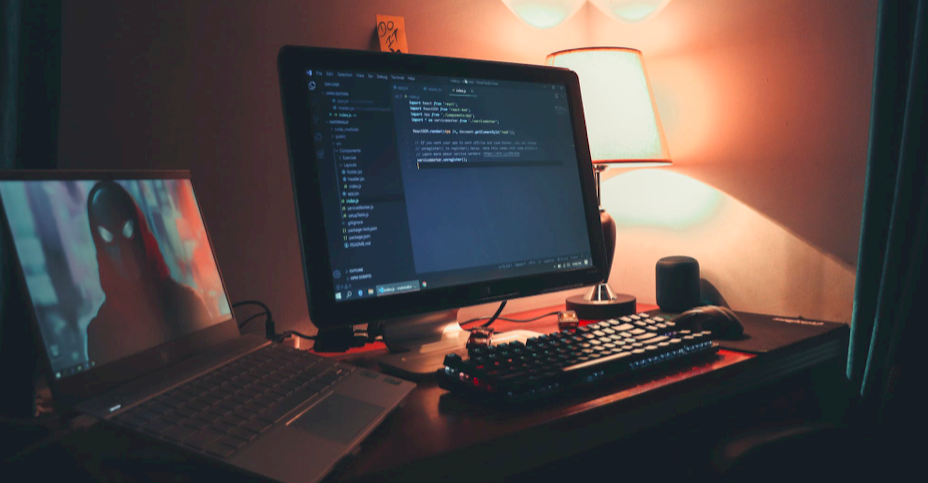
In JavaScript, null
, undefined
, and not defined
are three distinct concepts that refer to different values or states. In this article we shall look at null, undefined and not defined in Javascript and their unique use cases
Null
Null is the intentional absence of any object value. This could be a property of an object, or an expected value of a variable.
Example
let person = {
"name": "Lawson Luke",
"hobby": null
}
console.log(person.hobby); //null
When validating user inputs, values can also be checked for null values
let email = null;
if(email === null){
alert('Please enter your email');
}
Undefined
Undefined, in the simplest term, means that a property of variable is not initialized or does not exist.
let person = {
"name": "Lawson Luke",
"hobby": "coding",
}
console.log(person.age); //undefined
Not defined
“Not defined” refers to a variable that is not declared at any given point in time with the declaration keyword like var, let or const.
console.log(name); //Uncaught ReferenceError: name is not defined
It is important to note that null
and undefined
are distinct values, but they are both falsy values in JavaScript, which means they will evaluate to false
in a Boolean context. Also, not defined
is an error that occurs when you try to reference a variable or function that has not been declared
Conclusion
Now we know the difference between null, undefined and “not defined” in Javascript, and we can begin to write cleaner code and better logic.