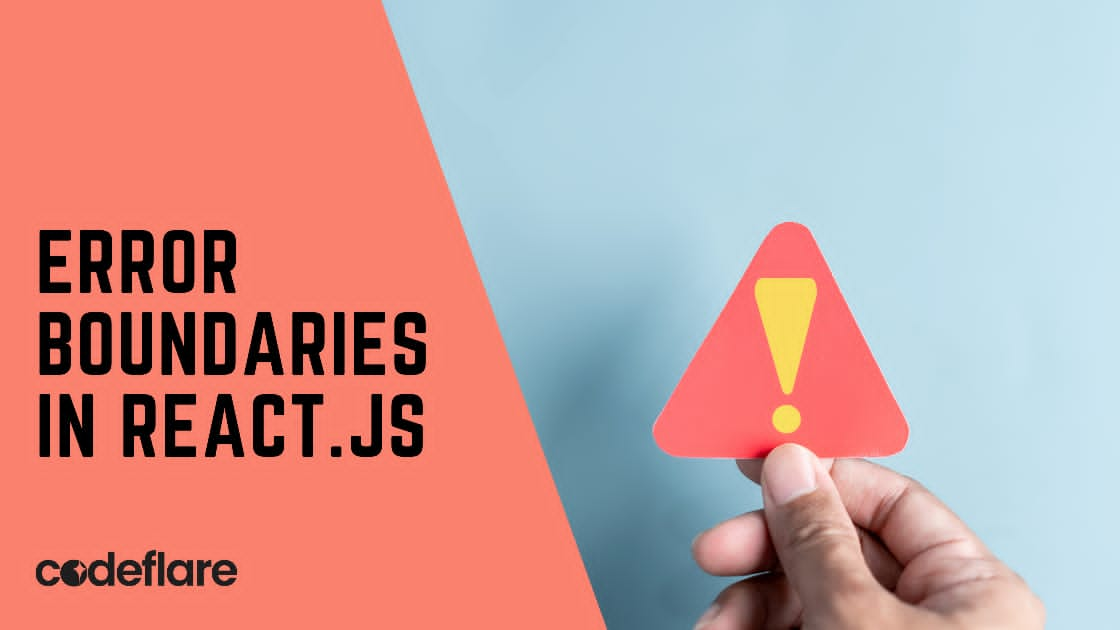
In modern web development, user experience is paramount. A critical aspect of maintaining a smooth user experience is handling errors effectively, especially in large and complex applications. React, a popular JavaScript library for building user interfaces, introduced a powerful feature called Error Boundaries in version 16. Error Boundaries provide a way to catch and handle errors in React components, preventing them from crashing the entire application. This article explores what Error Boundaries in React.js are, how they work, and how to implement them in your React applications.
What are Error Boundaries?
Error Boundaries are React components that catch JavaScript errors anywhere in their child component tree, log those errors, and display a fallback UI instead of crashing the entire component tree. Error Boundaries can catch errors during rendering, in lifecycle methods, and in constructors of the whole tree below them.
However, it’s important to note that Error Boundaries do not catch errors for:
- Event handlers
- Asynchronous code (e.g.,
setTimeout
orrequestAnimationFrame
callbacks) - Server-side rendering
- Errors thrown in the Error Boundary itself (rather than its children)
When to Use Error Boundaries
Error Boundaries are particularly useful in the following scenarios:
- Third-Party Integrations: When integrating third-party components or libraries that might throw errors unexpectedly.
- Critical UI Areas: Wrapping Error Boundaries around critical areas of your application, such as navigation components or parts of the UI that handle important user interactions, ensures that a single error doesn’t crash the entire application.
- Preventing App Crashes: In large applications with complex component hierarchies, a single error can cascade and cause the entire application to crash. Using Error Boundaries helps isolate these errors, allowing the rest of the application to function normally.
How to Implement Error Boundaries
Implementing Error Boundaries in React is straightforward. You create a class component that defines either or both of the following lifecycle methods:
static getDerivedStateFromError(error)
componentDidCatch(error, info)
Here’s an example of a basic Error Boundary component:
import React, { Component } from 'react';
class ErrorBoundary extends Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
// Update state so the next render shows the fallback UI
return { hasError: true };
}
componentDidCatch(error, info) {
// You can also log the error to an error reporting service
console.error("Error caught by Error Boundary:", error, info);
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
export default ErrorBoundary;
In this example:
- The
getDerivedStateFromError
method updates the component state to reflect that an error has occurred. - The
componentDidCatch
method logs the error information. This is where you can also report the error to an external service for further investigation.
Using the Error Boundary
To use the Error Boundary, wrap it around any component you want to protect:
import React from 'react';
import ErrorBoundary from './ErrorBoundary';
import MyComponent from './MyComponent';
function App() {
return (
<ErrorBoundary>
<MyComponent />
</ErrorBoundary>
);
}
export default App;
In this setup, if an error occurs within MyComponent
or its child components, the Error Boundary will catch it and display the fallback UI instead of allowing the error to crash the entire application.
Customizing the Fallback UI
The fallback UI is the interface shown when an error occurs. The example above uses a simple message: “Something went wrong.” However, in real-world applications, you might want to provide a more user-friendly message or even offer recovery options.
Here’s an example of a more customized fallback UI:
render() {
if (this.state.hasError) {
return (
<div className="error-container">
<h1>Oops! Something went wrong.</h1>
<p>We're working on fixing the problem. Please try again later.</p>
</div>
);
}
return this.props.children;
}
Error Boundaries and Hooks
As of now, you can’t use Error Boundaries directly in function components using hooks. Error Boundaries must be class components. However, you can create a custom hook that handles specific errors in functional components or wrap the function components with a class-based Error Boundary.
Best Practices for Using Error Boundaries
- Wrap High-Level Components: Place Error Boundaries high in your component tree to catch errors across multiple components.
- Use Multiple Error Boundaries: Consider using multiple Error Boundaries for different parts of your application. For example, one for your header, one for the main content area, and one for your sidebar.
- Graceful Degradation: Provide meaningful fallback UIs that guide users on what to do next when an error occurs.
- Error Reporting: Integrate with error-reporting tools (e.g., Sentry, LogRocket) to log errors caught by Error Boundaries for better debugging.
Conclusion
“Error Boundaries in React.js are a crucial tool in a developer’s toolkit, allowing you to catch and handle errors gracefully without disrupting the entire user experience. By implementing Error Boundaries in your React applications, you can effectively manage unexpected errors, ensuring a more robust and user-friendly experience.”
Working with PHP’s DateTime Class