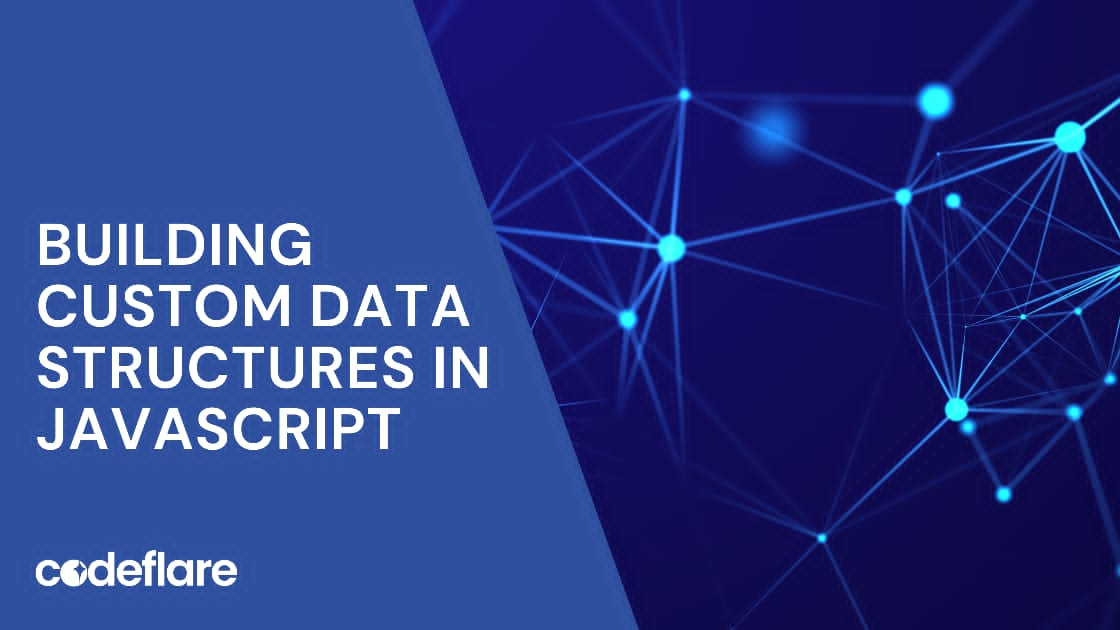
JavaScript, as a versatile programming language, provides several built-in data structures like arrays, objects, maps, and sets. However, there are times when these built-in structures might not meet the specific needs of your application. In such cases, building custom data structures tailored to your unique requirements becomes necessary. This article will guide you through the process of creating custom data structures in JavaScript, covering fundamental concepts, practical examples, and best practices.
Why Build Custom Data Structures?
Custom data structures are essential when the built-in options don’t offer the functionality or efficiency required for specific tasks. For instance, you might need a more specialized structure like a linked list, stack, queue, or binary tree to handle specific algorithms or optimize performance for certain operations. By creating your own data structures, you gain more control over how data is stored, accessed, and manipulated, leading to more efficient and maintainable code.
Key Concepts to Understand
Before diving into the implementation, it’s important to grasp some key concepts:
- Abstraction: This involves hiding the complex implementation details and exposing only the necessary parts of the data structure. Abstraction helps in making your data structures easier to use and understand.
- Encapsulation: This refers to bundling the data (variables) and methods (functions) that operate on the data into a single unit or class. Encapsulation protects the data from unauthorized access and modification.
- Efficiency: Efficiency in custom data structures is measured in terms of time complexity (how fast operations like insertions, deletions, and searches can be performed) and space complexity (how much memory is consumed).
