port = 5000; //port number where your server will be listening
app.get('/', (req, res) => { //get requests to the root ("/")
res.sendFile('index.html', {root: __dirname});
});
app.listen(port, () => { //server starts listening
console.log(`listening on port ${port}`);
});
5. Create the index.html file
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Node JS Simple App</title>
</head>
<body>
<div>Hello World!</div>
</body>
</html>
6. Finally, Run your application
Run your app with the following command:
node index
Congratulations!
You have created your first node JS application.
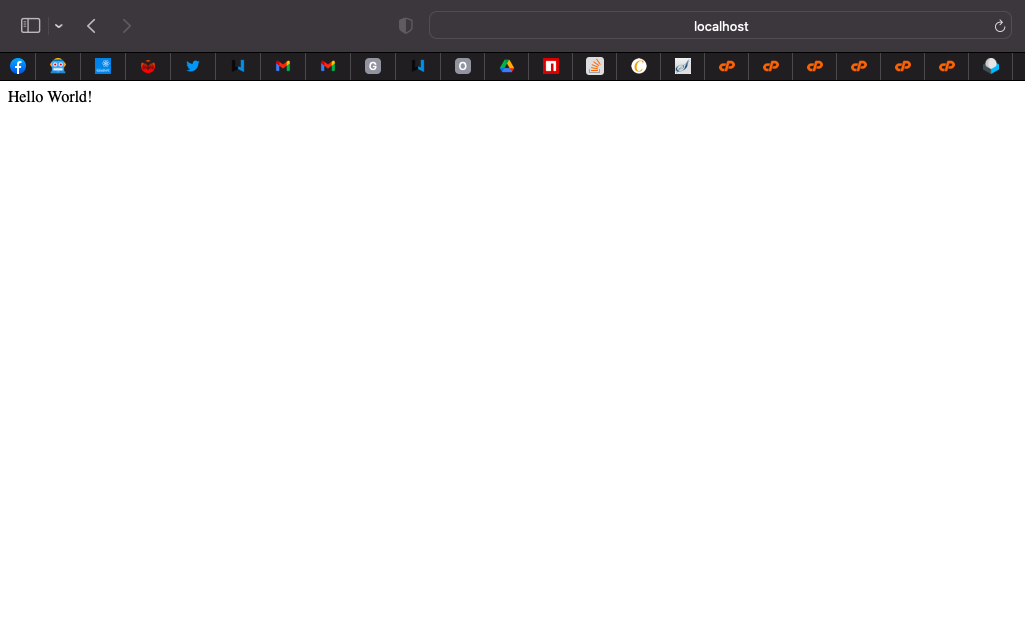
See Also
Create a login form with Node JS and MySQL
Create a registration from with Node JS and MySQL