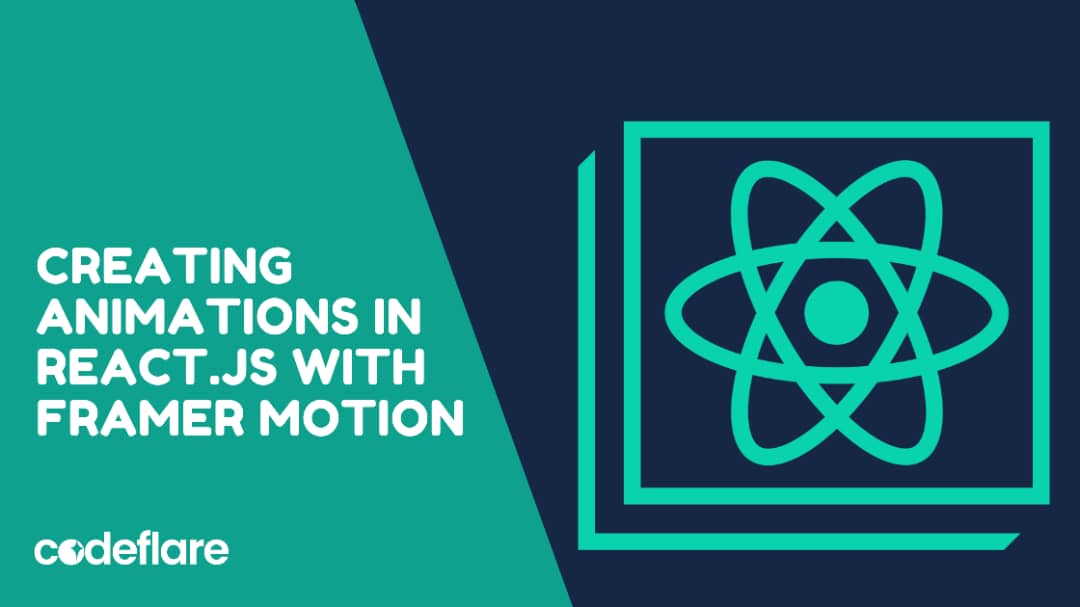
Introduction
Animations can significantly enhance the user experience of a web application, making it both more engaging and interactive. In particular, in React.js, Framer Motion offers a powerful and easy-to-use library for creating complex animations with minimal code. As a result, this article will guide you through the process of using Framer Motion to craft stunning animations in your React.js projects. Furthermore, we will explore both basic and advanced techniques, providing you with the knowledge needed to elevate your application’s visual appeal.
What is Framer Motion?
Framer Motion stands out as a popular animation library for React, offering a straightforward API for crafting intricate animations and transitions. It simplifies the process of animating components by providing features such as spring animations, layout transitions, and keyframe animations. Consequently, developers can effortlessly enhance their React applications with dynamic, smooth animations. By using Framer Motion, you avoid the complexities of traditional CSS or JavaScript animation techniques, making the implementation of engaging animations more accessible and efficient.
Getting Started with Framer Motion
1. Installation
To begin using Framer Motion, you need to install it via npm or yarn. Open your terminal and run:
npm install framer-motion
Or
yarn add framer-motion
2. Basic Usage
After installation, you can start using Framer Motion in your React components. Import motion
from the library and wrap your components with it. Here’s a simple example of animating a component:
import { motion } from 'framer-motion';
function AnimatedComponent() {
return (
<motion.div
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
transition={{ duration: 1 }}
>
<h1>Welcome to Framer Motion!</h1>
</motion.div>
);
}
export default AnimatedComponent;
In this example, the motion.div
component starts with zero opacity and animates to full opacity over one second.
Creating Complex Animations
Framer Motion allows for more sophisticated animations using keyframes, variants, and dynamic transitions. Here are a few advanced techniques:
1. Variants
In addition, variants let you define multiple animation states for a component, making it easier to handle complex animations.
import { motion } from 'framer-motion';
const variants = {
hidden: { opacity: 0 },
visible: { opacity: 1 },
};
function AnimatedComponent() {
return (
<motion.div
initial="hidden"
animate="visible"
variants={variants}
transition={{ duration: 1 }}
>
<h1>Complex Animations with Variants</h1>
</motion.div>
);
}
export default AnimatedComponent;
Here, the motion.div
transitions between the hidden
and visible
states defined in the variants
object.
2. Spring Animations
Moreover, Framer Motion’s spring animations create natural, physics-based animations:
import { motion } from 'framer-motion';
function SpringAnimation() {
return (
<motion.div
initial={{ x: -100 }}
animate={{ x: 0 }}
transition={{ type: 'spring', stiffness: 100 }}
>
<h1>Spring Animation</h1>
</motion.div>
);
}
export default SpringAnimation;
In this example, the component moves from left to right with a spring-like effect.
3. Layout Transitions
Framer Motion also supports animating layout changes seamlessly:
import { motion } from 'framer-motion';
import { useState } from 'react';
function LayoutAnimation() {
const [isExpanded, setIsExpanded] = useState(false);
return (
<motion.div
layout
initial={{ borderRadius: 10 }}
animate={{ borderRadius: isExpanded ? 50 : 10 }}
onClick={() => setIsExpanded(!isExpanded)}
style={{ width: 200, height: 200, backgroundColor: '#f00' }}
>
<h1>Click to Animate</h1>
</motion.div>
);
}
export default LayoutAnimation;
- Here, clicking the component triggers a layout transition that animates the border radius.
Best Practices for Using Framer Motion
- Performance Considerations: While Framer Motion is efficient, keep an eye on performance for complex animations, especially in large applications.
- Consistent Styling: Therefore, use consistent styling and animation timings to ensure a cohesive user experience.
- Testing: Consequently, test animations across different devices and browsers to ensure smooth performance and visual consistency.
Conclusion
Framer Motion greatly empowers React developers to create impressive animations with minimal effort. By leveraging its features, you can significantly enhance your web applications with engaging and dynamic animations that ultimately improve the user experience. Whether you’re animating simple components or designing more complex interactions, Framer Motion provides you with the tools you need to effectively bring your React applications to life. Additionally, the library simplifies the process, allowing you to achieve sophisticated effects with ease.
How to implement Authentication in React.js