Creating a Custom Stack
A stack is a basic data structure that follows the Last In, First Out (LIFO) principle. Here’s a simple implementation of a stack in JavaScript:
class Stack {
constructor() {
this.items = [];
}
// Add an element to the stack
push(element) {
this.items.push(element);
}
// Remove the top element from the stack
pop() {
if (this.isEmpty()) {
return "Stack is empty";
}
return this.items.pop();
}
// View the top element in the stack
peek() {
if (this.isEmpty()) {
return "Stack is empty";
}
return this.items[this.items.length - 1];
}
// Check if the stack is empty
isEmpty() {
return this.items.length === 0;
}
// Get the size of the stack
size() {
return this.items.length;
}
// Clear the stack
clear() {
this.items = [];
}
}
// Usage example
let stack = new Stack();
stack.push(10);
stack.push(20);
stack.push(30);
console.log(stack.pop()); // Output: 30
console.log(stack.peek()); // Output: 20
console.log(stack.size()); // Output: 2
Creating a Custom Queue
A queue is another fundamental data structure that follows the First In, First Out (FIFO) principle. Below is an implementation of a queue in JavaScript:
class Queue {
constructor() {
this.items = [];
}
// Add an element to the queue
enqueue(element) {
this.items.push(element);
}
// Remove the front element from the queue
dequeue() {
if (this.isEmpty()) {
return "Queue is empty";
}
return this.items.shift();
}
// View the front element in the queue
front() {
if (this.isEmpty()) {
return "Queue is empty";
}
return this.items[0];
}
// Check if the queue is empty
isEmpty() {
return this.items.length === 0;
}
// Get the size of the queue
size() {
return this.items.length;
}
// Clear the queue
clear() {
this.items = [];
}
}
// Usage example
let queue = new Queue();
queue.enqueue(10);
queue.enqueue(20);
queue.enqueue(30);
console.log(queue.dequeue()); // Output: 10
console.log(queue.front()); // Output: 20
console.log(queue.size()); // Output: 2
Creating a Custom Linked List
A linked list is a data structure consisting of nodes, where each node contains data and a reference (or link) to the next node in the sequence. Below is a simple implementation of a singly linked list in JavaScript:
class Node {
constructor(element) {
this.element = element;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.size = 0;
}
// Add an element to the linked list
add(element) {
let node = new Node(element);
let current;
if (this.head === null) {
this.head = node;
} else {
current = this.head;
while (current.next) {
current = current.next;
}
current.next = node;
}
this.size++;
}
// Remove an element from the linked list
remove(element) {
let current = this.head;
let prev = null;
while (current !== null) {
if (current.element === element) {
if (prev === null) {
this.head = current.next;
} else {
prev.next = current.next;
}
this.size--;
return current.element;
}
prev = current;
current = current.next;
}
return -1;
}
// Check if the linked list is empty
isEmpty() {
return this.size === 0;
}
// Get the size of the linked list
size_of_list() {
return this.size;
}
// Print the linked list
printList() {
let current = this.head;
let str = "";
while (current) {
str += current.element + " ";
current = current.next;
}
console.log(str);
}
}
// Usage example
let ll = new LinkedList();
ll.add(10);
ll.add(20);
ll.add(30);
ll.printList(); // Output: 10 20 30
ll.remove(20);
ll.printList(); // Output: 10 30
Best Practices for Building Custom Data Structures
- Understand the Problem: Before implementing a custom data structure, clearly understand the problem you’re trying to solve and determine if a custom solution is necessary.
- Optimize for Performance: Consider the time and space complexity of your data structure and strive for efficient operations.
- Test Thoroughly: Rigorously test your custom data structures to ensure they function correctly in all scenarios.
- Document Your Code: Provide clear documentation for your custom data structures to make them easier to understand and maintain.
Conclusion
Building custom data structures in JavaScript allows you to create tailored solutions for specific problems that built-in structures might not adequately address. Whether it’s a stack, queue, linked list, or more complex structures like trees or graphs, understanding how to implement these from scratch gives you greater control and flexibility in your programming projects. By mastering these techniques, you can optimize your code for performance and maintainability, making your applications more robust and efficient.
Advanced features and techniques on Laravel PHP Framework