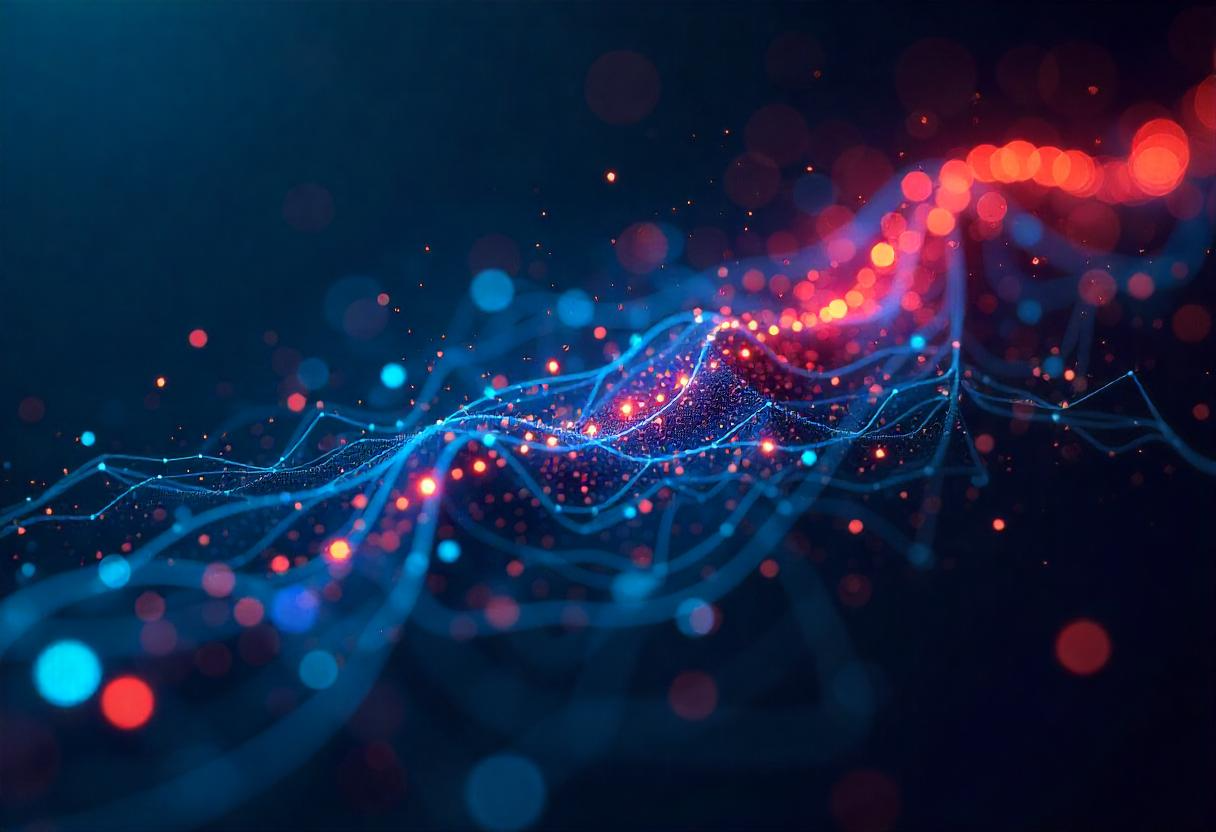
Handling large amounts of data efficiently can be a challenge for developers, especially when working with files, APIs, or network requests. In Node.js, streams provide a powerful way to handle such data, enabling it to be processed incrementally without exhausting system memory. This article explores what streams are, how they work, and why they are essential for dealing with large datasets in Node.js. Attend a professional software development training in Abuja
What Are Streams in Node.js?
In simple terms, a stream is a continuous flow of data. Streams allow you to read or write data piece by piece, rather than loading the entire dataset into memory at once. Node.js streams follow the pattern of working with chunks, making them ideal for handling large files, media processing, or streaming data over the network.
Types of Streams in Node.js
Node.js offers four main types of streams:
- Readable Streams
- Used to read data from a source (e.g., file input).
- Example:
fs.createReadStream()
to read a file in chunks.
- Writable Streams
- Used to write data to a destination (e.g., file output).
- Example:
fs.createWriteStream()
to write data to a file.
- Duplex Streams
- These streams are both readable and writable (e.g., TCP socket).
- Transform Streams
- Used to modify or transform data as it passes through (e.g., zipping or encrypting files).
How Streams Work: A Simple Example
Below is an example of how to use a readable stream to read a file in chunks and log the data:
const fs = require('fs');
// Create a readable stream
const readStream = fs.createReadStream('largeFile.txt', 'utf8');
// Handle the 'data' event to read chunks
readStream.on('data', (chunk) => {
console.log('Received chunk:', chunk);
});
// Handle the 'end' event
readStream.on('end', () => {
console.log('Finished reading file');
});
In this example:
- The file is read in small chunks rather than loading it all at once.
- This approach prevents memory overload and ensures smooth data handling.
Advantages of Using Streams
- Memory Efficiency
- Streams handle data incrementally, preventing large datasets from consuming all available memory.
- Faster Performance
- Streams allow data processing to begin as soon as the first chunk is available, leading to faster operations.
- Scalability
- Suitable for applications dealing with massive files, such as media servers or logging systems.
- Pipelining and Chaining
- Streams can be chained using the
pipe()
method, making it easy to pass data between different operations.
- Streams can be chained using the
Using the pipe()
Method
The pipe()
method allows you to connect streams and pass data efficiently between them. Here’s an example of copying a large file using streams:
const fs = require('fs');
// Create readable and writable streams
const readStream = fs.createReadStream('source.txt');
const writeStream = fs.createWriteStream('destination.txt');
// Pipe data from readStream to writeStream
readStream.pipe(writeStream);
console.log('File copied successfully!');
Error Handling in Streams
Handling errors is crucial when working with streams, as they involve real-time data processing. Here’s how to manage errors:
readStream.on('error', (err) => {
console.error('Error reading file:', err);
});
writeStream.on('error', (err) => {
console.error('Error writing file:', err);
});
Use Cases of Node.js Streams
- Reading and writing large files (e.g., media files or log files).
- Streaming video or audio over the internet.
- Handling HTTP requests and responses in web servers.
- Real-time data processing (e.g., live chat applications).
- Compressing or encrypting files using transform streams.
Conclusion
Node.js streams are a powerful tool for efficiently handling large datasets, offering memory efficiency, scalability, and real-time data processing. Whether you’re working on file operations, network requests, or media streaming, understanding how to use streams effectively can significantly improve your Node.js applications. With the ability to pipe and chain streams, developers can create efficient workflows and avoid common pitfalls like memory overflow.