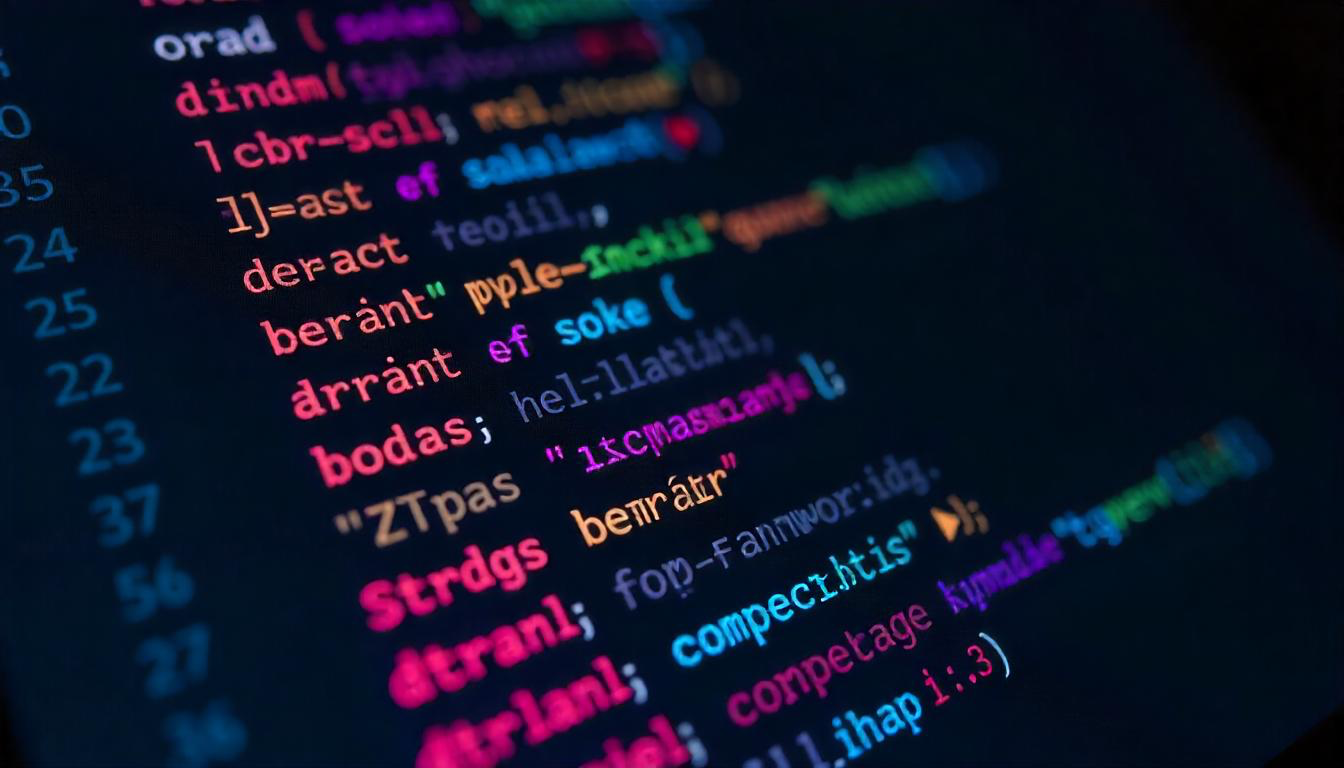
JavaScript can sometimes behave in unexpected ways, especially when comparing arrays and objects. If you’ve ever wondered why [] === []
evaluates to false
, this guide explains the reason and provides practical solutions for comparing arrays and objects correctly. See Optional Chaining (?.): How to Avoid ‘Cannot Read Property’ Errors in JavaScript
1. Why [] === []
is false
in JavaScript
The Root Cause: Reference vs. Value Comparison
In JavaScript, arrays and objects are reference types, meaning:
- When you create an array (
[]
) or object ({}
), JavaScript stores it in memory and returns a reference (memory address) to it. - The
===
(strict equality) operator checks if two variables point to the same memory location, not if their contents are identical.
const arr1 = [];
const arr2 = [];
console.log(arr1 === arr2); // false (different memory references)
console.log(arr1 === arr1); // true (same reference)
How Primitive Types Differ
Unlike arrays/objects, primitives (numbers, strings, booleans, etc.) are compared by value:
console.log(5 === 5); // true
console.log("hello" === "hello"); // true
2. How to Compare Arrays & Objects Properly
Since ===
doesn’t work for deep comparisons, here are alternative methods:
A. Comparing Arrays
Method 1: JSON.stringify()
(Simple, but Limited)
Converts arrays to strings for comparison:
const arr1 = [1, 2, 3];
const arr2 = [1, 2, 3];
console.log(JSON.stringify(arr1) === JSON.stringify(arr2)); // true
⚠ Limitation: Fails if elements are in different orders or contain undefined
/functions.
Method 2: Manual Loop Check (More Reliable)
Compare each element individually:
function areArraysEqual(arr1, arr2) {
if (arr1.length !== arr2.length) return false;
return arr1.every((item, index) => item === arr2[index]);
}
console.log(areArraysEqual([1, 2], [1, 2])); // true
console.log(areArraysEqual([1, 2], [2, 1])); // false (order matters)
Method 3: Using lodash.isEqual
(Best for Complex Arrays)
The Lodash library provides deep comparison:
import _ from 'lodash';
console.log(_.isEqual([1, 2], [1, 2])); // true
B. Comparing Objects
Method 1: JSON.stringify()
(Works for Simple Objects)
const obj1 = { name: "Alice" };
const obj2 = { name: "Alice" };
console.log(JSON.stringify(obj1) === JSON.stringify(obj2)); // true
⚠ Limitation: Fails if properties are in different orders.
Method 2: Manual Key-Value Check
function areObjectsEqual(obj1, obj2) {
const keys1 = Object.keys(obj1);
const keys2 = Object.keys(obj2);
if (keys1.length !== keys2.length) return false;
return keys1.every(key => obj1[key] === obj2[key]);
}
console.log(areObjectsEqual({ a: 1 }, { a: 1 })); // true
Method 3: Using lodash.isEqual
(Best for Nested Objects)
console.log(_.isEqual({ a: 1 }, { a: 1 })); // true
3. Key Takeaways
✔ [] === []
is false
because arrays/objects are compared by reference, not content.
✔ Use JSON.stringify()
for simple comparisons (but be cautious with order/undefined
).
✔ For deep comparisons, use lodash.isEqual
or write a custom function.
✔ Primitives (5
, "hello"
) compare by value, so ===
works as expected.
Final Tip: When to Use Each Method
Case | Best Approach |
---|---|
Simple arrays/objects | JSON.stringify() |
Order-sensitive arrays | Manual loop check |
Complex/nested objects | lodash.isEqual() |
Performance-critical | Custom comparison function |
Now you know why [] === []
is false
—and how to compare arrays & objects properly! 🚀