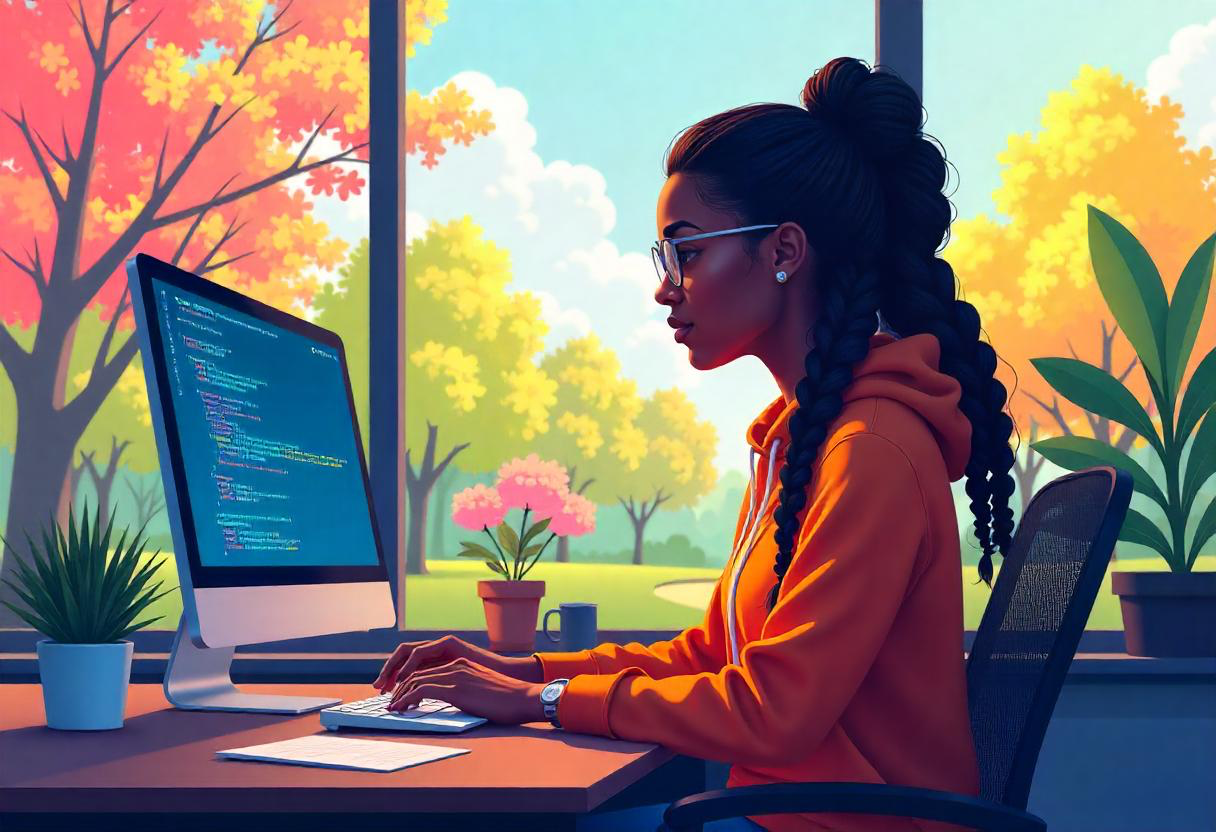
When selecting DOM elements in JavaScript, two common methods are document.querySelector()
and document.getElementById()
. But which one is faster and when should you use each? Let’s break it down. See Memory Management in JavaScript.
1. Speed Comparison 🚀
Benchmark tests consistently show that:
✅ getElementById()
is faster than querySelector()
.
Why?
getElementById()
directly accesses the DOM’s optimized ID lookup system.querySelector()
uses a CSS selector engine, which adds slight overhead.
Performance Test Example
console.time('getElementById');
for (let i = 0; i < 10000; i++) {
document.getElementById('test');
}
console.timeEnd('getElementById'); // ~1-5ms
console.time('querySelector');
for (let i = 0; i < 10000; i++) {
document.querySelector('#test');
}
console.timeEnd('querySelector'); // ~5-15ms
Result: getElementById()
is 2-10x faster in most cases.
2. When to Use Each?
✅ Use getElementById()
When:
- You only need to select one element by ID.
- Performance is critical (e.g., in loops or animations).
✅ Use querySelector()
When:
- You need complex CSS selectors (e.g.,
.class
,[attribute]
,parent > child
). - You want a single method for all selections (IDs, classes, etc.).
3. Key Takeaways
Method | Speed | Use Case |
---|---|---|
getElementById() | ⚡ Fastest | Best for simple ID lookups. |
querySelector() | 🐢 Slower | Best for complex selectors. |
Final Verdict
- If speed matters, use
getElementById()
. - If flexibility matters, use
querySelector()
.
Pro Tip: For modern JS, getElementById()
is still king for pure performance—but querySelector()
is more versatile.
Which do you prefer? 🚀