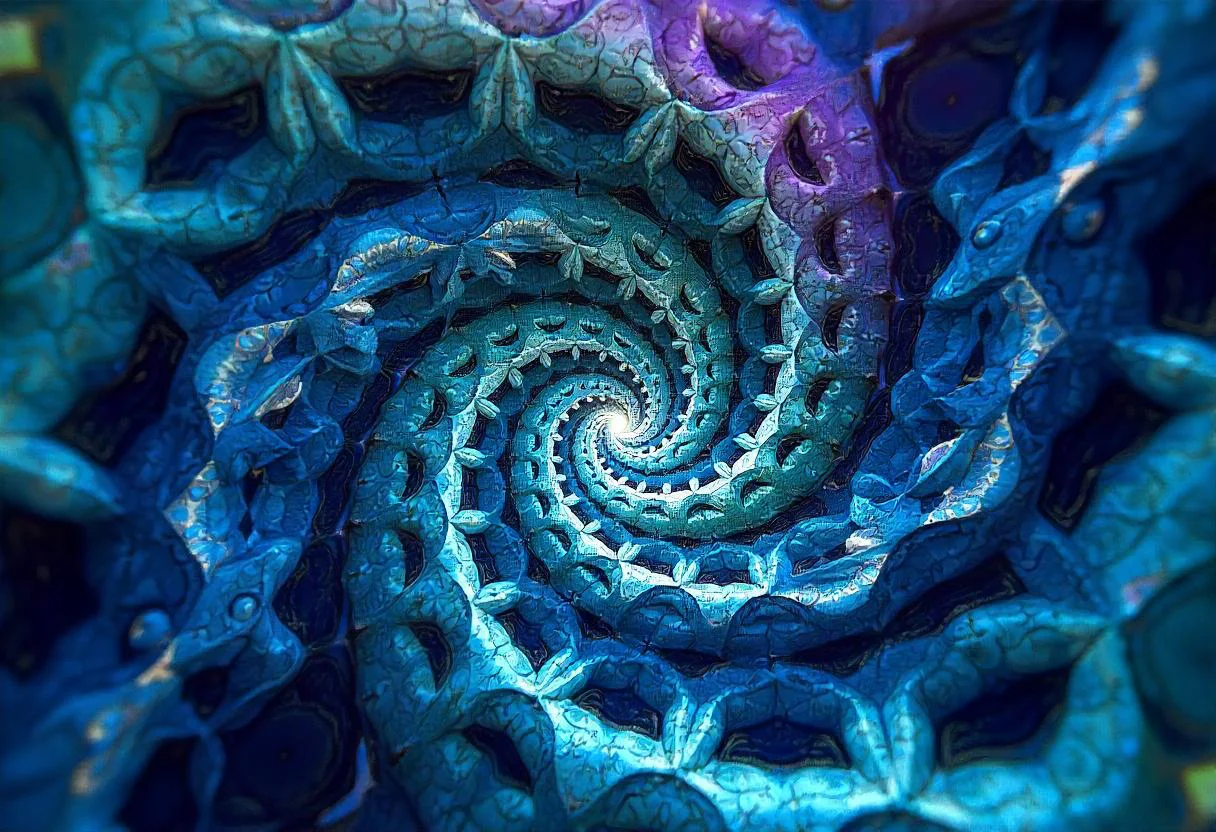
Recursion is a programming technique where a function calls itself to solve smaller instances of the same problem. It’s elegant but tricky for beginners—let’s break it down with clear examples in Python, JavaScript, and Java.
What is Recursion?
You’re Not a Senior Developer Until You Have These 8 Traits
Recursion solves problems by:
- Base Case – The simplest scenario (stops recursion).
- Recursive Case – Breaking the problem into smaller subproblems.
Example: Factorial Calculation
See document.querySelector() vs. getElementById(): Which is Faster?
1. Python
def factorial(n):
if n == 1: # Base case
return 1
return n * factorial(n - 1) # Recursive case
print(factorial(5)) # Output: 120
2. JavaScript
function factorial(n) {
if (n === 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
console.log(factorial(5)); // Output: 120
3. Java
public class Main {
static int factorial(int n) {
if (n == 1) return 1; // Base case
return n * factorial(n - 1); // Recursive case
}
public static void main(String[] args) {
System.out.println(factorial(5)); // Output: 120
}
}
Another Example: Fibonacci Sequence
Python
def fibonacci(n):
if n <= 1: return n # Base case
return fibonacci(n - 1) + fibonacci(n - 2)
print(fibonacci(6)) # Output: 8
JavaScript
javascript
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
console.log(fibonacci(6)); // Output: 8
3. Java
public class Main {
static int fibonacci(int n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
public static void main(String[] args) {
System.out.println(fibonacci(6)); // Output: 8
}
}
When to Use Recursion?
✔ Tree/Graph Traversal (e.g., binary search trees).
✔ Divide & Conquer Algorithms (e.g., mergesort).
✔ Problems with repetitive subproblems (but watch for inefficiency!).
Watch Out For:
❌ Missing base case → Stack Overflow!
❌ Exponential time complexity (e.g., naive Fibonacci).
Final Challenge
Try implementing a recursive sum of numbers from 1
to n
in all three languages!